Rotación de texto JavaFX
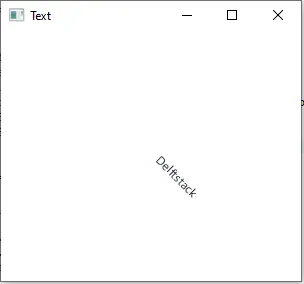
Los nodos de texto se pueden crear usando la clase JavaFX.scene.text.Text
, y para rotar el texto usamos setRotate()
en JavaFX. Este tutorial demuestra cómo rotar texto en JavaFX.
Rotación de texto JavaFX
El método setRotate()
se usa en JavaFX para rotar el texto. Los nodos de texto se colocan en la escena y se rotarán alrededor de las posiciones x
e y
.
Estas posiciones x
e y
se pasan al texto cuando se crea una instancia de la clase Text
.
Sintaxis:
text.setRotate(int);
El int
en la sintaxis anterior es el valor de rotación del texto. Los siguientes son los pasos para rotar el texto en JavaFX.
-
Cree una clase que amplíe la clase
Application
. -
Establezca el título en el escenario en el método
start
. -
Cree un grupo instanciando la clase
Group
. -
Cree una escena instanciando la clase
Scene
y pasándole elGroup
. -
Inicialice la
x
,y
yRGB
con los valores dados. -
Cree el texto instanciando la clase
Text
y pasando los valoresx
,y
ytext
. -
Rellene el color del texto con el método
SetFill()
. -
Establezca el grado de rotación utilizando el método
setRotate()
. -
Añade el
text
algroup
. -
Pase la escena al escenario y visualice el escenario mediante el método
Show
e inicie la aplicación en el métodomain
.
Intentemos implementar un ejemplo basado en los pasos anteriores.
Código fuente completo:
package delftstack;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class JavaFX_TextRotate extends Application {
@Override
public void start(Stage Demo_Stage) {
Demo_Stage.setTitle("Text");
Group Group_Root = new Group();
Scene Demo_Scene = new Scene(Group_Root, 300, 250, Color.WHITE);
int x = 150;
int y = 150;
int RED = 30;
int GREEN = 40;
int BLUE = 50;
Text Demo_Text = new Text(x, y, "Delftstack");
Demo_Text.setFill(Color.rgb(RED, GREEN, BLUE, .99));
Demo_Text.setRotate(45);
Group_Root.getChildren().add(Demo_Text);
Demo_Stage.setScene(Demo_Scene);
Demo_Stage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}
El código anterior rotará el texto a 45 grados.
Producción:
Aquí hay otro ejemplo que gira los múltiples textos en función de las posiciones aleatorias x
e y
y los grados aleatorios de rotación.
Código de ejemplo:
package delftstack;
import java.util.Random;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class JavaFX_Rotate extends Application {
@Override
public void start(Stage Demo_Stage) {
Demo_Stage.setTitle("TEXT");
Group Group_Root = new Group();
Scene Demo_Scene = new Scene(Group_Root, 300, 250, Color.WHITE);
Random Random_Number = new Random(System.currentTimeMillis());
for (int i = 0; i < 100; i++) {
int x = Random_Number.nextInt((int) Demo_Scene.getWidth());
int y = Random_Number.nextInt((int) Demo_Scene.getHeight());
int RED = Random_Number.nextInt(255);
int GREEN = Random_Number.nextInt(255);
int BLUE = Random_Number.nextInt(255);
Text Demo_Text = new Text(x, y, "Delftstack");
int Rotation = Random_Number.nextInt(180);
Demo_Text.setFill(Color.rgb(RED, GREEN, BLUE, .99));
Demo_Text.setRotate(Rotation);
Group_Root.getChildren().add(Demo_Text);
}
Demo_Stage.setScene(Demo_Scene);
Demo_Stage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}
El código de arriba rotará múltiples textos usando la x
, y
, RGB
aleatoria y el grado de rotación.
Producción:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook