Angular Page Refresh
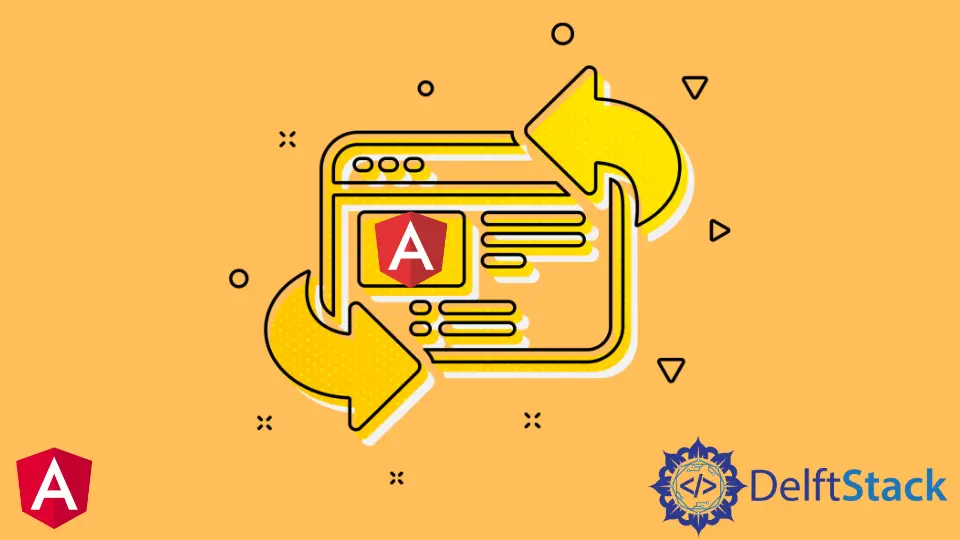
Being a single-page application, Angular requires that the app is refreshed by default when new data is passed. We will look at the best method to do a page refresh on Angular.
Install and Import Some Dependencies
First, we will have to head to our editor, open the terminal most preferably Visual Studio Code and create the app-routing.module.ts
using the ng generate
function.
Then, we run the $ ng generate component home
command, after which we run the $ ng generate component about
command.
After the app-routing.module.ts
file has been created, we open its file and import the following components.
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { HomeComponent } from './home/home.component';
import { AboutComponent } from './about/about.component';
Next, add the routes as follows:
const routes: Routes = [
{ path: 'home', component: HomeComponent },
{ path: 'about', component: AboutComponent }
];
This creates two pages with separate URLs, such that if you head to the /home
path, it takes you to the home component; the about component does likewise.
While still in the app-routing.ts
page, we code in these commands:
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
Create a Button to Refresh Page in Angular
Then we head over to the app.component.html
to create a button:
<div style="text-align:center">
<h1>
Welcome to {{ title }}!
</h1>
<a routerLink="/test" >Test</a>
<button type="button" (click)="refresh()" >
Refresh
</button>
</div>
<router-outlet></router-outlet>
Our final stop will be in app.component.ts
section where we pass the next snippet of codes. But first, we have to import some functions:
import { Component } from '@angular/core';
import { Router } from '@angular/router';
import { Location } from '@angular/common';
Then we run these codes in the app.component.ts
:
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'refreshPage';
constructor(public _router: Router, public _location: Location) { }
refresh(): void {
this._router.navigateByUrl("/refresh", { skipLocationChange: true }).then(() => {
console.log(decodeURI(this._location.path()));
this._router.navigate([decodeURI(this._location.path())]);
});
}
}
The real magic happens with the skipLocationChange
function. When the refresh button is clicked, data is passed on the page without refreshing the whole page, such that it is not even recorded in the browser’s history.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn