在 Pandas 中加载 JSON 文件
- 将 JSON 文件加载到 Pandas DataFrame 中
- 将面向索引的 JSON 文件加载到 Pandas DataFrame 中
- 将面向列的 JSON 文件加载到 Pandas DataFrame 中
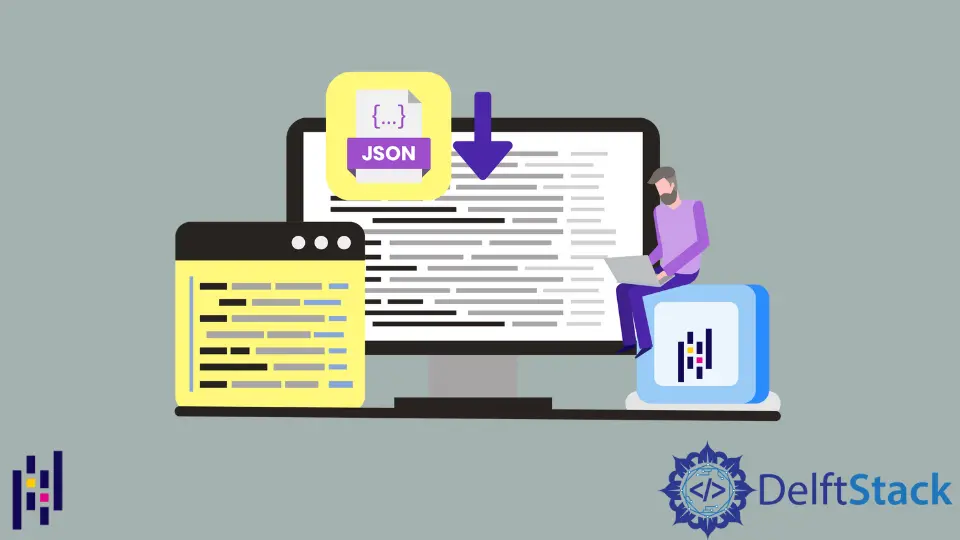
本教程介绍了如何使用 pandas.read_json()
方法将一个 JSON 文件加载到 Pandas DataFrame 中。
将 JSON 文件加载到 Pandas DataFrame 中
我们可以使用 pandas.read_json()
函数将 JSON 文件的路径作为参数传递给 pandas.read_json()
函数,将 JSON 文件加载到 Pandas DataFrame 中。
{
"Name": {"1": "Anil", "2": "Biraj", "3": "Apil", "4": "Kapil"},
"Age": {"1": 23, "2": 25, "3": 28, "4": 30},
}
示例 data.json
文件的内容如上所示。我们将从上述 JSON 文件中创建一个 DataFrame。
import pandas as pd
df = pd.read_json("data.json")
print("DataFrame generated using JSON file:")
print(df)
输出:
DataFrame generated using JSON file:
Name Age
1 Anil 23
2 Biraj 25
3 Apil 28
4 Kapil 30
它显示的是由 data.json
文件中的数据生成的 DataFrame。我们必须确保在当前工作目录下有 data.json
文件才能生成 DataFrame,否则我们需要提供 JSON 文件的完整路径作为 pandas.read_json()
方法的参数。
由 JSON 文件形成的 DataFrame 取决于 JSON 文件的方向。我们一般有三种不同的 JSON 文件的面向。
- 面向索引
- 面向值
- 面向列
将面向索引的 JSON 文件加载到 Pandas DataFrame 中
{
"0": {"Name": "Anil", "Age": 23},
"1": {"Name": "Biraj", "Age": 25},
"2": {"Name": "Apil", "Age": 26},
}
这是一个面向索引的 JSON 文件的例子,其中顶层键代表数据的索引。
import pandas as pd
df = pd.read_json("data.json")
print("DataFrame generated from Index Oriented JSON file:")
print(df)
输出:
DataFrame generated from Index Oriented JSON file:
0 1 2
Name Anil Biraj Apil
Age 23 25 26
它将从 data.json
文件中创建一个 DataFrame,顶层键在 DataFrame 中表示为列。
将面向值的 JSON 文件加载到 Pandas DataFrame 中
[["Anil", 23], ["Biraj", 25], ["Apil", 26]]
这是一个面向值的 JSON 文件的例子,数组中的每个元素代表每一行的值。
import pandas as pd
df = pd.read_json("data.json")
print("DataFrame generated from Value Oriented JSON file:")
print(df)
输出:
DataFrame generated from Value Oriented JSON file:
0 1
0 Anil 23
1 Biraj 25
2 Apil 26
它将从 data.json
文件中创建一个 DataFrame,JSON 文件中数组的每个元素将在 DataFrame 中表示为一行。
将面向列的 JSON 文件加载到 Pandas DataFrame 中
{"Name": {"1": "Anil", "2": "Biraj", "3": "Apil"}, "Age": {"1": 23, "2": 25, "3": 28}}
它是一个面向列的 JSON 文件顶层索引的例子,代表数据的列名。
import pandas as pd
df = pd.read_json("data.json")
print("DataFrame generated from Column Oriented JSON file:")
print(df)
输出:
DataFrame generated from Column Oriented JSON file:
Name Age
1 Anil 23
2 Biraj 25
3 Apil 28
它将从 data.json
文件中创建一个 DataFrame,JSON 文件的顶层索引将作为 DataFrame 中的列名。
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn