How to Use @Input() in Angular
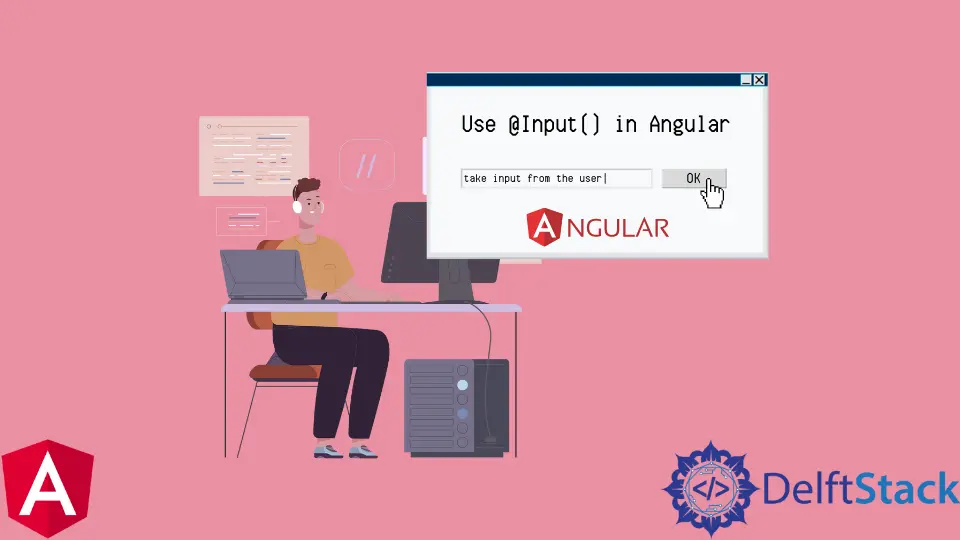
This article is all about Angular @Input()
, a function used in Angular to take input from the user and then use it for processing in the program. It is also possible to use this function for reading a file and getting data from an external API.
the @Input()
Directive in Angular
The @Input()
directive, as the name suggests, is used for input fields. @Input()
is best for capturing user input in form fields and text boxes.
When the user enters information, it automatically updates the value of the field or text box. It also accepts values from other inputs and ngModelOptions
to automatically update its value.
The @Input()
directive has three attributes: type
, name
, and value
.
- The
type
attribute specifies the input field type to be created. - The
name
attribute specifies the id of the input field and will be used as its name in JavaScript code. - The
value
attribute is required if you create a text input field with no default value set.
Below is a list of valid types for an input field:
Text
Password
Email Address
Number
Date Picker
(Date
)Time Picker
(Time
)Range Slider
(Range
)Checkbox
(Checkbox
)
To use @Input()
, you need to add it as a directive on the desired HTML element.
@Input()
is used to transmit data from the parent component to the child component, whereas @Output()
is used to pass data from the child component to the parent component. A typical example of @Input()
is to ask for the user’s age.
The following code will display a prompt asking for the user’s age and then return it to the calling function, which will assign it to a variable called age.
var age = Input("Please enter your age");
Steps to Use @Input()
in Angular Applications
There are three steps to use @Input()
in Angular applications:
-
Add
@Input()
to the HTML element. -
Add the
ng-model
attribute to the element you want to bind with input. -
Assign an expression for the
ng-model
attribute in your controller.
TypeScript code:
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
isFocusStyle = false;
type = 'INFO';
onFocus() {
this.isFocusStyle = true;
this.type = 'INFO';
}
onEnter() {
this.isFocusStyle = false;
const input = document.querySelector('input');
}
}
HTML code:
<h1>Example of Angular Input</h1>
<div class="input-wrapper" [ngClass]="{'focus-style': isFocusStyle}">
<input type="test" placeholder=" Write " (focus)="onFocus()">
</div>
Click here to check the live demonstration of the code as mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook