在 React 中為元件設定預設 props
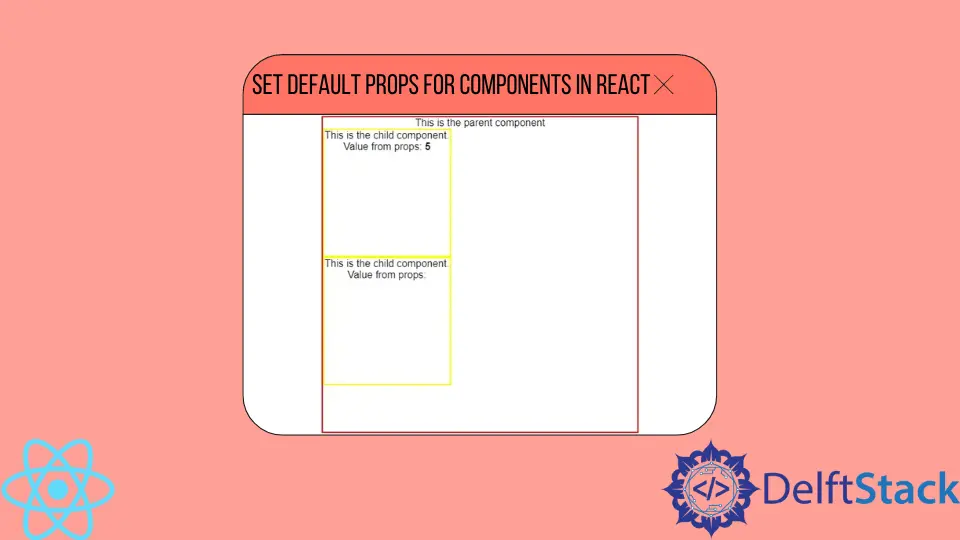
React 是當今使用最廣泛的 UI 庫。該庫是圍繞虛擬 DOM 和元件等概念設計的,以實現高效的程式碼可重用性。
React Web 應用程式由元件組成,這些元件是 UI 的小型構建塊。它們中的每一個都可以具有自定義的外觀和功能。
你需要在許多小元件之間拆分功能,以便為你的應用提供某些功能。出於這個原因,React 提供了多種方式來在它們之間共享資料和函式。
使用 props
是從父元件向子元件共享資料的最直接方式之一。
React 中的預設 props
在 React 中,通過 props 從父元件接收資料並顯示在子元件中是很常見的。子元件接受 props
作為引數,在 JSX 中,它們使用 props
中的資料來顯示資訊。
在 React 中,通常使用子元件根據通過 props
提供給它們的資料來顯示圖表、文字和其他視覺效果。React 建立在程式碼可重用性之上,因此同一個子元件會有很多例項。
在某些情況下,來自父元件的必要資料將被傳遞給子元件,但有時子元件可能不會收到任何資料。在這種情況下,有必要設定預設 props 來顯示一些值,即使子元件沒有收到任何資料。
讓我們看一個例子。
export default function App() {
return (
<div
className="App"
style={{ width: 500, height: 500, border: "2px solid red" }}
>
This is the parent component
<Child number={5}></Child>
<Child></Child>
</div>
);
}
function Child(props) {
return (
<div style={{ width: 200, height: 200, border: "2px solid yellow" }}>
This is the child component. Value from props: <b>{props.number}</b>
</div>
);
}
如果沒有預設的 props,沒有接收到 props
但在 JSX 中顯示 props
值的子元件將不會顯示任何內容。這個例子渲染了兩個子元件,一個帶有 props
,一個沒有。
讓我們看一下輸出。
什麼都不顯示不是一個好的使用者體驗實踐。你的使用者可能認為你的 React 應用程式出現故障。
如果你的子元件在理想情況下沒有收到任何 props,你應該顯示一條訊息來傳達這一點。
在 React 中為功能元件設定預設 props
功能元件提供了一種簡單的語法來呈現視覺元素。從 React 16.8 版開始,你甚至可以實現狀態功能並在元件的不同生命週期上執行功能。
你可以使用 defaultProps
屬性為功能元件設定預設 props。讓我們來看看。
export default function App() {
return (
<div
className="App"
style={{ width: 500, height: 500, border: "2px solid red" }}
>
This is the parent component
<Child number={5}></Child>
<Child></Child>
</div>
);
}
function Child(props) {
return (
<div style={{ width: 200, height: 200, border: "2px solid yellow" }}>
This is the child component. Number from props: <b>{props.number}</b>
</div>
);
}
Child.defaultProps = {
number: "number not passed"
};
你可以在 CodeSandbox 上檢視演示。
在這種情況下,沒有收到任何 props 的子元件的第二個例項將顯示字串 "number not pass"
。它不會像沒有 defaultProps
時那樣為空。
當我們提供 props
時,子元件將顯示我們傳遞的值。未通過 props
物件傳遞的值的預設 props 替代。
在 React 中為類元件設定預設 props
類元件的語法不同於功能元件。React 開發人員仍然必須使用 defaultProps
屬性來設定預設 props,但實現方式不同。
這是一個在類元件上設定 defaultProps
屬性的簡單示例。
class Child extends React.Component {
constructor(props) {}
static defaultProps = {
number: "number not passed"
}
render() {
return <div>
<div style={{ width: 200, height: 200, border: "2px solid yellow" }}>
This is the child component. Number from props: <b>{this.props.number}</b>
</div>
</div>
}
}
此類元件將具有與上述功能元件相同的輸出。
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn