How to Create a Search Filter in Angular
- the Angular Search Filter
- Steps to Create a Search Filter in Angular
- Advantages of Custom Search Filter
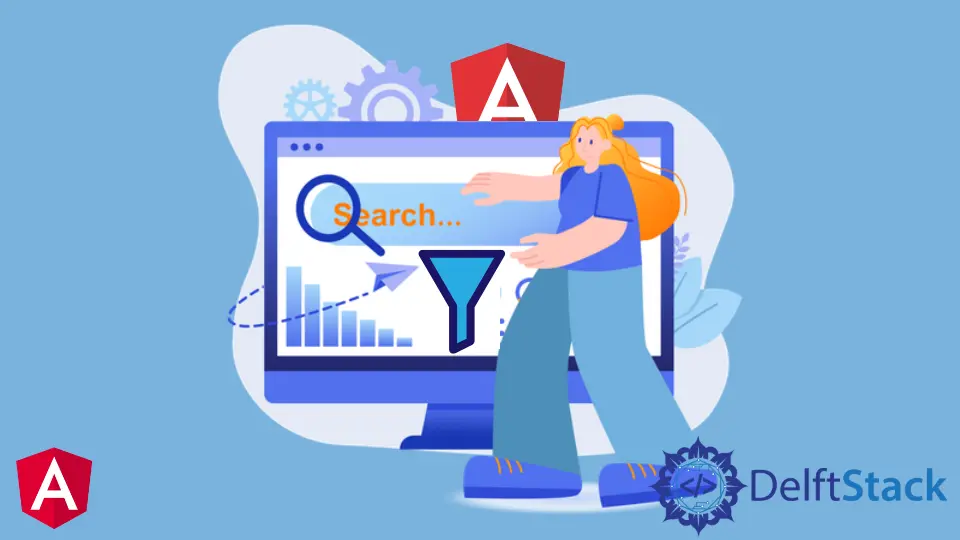
This article will demonstrate how to use the ng2-search-filter
package and ngfor
loop directive to create a search filter in an Angular project to filter the collected data.
the Angular Search Filter
The Angular framework includes many features that can create various applications. One such feature is the ability to use pipes
, which are filters that can be applied to data before it is displayed on the screen.
Angular search filter provides a declarative way to filter data as it enters or leaves a controller’s scope. The filter can be applied to any data object: strings, arrays, objects, or even primitives.
Filters are executed from left to right until one returns true, or all have been executed without returning true. The best approach to construct a custom search in Angular is to use the ng2-search-filter
package rather than using any third-party library.
Steps to Create a Search Filter in Angular
This section will demonstrate the steps required for creating a search filter in Angular.
Install the Angular CLI
First, install the Angular CLI tool, which is used to develop Angular applications. Run this command to install Angular CLI.
npm install @angular/cli
Install the ng2-search-filter
Package
The second and the most important step is to install the ng2-search-filter
package.
npm i ng2-search-filter
The ng2-search-filter
provides a search input component for Angular apps. It allows the user to filter a list of items by typing into the input box.
The component is highly configurable and supports many different data sources.
Import the Modules
The third step is to import the FormsModule
and Ng2SearchPipeModule
in the App Module class. Because if you want to create a search form for your website, you would need to import both modules.
Write the following code in your Angular app.
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { Ng2SearchPipeModule } from 'ng2-search-filter';
import { AppComponent } from './app.component';
@NgModule({
imports: [ BrowserModule, FormsModule, Ng2SearchPipeModule ],
declarations: [ AppComponent ],
bootstrap: [ AppComponent ]
})
export class AppModule { }
Write the TypeScript and HTML Code
This step will decide how your search filter will look. We will write TypeScript and HTML code.
TypeScript code:
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css']
})
export class AppComponent {
searchText: any;
names = [
{ country: 'Adil'},
{ country: 'John'},
{ country: 'Jinku'},
{ country: 'Steve'},
{ country: 'Sam'},
{ country: 'Zeed'},
{ country: 'Abraham'},
{ country: 'Heldon'}
];
}
HTML code:
<h1>Example of Angular Search Filter</h1>
<div class="container">
<div class="search-name">
<input class="form-control" type="text" name="search" [(ngModel)]="searchText" autocomplete="on" placeholder=" SEARCH ">
</div>
<ul *ngFor="let name of names | filter:searchText">
<li>
<span>{{name.country}}</span>
</li>
</ul>
</div>
In this example, we wrote some random names, and with the help of the ng2-search-filter
and ng-for
loop, we were able to filter the data by searching.
Advantages of Custom Search Filter
We have learned all the steps to create a search filter in Angular. Let’s look at the benefits of creating a custom search filter with the help of the ng2-search-filter
package.
- It is easy to use and customize according to the application’s needs.
- It is an Angular core component that provides flexibility and accuracy.
- The search filter can be used with any data, not just strings.
Click here to check the live demonstration of all the steps above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook