在 C# 中对整数数组求和
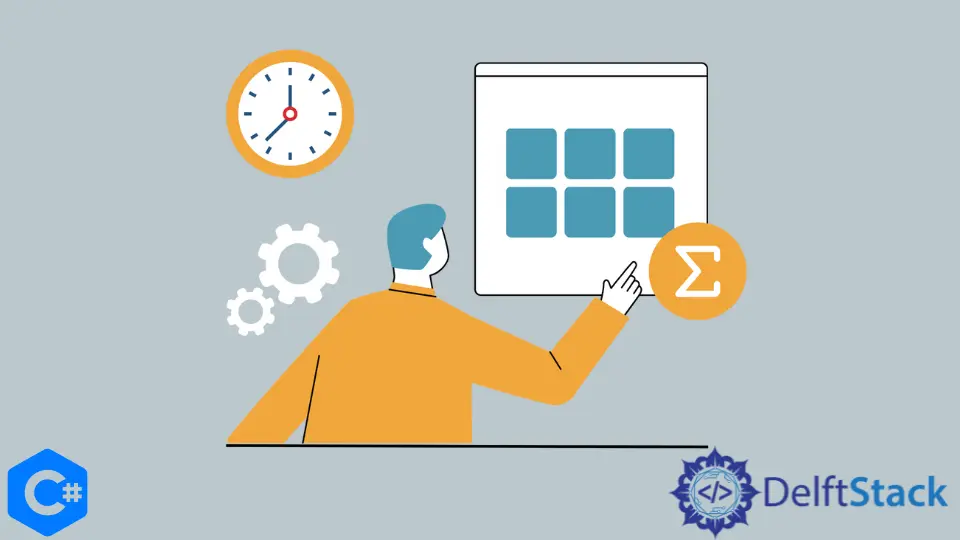
本文将介绍如何在 C# 中对整数数组求和。
使用 sum()
方法对 C#
中的整数数组求和
IEnumerable
派生自 System.Collections.Generic
命名空间。它是一个定义 GetEnumerator
函数的接口。它允许循环通过一组类或匿名类型列表。
sum() 方法是在 System.Linq
命名空间中找到的扩展方法。此方法将 IEnumerable
中的所有数值相加,如列表或数组。
sum()
方法可用于实现 IEnumerable
的对象,该对象具有所有 C# 数字数据类型,如 int
、long
、double
和 decimal
。这是一种通过避免循环来添加数字集合的优化方式。
这种方法鼓励更少的代码行,并可能减少错误,但有一些开销使其比 for
循环慢。
下面是使用 sum()
的代码示例。
using System;
using System.Linq;
namespace MyApplication {
class Program {
static void Main(string[] args) {
int[] arr = new int[] { 1, 2, 3 };
int sum = arr.Sum();
Console.WriteLine(sum);
}
}
}
输出:
6
使用 Array.foreach
方法对 C#
中的整数数组求和
foreach
语句是一种简洁且不太复杂的遍历数组元素的方法。foreach
方法以升序处理一维数组的元素,从索引 0 处的元素开始到索引 array.length
- 1 处的元素。
delegate
是一种类型安全且安全的引用类型。它用于封装命名或匿名方法。
必须使用具有兼容返回类型的方法或 lambda 表达式来实例化委托。我们使用嵌套在 foreach
语句中的 lambda 表达式。
下面是使用 foreach
方法的代码示例。
using System;
namespace MyApplication {
class Program {
static void Main(string[] args) {
int[] arr = new int[] { 1, 2, 3 };
int sum = 0;
Array.ForEach(arr, i => sum += i);
Console.WriteLine(sum);
}
}
}
输出:
6
使用 Enumerable.Aggregate()
方法对 C#
中的整数数组求和
Enumerable.Aggregate
方法存在于 System.Linq
命名空间中。它在跟踪或存储先前结果的同时对列表或数组的每个元素执行数学运算。
例如,我们必须对数组或数字列表 {2,4,6,8} 执行加法运算。Aggregate
函数将 2 和 4 相加,将结果(即 6)向前传递,将该结果与下一个元素 (6+6) 相加,将结果向前传递,然后将其与下一个元素 (12+8) 相加。处理最后一个数字时,它返回最终结果。
下面是一个代码示例。
using System;
using System.Linq;
namespace MyApplication {
class Program {
static void Main(string[] args) {
int[] arr = new int[] { 1, 2, 3 };
int sum = arr.Aggregate((total, next) => total + next);
Console.WriteLine(sum);
}
}
}
输出:
6