Comment trouver une sous-chaîne en C++
-
Utiliser la méthode
find
pour trouver une sous-chaîne dans une chaîne en C++ -
Utiliser la méthode
rfind
pour trouver une sous-chaîne dans une chaîne en C++
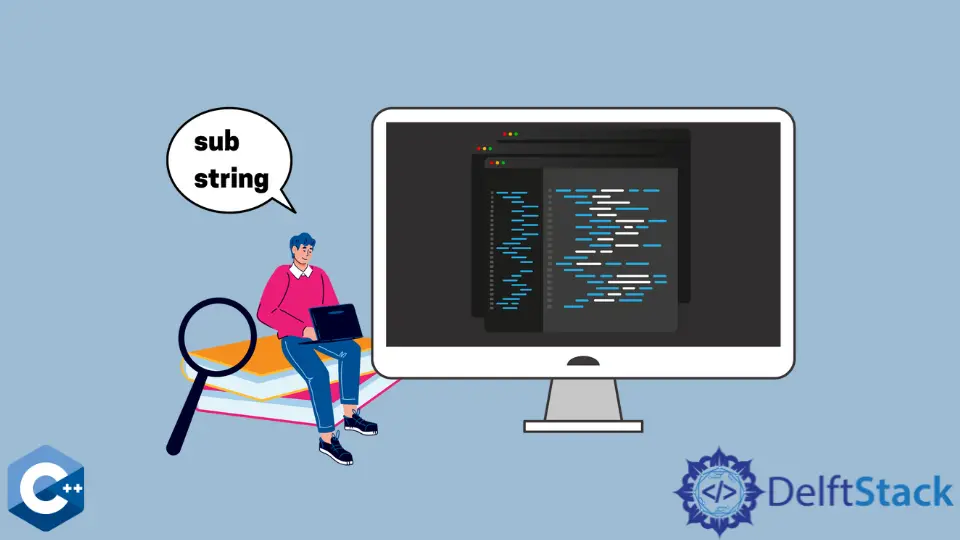
Cet article présente des méthodes pour trouver une sous-chaîne donnée dans une chaîne de caractères en C++.
Utiliser la méthode find
pour trouver une sous-chaîne dans une chaîne en C++
La façon la plus simple de résoudre le problème est la fonction find
, qui est une méthode de chaîne
intégrée, et qui prend un autre objet chaîne
comme argument.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string str1 = "this is random string oiwao2j3";
string str2 = "oiwao2j3";
string str3 = "random s tring";
str1.find(str2) != string::npos
? cout << "str1 contains str2" << endl
: cout << "str1 does not contain str3" << endl;
str1.find(str3) != string::npos
? cout << "str1 contains str3" << endl
: cout << "str1 does not contain str3" << endl;
return EXIT_SUCCESS;
}
Production:
str1 contains str2
str1 does not contain str3
Vous pouvez également utiliser la fonction find
pour comparer des plages de caractères spécifiques dans deux chaînes de caractères. Pour ce faire, vous devez passer la position de départ et la longueur de la plage en argument à la méthode find
:
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
using std::stoi;
int main() {
string str1 = "this is random string oiwao2j3";
string str3 = "random s tring";
constexpr int length = 6;
constexpr int pos = 0;
str1.find(str3.c_str(), pos, length) != string::npos
? cout << length << " chars match from pos " << pos << endl
: cout << "no match!" << endl;
return EXIT_SUCCESS;
}
Production:
6 chars match from pos 0
Utiliser la méthode rfind
pour trouver une sous-chaîne dans une chaîne en C++
La méthode rfind
a une structure similaire à celle de find
. Nous pouvons utiliser rfind
pour trouver la dernière sous-chaîne ou spécifier une certaine plage pour la faire correspondre à la sous-chaîne donnée.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
using std::stoi;
int main() {
string str1 = "this is random string oiwao2j3";
string str2 = "oiwao2j3";
str1.rfind(str2) != string::npos
? cout << "last occurrence of str3 starts at pos " << str1.rfind(str2)
<< endl
: cout << "no match!" << endl;
return EXIT_SUCCESS;
}
Production:
last occurrence of str3 starts at pos 22
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookArticle connexe - C++ String
- Comparaison de chaîne et de caractère en C++
- Supprimer le dernier caractère d'une chaîne en C++
- Obtenir le dernier caractère d'une chaîne en C++
- Différences entre l'opérateur sizeof et la fonction strlen pour les chaînes en C++
- Effacer la chaîne en C++
- Imprimer toutes les permutations de la chaîne en C++