如何在 c++ 中查找字符串中的子字符串
Jinku Hu
2023年10月12日
C++
C++ String
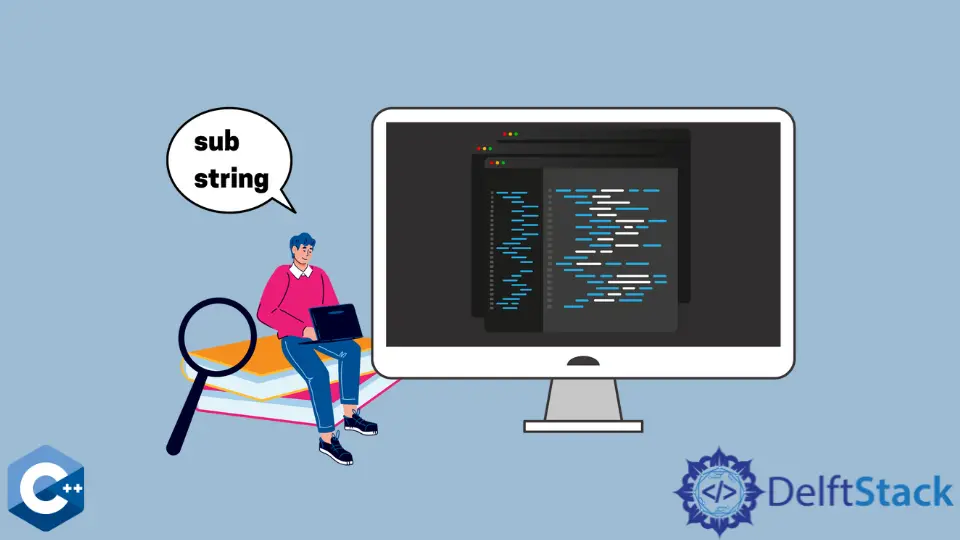
本文演示了在 C++ 中查找字符串中给定子字符串的方法。
使用 find
方法在 C++ 中查找字符串中的子字符串
最直接的解决方法是 find
函数,它是一个内置的字符串方法,它以另一个字符串对象作为参数。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string str1 = "this is random string oiwao2j3";
string str2 = "oiwao2j3";
string str3 = "random s tring";
str1.find(str2) != string::npos
? cout << "str1 contains str2" << endl
: cout << "str1 does not contain str3" << endl;
str1.find(str3) != string::npos
? cout << "str1 contains str3" << endl
: cout << "str1 does not contain str3" << endl;
return EXIT_SUCCESS;
}
输出:
str1 contains str2
str1 does not contain str3
或者,你可以使用 find
来比较两个字符串中的特定字符范围。要做到这一点,你应该把范围的起始位置和长度作为参数传递给 find
方法。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
using std::stoi;
int main() {
string str1 = "this is random string oiwao2j3";
string str3 = "random s tring";
constexpr int length = 6;
constexpr int pos = 0;
str1.find(str3.c_str(), pos, length) != string::npos
? cout << length << " chars match from pos " << pos << endl
: cout << "no match!" << endl;
return EXIT_SUCCESS;
}
输出:
6 chars match from pos 0
使用 rfind
方法在 C++ 中查找字符串中的子字符串
rfind
方法的结构与 find
类似。我们可以利用 rfind
来寻找最后的子字符串,或者指定一定的范围来匹配给定的子字符串。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
using std::stoi;
int main() {
string str1 = "this is random string oiwao2j3";
string str2 = "oiwao2j3";
str1.rfind(str2) != string::npos
? cout << "last occurrence of str3 starts at pos " << str1.rfind(str2)
<< endl
: cout << "no match!" << endl;
return EXIT_SUCCESS;
}
输出:
last occurrence of str3 starts at pos 22
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu