C# 中的列表数组
Muhammad Maisam Abbas
2023年10月12日
Csharp
Csharp Array
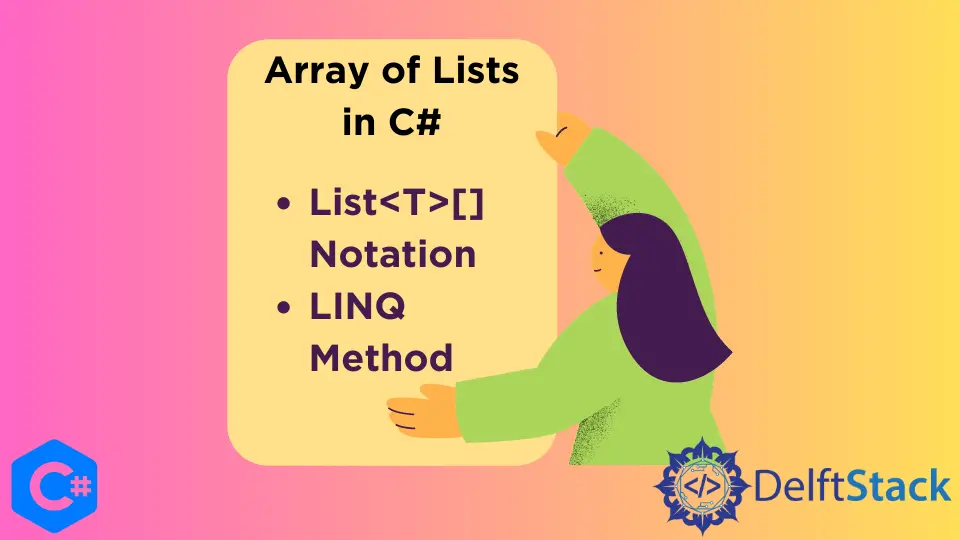
本教程将讨论在 C# 中创建列表数组的方法。
C# 中带有 List<T>[]
表示法的列表数组
List<T>[]
表示法可用于声明 C# 中 T
类型的列表的数组。请记住,这只会声明一个空引用数组。我们仍然必须使用 new
关键字在 List<T>[]
的每个索引处初始化每个列表。
using System;
using System.Collections.Generic;
namespace array_of_lists {
class Program {
static void Main(string[] args) {
List<int>[] arrayList = new List<int>[3];
arrayList[0] = new List<int> { 1, 2, 3 };
arrayList[1] = new List<int> { 4, 5, 6 };
arrayList[2] = new List<int> { 7, 8, 9 };
foreach (var list in arrayList) {
foreach (var element in list) {
Console.WriteLine(element);
}
}
}
}
}
输出:
1
2
3
4
5
6
7
8
9
在上面的代码中,我们用 C# 中的 List<T>[]
表示法和 new
关键字声明并初始化了包含整数值的列表 arrayList
的数组。对于小型数组,上述方法是可以的。但是,如果我们的数组很大,则此方法可能会变得非常费力,并且会占用大量代码。此方法仅适用于长度较小的数组。
C# 中使用 LINQ 方法的列表数组
LINQ 用于将查询功能与 C# 中的数据结构集成在一起。我们可以使用 LINQ 在 C# 中声明和初始化列表数组。
using System;
using System.Collections.Generic;
namespace array_of_lists {
class Program {
static void Main(string[] args) {
List<int>[] arrayList = new List<int> [3].Select(item => new List<int> { 1, 2, 3 }).ToArray();
foreach (var list in arrayList) {
foreach (var element in list) {
Console.WriteLine(element);
}
}
}
}
}
输出:
1
2
3
1
2
3
1
2
3
在上面的代码中,我们用 C# 中的 List<T>[]
表示法和 new
关键字声明并初始化了包含整数值的列表 arrayList
的数组。对于较大的数组,此方法比以前的示例劳动强度低,因此它更适合于具有较大长度的数组。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn