How to Use AngularJS $resource
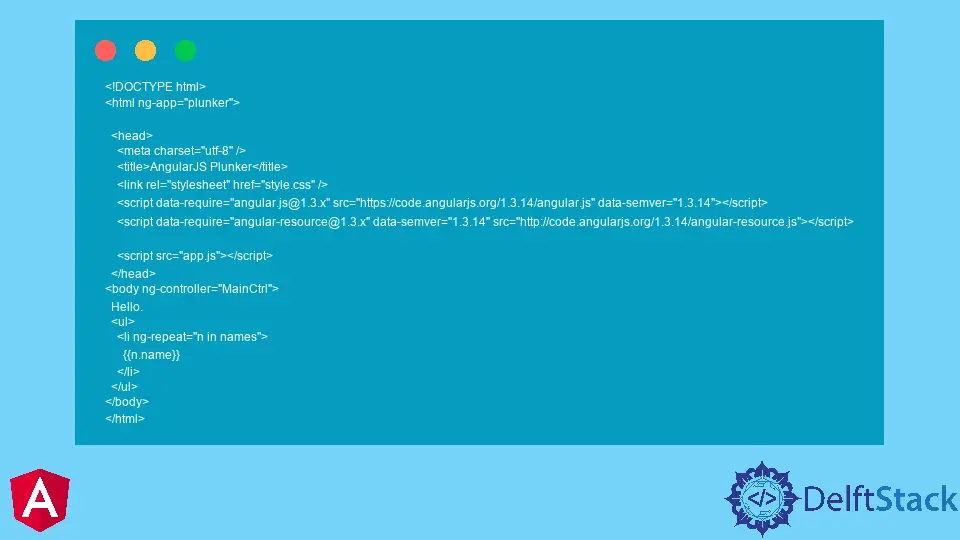
AngularJS $resource
is a service that lets you access RESTful
web services in a way that is more Angular-friendly. $resource
takes care of serializing and deserializing data for you to focus on the application logic.
Moreover, AngularJS $resource
provides functions to communicate with RESTful
APIs. These functions simplify retrieving data from various sources, supporting all HTTP methods.
By default, $resource
performs the following five REST
operations.
- Getting a list of items
- Getting an item
- Inserting an item
- Updating an item
- Deleting an item
AngularJS $resource
vs. $http
The $resource
service is a way to query remote resources, like REST endpoints. It is an AngularJS service that provides functions for creating objects representing remote data and performing CRUD
operations on those objects.
$http
is an AngularJS service that provides functions for making AJAX
requests to the server. It can be used with any AJAX
library, but it must be used with AngularJS’s $resource
service to get the full CRUD
functionality.
$resource
is a variable that is declared once and then used to call get, post, query, remove, and delete across our controller/service. The $resource
contains all the handy methods built-in, which is a great feature.
We don’t need to write repeating code when accessing REST
API with $resource
, which allows us to make our calls more superficial and more relevant.
Example of AngularJS $resource
JavaScript Code:
var app = angular.module('plunker', ['ngResource']);
app.factory('demo', function($resource) {
var url = 'sample.json';
var res = $resource(url, null, {
query: {
isArray: true,
transformResponse: function(data) {
var items = angular.fromJson(data);
var models = [];
class Person {
constructor(config) {
this.name=config.name;
}
sayHello() {
} }
angular.forEach(items, function(item) {
models.push(new Person(item));
});
console.log("models: ", models);
return models;
}
}
});
return res;
});
app.controller('MainCtrl', function($scope, demo) {
demo.query()
.$promise.then(function (data) {
$scope.names = data;
});
});
HTML Code:
<!DOCTYPE html>
<html ng-app="plunker">
<head>
<meta charset="utf-8" />
<title>AngularJS Plunker</title>
<link rel="stylesheet" href="style.css" />
<script data-require="angular.js@1.3.x" src="https://code.angularjs.org/1.3.14/angular.js" data-semver="1.3.14"></script>
<script data-require="angular-resource@1.3.x" data-semver="1.3.14" src="http://code.angularjs.org/1.3.14/angular-resource.js"></script>
<script src="app.js"></script>
</head>
<body ng-controller="MainCtrl">
Hello.
<ul>
<li ng-repeat="n in names">
{{n.name}}
</li>
</ul>
</body>
</html>
It’s a powerful and helpful tool for developers, simplifying the process of querying data from the server.
It is most commonly used for CRUD
operations on RESTful
APIs, but it can also be used to retrieve data from any API.
The main benefit of AngularJS $resource
is that it saves time and makes coding easier by reducing the need to write boilerplate code.
Click here to check the live demonstration of the code as mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook