如何在 PHP 中获取字符串的最后一个字符
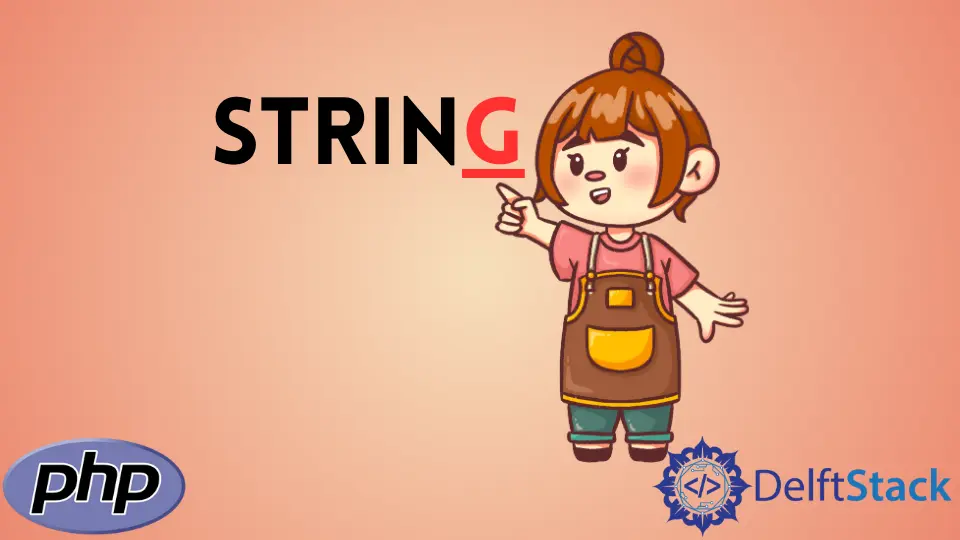
在本文中,我们将介绍获取字符串的最后一个字符的方法。
- 使用
substr()
函数 - 通过直接字符串访问
- 使用
for
循环
使用 substr()
函数获取 PHP 中字符串的最后一个字符
PHP 中内置的 substr()
函数可以获取字符串的子字符串。此函数具有三个参数,其中一个是可选参数。使用此函数的正确语法如下
substr($stringName, $startingPosition, $lengthOfSubstring);
$stringName
是输入字符串。$startngPosition
是子字符串的起始索引。$lengthOfSubstring
是子字符串的长度。变量 $stringName
和 $startingPosition
是必需参数,而 $lengthOfSubstring
是可选参数。当省略 $lengthOfSubstring
时,子字符串将从 $startngPosition
开始,直到输入字符串的末尾。
<?php
$string = 'This is a string';
$lastChar = substr($string, -1);
echo "The last char of the string is $lastChar.";
?>
如果 $startingPosition
具有正值,则子字符串从字符串的左侧开始,即字符串的开头。如果 $startingPosition
是负数的话,则子字符串从字符串的右侧开始,即字符串的结尾。
为了获得字符串的最后一个字符,这里我们将 -1
作为 $startingPosition
的值。-1
表示字符串的最后一个字符。
输出:
The last char of the string is g.
使用直接字符串访问获取 PHP 中字符串的最后一个字符
字符串是一个字符数组。我们可以通过考虑字符串的最后一个字符来获取字符串的最后一个字符。
<?php
$string = "This is a string";
$lengthOfString = strlen($string);
$lastCharPosition = $lengthOfString-1;
$lastChar = $string[$lastCharPosition];
echo "The last char of the string is $lastChar.";
?>
字符串的最后一个字符的索引比字符串的长度小 1,因为索引从 0 开始。$lengthOfString
使用 strlen()
函数。$lastCharPosition
存放着字符串的最后一个字符的索引。输出是通过传递 $lastCharPosition
作为字符数组的索引之后的最后一个字符 char
。
输出:
The last char of the string is g.
从 PHP 7.1.0 进行负字符串索引访问
从 PHP 7.1.0 开始,字符串的负索引也开始支持。因此,我们可以使用-1 作为索引来获取 PHP 中字符串的最后一个字符。
示例代码:
<?php
$string = "This is a string";
$lastChar = $string[-1];
echo "The last char of the string is $lastChar.";
?>
输出:
The last char of the string is g.
使用 for
循环获取 PHP 中字符串的最后一个字符
我们可以通过将字符串的最后一个 char 存储到新的字符串中,然后使用 for
循环获取最后一个 char。我们将遍历字符串并到达最后一个字符。
<?php
$string = "This is a string";
$lastCharPosition = strlen($string) - 1;
for ($x = $lastCharPosition; $x < strlen($string); $x++) {
$newString = $string[$x];
}
echo "The last char of the string is $newString.";
?>
我们将使用 strlen()
函数获得最后一个字符的索引,并在 for
循环中将其分配给 $x
。
输出:
The last char of the string is g.