JavaFX 文本旋转
Sheeraz Gul
2024年2月15日
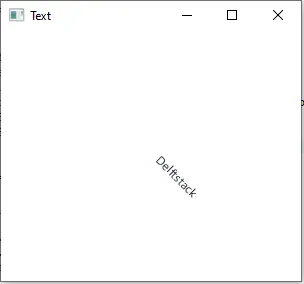
可以使用 JavaFX.scene.text.Text
类创建文本节点,并在 JavaFX 中使用 setRotate()
来旋转文本。本教程演示了如何在 JavaFX 中旋转文本。
JavaFX 文本旋转
JavaFX 中使用 setRotate()
方法来旋转文本。文本节点放置在场景中,并将围绕 x
和 y
位置旋转。
在实例化 Text
类时,这些 x
和 y
位置被传递到文本中。
语法:
text.setRotate(int);
上述语法中的 int
是文本的旋转值。以下是在 JavaFX 中旋转文本的步骤。
-
创建一个扩展
Application
类的类。 -
在
start
方法中将标题设置为舞台。 -
通过实例化
Group
类来创建一个组。 -
通过实例化
Scene
类并将Group
传递给它来创建场景。 -
用给定的值初始化
x
、y
和RGB
。 -
通过实例化
Text
类并传递x
、y
和text
值来创建文本。 -
通过
SetFill()
方法为文本填充颜色。 -
使用
setRotate()
方法设置旋转度数。 -
将
文本
添加到组
。 -
将场景传递到舞台并通过
Show
方法显示舞台并在 main 方法中启动应用程序。
让我们尝试根据上述步骤实现一个示例。
完整源代码:
package delftstack;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class JavaFX_TextRotate extends Application {
@Override
public void start(Stage Demo_Stage) {
Demo_Stage.setTitle("Text");
Group Group_Root = new Group();
Scene Demo_Scene = new Scene(Group_Root, 300, 250, Color.WHITE);
int x = 150;
int y = 150;
int RED = 30;
int GREEN = 40;
int BLUE = 50;
Text Demo_Text = new Text(x, y, "Delftstack");
Demo_Text.setFill(Color.rgb(RED, GREEN, BLUE, .99));
Demo_Text.setRotate(45);
Group_Root.getChildren().add(Demo_Text);
Demo_Stage.setScene(Demo_Scene);
Demo_Stage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}
上面的代码会将文本旋转 45 度。
输出:
这是另一个基于随机 x
和 y
位置和随机旋转度数旋转多个文本的示例。
示例代码:
package delftstack;
import java.util.Random;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class JavaFX_Rotate extends Application {
@Override
public void start(Stage Demo_Stage) {
Demo_Stage.setTitle("TEXT");
Group Group_Root = new Group();
Scene Demo_Scene = new Scene(Group_Root, 300, 250, Color.WHITE);
Random Random_Number = new Random(System.currentTimeMillis());
for (int i = 0; i < 100; i++) {
int x = Random_Number.nextInt((int) Demo_Scene.getWidth());
int y = Random_Number.nextInt((int) Demo_Scene.getHeight());
int RED = Random_Number.nextInt(255);
int GREEN = Random_Number.nextInt(255);
int BLUE = Random_Number.nextInt(255);
Text Demo_Text = new Text(x, y, "Delftstack");
int Rotation = Random_Number.nextInt(180);
Demo_Text.setFill(Color.rgb(RED, GREEN, BLUE, .99));
Demo_Text.setRotate(Rotation);
Group_Root.getChildren().add(Demo_Text);
}
Demo_Stage.setScene(Demo_Scene);
Demo_Stage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}
上面的代码将使用随机的 x
、y
、RGB
和旋转度数旋转多个文本。
输出:
作者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook