Pandas DataFrame.reset_index() Function
Minahil Noor
Jan 30, 2023
Pandas
Pandas DataFrame
-
Syntax of
pandas.DataFrame.replace_index()
: -
Example Codes:
DataFrame.reset_index()
Method to Reset the Index of a Dataframe -
Example Codes:
DataFrame.reset_index()
Method to Reset the Index of a MultiIndex Dataframe
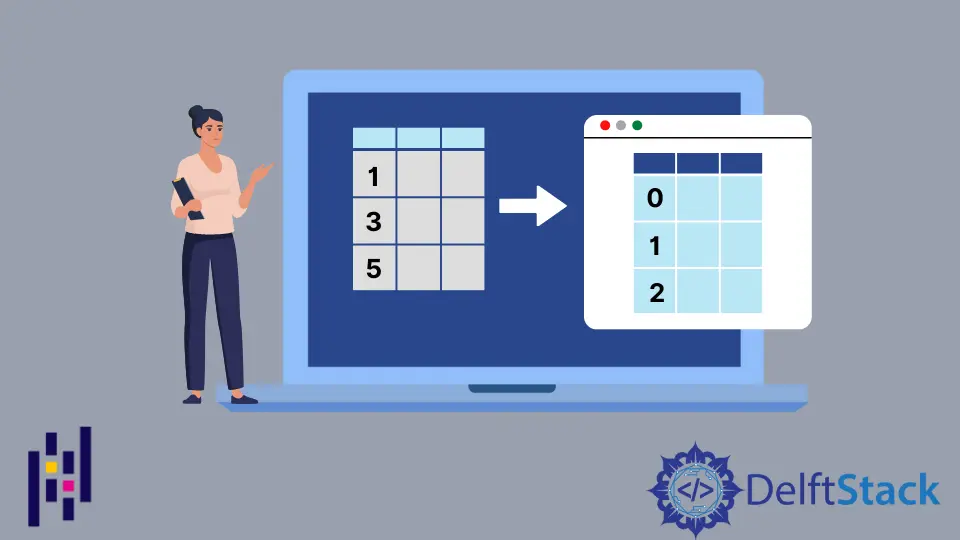
Python Pandas DataFrame.reset_index()
function resets the index of the given DataFrame. It replaces the old index with the default index. If the given DataFrame has a MultiIndex, then this method removes all levels.
Syntax of pandas.DataFrame.replace_index()
:
DataFrame.replace_index(level=None, drop=False, inplace=False, col_level=0, col_fill="")
Parameters
level |
It is an integer, string, tuple, or list type parameter. If passed, then the function will remove the passed level. |
drop |
It is a Boolean parameter. It specifies inserting index into DataFrame column. It resets the index to the default integer index. |
inplace |
It is a Boolean parameter. It specifies modifying the given DataFrame or creating a new object. |
col_level |
It is an integer or string type parameter. It tells which level the labels are inserted into if the columns have multiple levels. |
col_fill |
It is an object type parameter. It tells how the other levels are named if the columns have multiple levels. |
Return
It returns the Dataframe with the new index or None if inplace=True
.
Example Codes: DataFrame.reset_index()
Method to Reset the Index of a Dataframe
import pandas as pd
dataframe=pd.DataFrame({'Attendance': {0: 60, 1: 100, 2: 80,3: 78,4: 95},
'Name': {0: 'Olivia', 1: 'John', 2: 'Laura',3: 'Ben',4: 'Kevin'},
'Obtained Marks': {0: 90, 1: 75, 2: 82, 3: 64, 4: 45}})
print("The Original Data frame is: \n")
print(dataframe)
dataframe1 = dataframe.reset_index()
print("The Modified Data frame is: \n")
print(dataframe1)
Output:
The Original Data frame is:
Attendance Name Obtained Marks
0 60 Olivia 90
1 100 John 75
2 80 Laura 82
3 78 Ben 64
4 95 Kevin 45
The Modified Data frame is:
index Attendance Name Obtained Marks
0 0 60 Olivia 90
1 1 100 John 75
2 2 80 Laura 82
3 3 78 Ben 64
4 4 95 Kevin 45
The function has returned the DataFrame with a new index.
If you do not wish to see another index column, then you can set the parameter drop= True
. It will reset the index to the default index column.
import pandas as pd
dataframe=pd.DataFrame({'Attendance': {0: 60, 1: 100, 2: 80,3: 78,4: 95},
'Name': {0: 'Olivia', 1: 'John', 2: 'Laura',3: 'Ben',4: 'Kevin'},
'Obtained Marks': {0: 90, 1: 75, 2: 82, 3: 64, 4: 45}})
print("The Original Data frame is: \n")
print(dataframe)
dataframe1 = dataframe.reset_index(drop= True)
print("The Modified Data frame is: \n")
print(dataframe1)
Output:
The Original Data frame is:
Attendance Name Obtained Marks
0 60 Olivia 90
1 100 John 75
2 80 Laura 82
3 78 Ben 64
4 95 Kevin 45
The Modified Data frame is:
Attendance Name Obtained Marks
0 60 Olivia 90
1 100 John 75
2 80 Laura 82
3 78 Ben 64
4 95 Kevin 45
Example Codes: DataFrame.reset_index()
Method to Reset the Index of a MultiIndex Dataframe
import pandas as pd
import numpy as np
index = pd.MultiIndex.from_tuples([(1, 'Sarah'),
(1, 'Peter'),
(2, 'Harry'),
(2, 'Monika')],
names=['class', 'name'])
columns = pd.MultiIndex.from_tuples([('Performance', 'max'),
('Grade', 'type')])
dataframe = pd.DataFrame([('Good', 'A'),
( 'Best', 'A+'),
( 'Bad', 'C'),
(np.nan, 'F')],
index=index,
columns=columns)
print("The Original Data frame is: \n")
print(dataframe)
dataframe1 = dataframe.reset_index(drop= True)
print("The Modified Data frame is: \n")
print(dataframe1)
Output:
The Original Data frame is:
Performance Grade
max type
class name
1 Sarah Good A
Peter Best A+
2 Harry Bad C
Monika NaN F
The Modified Data frame is:
Performance Grade
max type
0 Good A
1 Best A+
2 Bad C
3 NaN F
The function has reset the index and added the default integer index.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe