lock Statement in C#
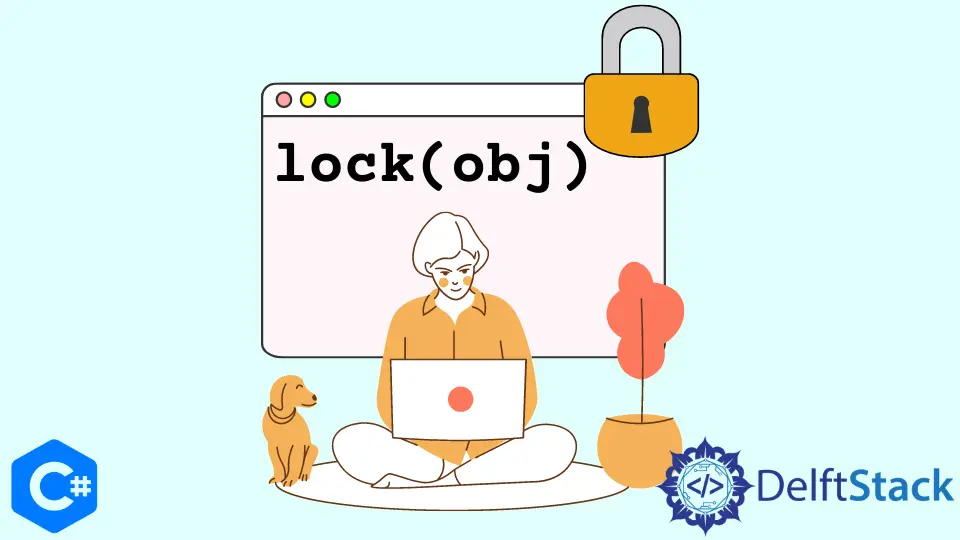
In this tutorial, we will discuss the lock
statement in C#.
the lock
Statement in C#
The lock(obj)
statement specifies that the following section of code cannot be accessed by more than one thread at the same time in C#. The obj
parameter inside the lock(obj)
statement is an instance of the object
class. The lock(obj)
statement provides an efficient way of managing threads in C#. If the code inside the lock(obj)
is accessed by one thread and another thread wants to access the same code, the second thread will have to wait for the first thread to execute it. The lock(obj)
statement ensures sequential execution of the specified section of code. To demonstrate this phenomenon, we will first show you the code’s outcome without any thread management with the lock(obj)
statement.
using System;
using System.Threading;
namespace lock_statement {
class Program {
static readonly object lockname = new object();
static void display() {
for (int a = 1; a <= 3; a++) {
Console.WriteLine("The value to be printed is: {0}", a);
}
}
static void Main(string[] args) {
Thread firstthread = new Thread(display);
Thread secondthread = new Thread(display);
firstthread.Start();
secondthread.Start();
}
}
}
Output:
The value to be printed is: 1
The value to be printed is: 1
The value to be printed is: 2
The value to be printed is: 3
The value to be printed is: 2
The value to be printed is: 3
As we can see, the threads firstthread
and secondthread
both access the code inside the loop randomly. We will show you the outcome of the code with thread management with the lock(obj)
statement.
using System;
using System.Threading;
namespace lock_statement {
class Program {
static readonly object lockname = new object();
static void display() {
lock (lockname) {
for (int a = 1; a <= 3; a++) {
Console.WriteLine("The value to be printed is: {0}", a);
}
}
}
static void Main(string[] args) {
Thread firstthread = new Thread(display);
Thread secondthread = new Thread(display);
firstthread.Start();
secondthread.Start();
}
}
}
Output:
The value to be printed is: 1
The value to be printed is: 2
The value to be printed is: 3
The value to be printed is: 1
The value to be printed is: 2
The value to be printed is: 3
This time, the code inside the loop is sequentially accessed by both firstthread
and secondthread
. We can place code in which we change a variable’s value inside a lock(obj)
statement to prevent any data loss.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn