在 React 中將 props 傳遞給孩子
- 在 React 中將 props 傳遞給孩子
-
使用
Context
API 將 props 傳遞給 React 中的孩子 -
在 React 中使用
React.cloneElement()
將 props 傳遞給兒童
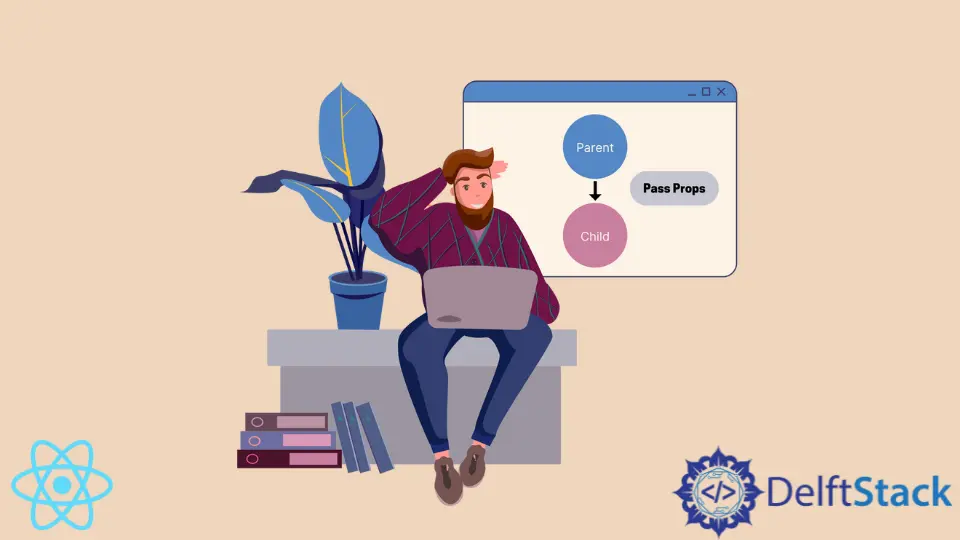
React 開發人員依靠元件的可重用性來構建功能強大且可維護的應用程式。
react 庫具有組合模型,這增加了利用可重用元件的靈活性。在某些情況下,你無法預測元件的子元件將是什麼。
例如,在構建訊息應用程式時,你的對話方塊可能包含表情符號和一段文字。
React 提供了一個 props.children
特性來增加元件的可重用性。簡單來說,React 開發人員可以使用 this.props.children
來顯示放置在父元件的開始和結束標記之間的值(通常是 UI 元素)。
在 React 中將 props 傳遞給孩子
假設你有通過 props.children
接收的子元素。要顯示它們,你需要在 return
語句中包含 props.children
或 this.props.children
(用於類元件)。
這是一個包含三個元件的示例:頂部的父元件 App
和帶有子 Text
元件的 Box
元件。讓我們來看看:
class App extends Component {
render() {
return <Box>
<Text></Text>
</Box>;
}
}
class Box extends Component {
render() {
return <div>{this.props.children}</div>;
}
}
class Text extends Component {
render() {
return <p>Welcome to App</p>;
}
}
但是如果你需要將額外的 props 傳遞給包含在 props.children
中的元素或元件怎麼辦?在我們的示例中,我們可能需要為文字指定 color
或 fontSize
屬性。
讓我們探索兩個很好的選擇來做到這一點。
使用 Context
API 將 props 傳遞給 React 中的孩子
Context
允許你將 props 傳遞給整個元件樹。它非常有用,因為你在頂部有一個 App
元件,但想要將事件處理程式傳遞給樹底部的子元件。
你可以手動傳遞它,但這會非常耗時且難以追蹤。相反,你可以使用 Context
使每個子元件中的值都可用。
從技術上講,Context
與 props
不同,但它可以在我們的情況下完成工作。為此,我們將使用可在所有 React 應用程式中使用的 React.createContext()
方法。
首先,我們定義一個變數來儲存 Context
例項:
import React, { Component } from 'react';
import { render } from 'react-dom';
const SampleContext = React.createContext()
class App extends Component {
render() {
return <Box>
<Text></Text>
</Box>;
}
}
請注意,該變數是在類元件的範圍之外建立的。
我們找到顯示 this.props.children
的元件並用 <SampleContext.Provider>
包裹它。包裝器的開始和結束標記之間的所有內容都可以訪問我們定義的值。
然後我們指定值:
class Box extends Component {
render() {
return <SampleContext.Provider value={{color: "red"}}>
{this.props.children}
</SampleContext.Provider>;
}
}
現在,我們轉到像 this.props.children
這樣被傳遞的元件,並將 contextType
屬性設定為等於我們最初建立的變數:
class Text extends Component {
static contextType = SampleContext
render() {
return <p style={{color: this.context.color}}>Welcome to App</p>;
}
}
一旦我們這樣做了,我們就可以從 Context
訪問值。在這種情況下,我們定義了一個內聯樣式物件並將 color
屬性設定為 this.context.color
,紅色。
文字確實是紅色
,如播放程式碼中所見。你可以嘗試自己編輯程式碼,方法是在 Context
中新增或刪除屬性並檢視它是否有效。
在 React 中使用 React.cloneElement()
將 props 傳遞給兒童
或者,你也可以使用 cloneElement()
方法將自定義 props 新增到你的 props.children
中的元素或元件。我們來看一個例子,消化一下:
<>{React.cloneElement(props.children, {color: "red"})}</>
在我們的示例中,該方法接受兩個引數。首先,我們必須傳遞給它 props.children
,它將用作克隆的起點。這意味著克隆的元素將擁有原始元素的所有 props
。
cloneElement()
方法還保留了 refs,這在與 DOM 互動時很重要。
作為第二個引數,我們必須傳遞我們希望元件接收的所有附加 props。在這種情況下,我們將傳遞一個值為 "red"
的 color
prop。
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn