在 React 中为组件设置默认 props
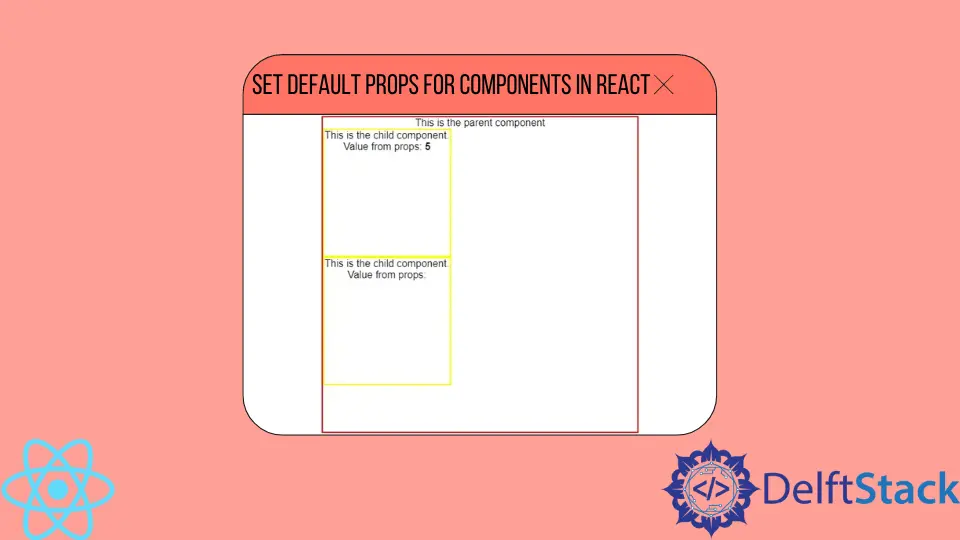
React 是当今使用最广泛的 UI 库。该库是围绕虚拟 DOM 和组件等概念设计的,以实现高效的代码可重用性。
React Web 应用程序由组件组成,这些组件是 UI 的小型构建块。它们中的每一个都可以具有自定义的外观和功能。
你需要在许多小组件之间拆分功能,以便为你的应用提供某些功能。出于这个原因,React 提供了多种方式来在它们之间共享数据和函数。
使用 props
是从父组件向子组件共享数据的最直接方式之一。
React 中的默认 props
在 React 中,通过 props 从父组件接收数据并显示在子组件中是很常见的。子组件接受 props
作为参数,在 JSX 中,它们使用 props
中的数据来显示信息。
在 React 中,通常使用子组件根据通过 props
提供给它们的数据来显示图表、文本和其他视觉效果。React 建立在代码可重用性之上,因此同一个子组件会有很多实例。
在某些情况下,来自父组件的必要数据将被传递给子组件,但有时子组件可能不会收到任何数据。在这种情况下,有必要设置默认 props 来显示一些值,即使子组件没有收到任何数据。
让我们看一个例子。
export default function App() {
return (
<div
className="App"
style={{ width: 500, height: 500, border: "2px solid red" }}
>
This is the parent component
<Child number={5}></Child>
<Child></Child>
</div>
);
}
function Child(props) {
return (
<div style={{ width: 200, height: 200, border: "2px solid yellow" }}>
This is the child component. Value from props: <b>{props.number}</b>
</div>
);
}
如果没有默认的 props,没有接收到 props
但在 JSX 中显示 props
值的子组件将不会显示任何内容。这个例子渲染了两个子组件,一个带有 props
,一个没有。
让我们看一下输出。
什么都不显示不是一个好的用户体验实践。你的用户可能认为你的 React 应用程序出现故障。
如果你的子组件在理想情况下没有收到任何 props,你应该显示一条消息来传达这一点。
在 React 中为功能组件设置默认 props
功能组件提供了一种简单的语法来呈现视觉元素。从 React 16.8 版开始,你甚至可以实现状态功能并在组件的不同生命周期上执行功能。
你可以使用 defaultProps
属性为功能组件设置默认 props。让我们来看看。
export default function App() {
return (
<div
className="App"
style={{ width: 500, height: 500, border: "2px solid red" }}
>
This is the parent component
<Child number={5}></Child>
<Child></Child>
</div>
);
}
function Child(props) {
return (
<div style={{ width: 200, height: 200, border: "2px solid yellow" }}>
This is the child component. Number from props: <b>{props.number}</b>
</div>
);
}
Child.defaultProps = {
number: "number not passed"
};
你可以在 CodeSandbox 上查看演示。
在这种情况下,没有收到任何 props 的子组件的第二个实例将显示字符串 "number not pass"
。它不会像没有 defaultProps
时那样为空。
当我们提供 props
时,子组件将显示我们传递的值。未通过 props
对象传递的值的默认 props 替代。
在 React 中为类组件设置默认 props
类组件的语法不同于功能组件。React 开发人员仍然必须使用 defaultProps
属性来设置默认 props,但实现方式不同。
这是一个在类组件上设置 defaultProps
属性的简单示例。
class Child extends React.Component {
constructor(props) {}
static defaultProps = {
number: "number not passed"
}
render() {
return <div>
<div style={{ width: 200, height: 200, border: "2px solid yellow" }}>
This is the child component. Number from props: <b>{this.props.number}</b>
</div>
</div>
}
}
此类组件将具有与上述功能组件相同的输出。
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn