在 Python 中將檔案讀取為字串
-
在 Python 中使用
read()
方法將文字檔案讀取為字串 -
在 Python 中使用
pathlib.read_text()
函式將文字檔案讀取為字串 -
在 Python 中使用
join()
函式將文字檔案讀取為字串
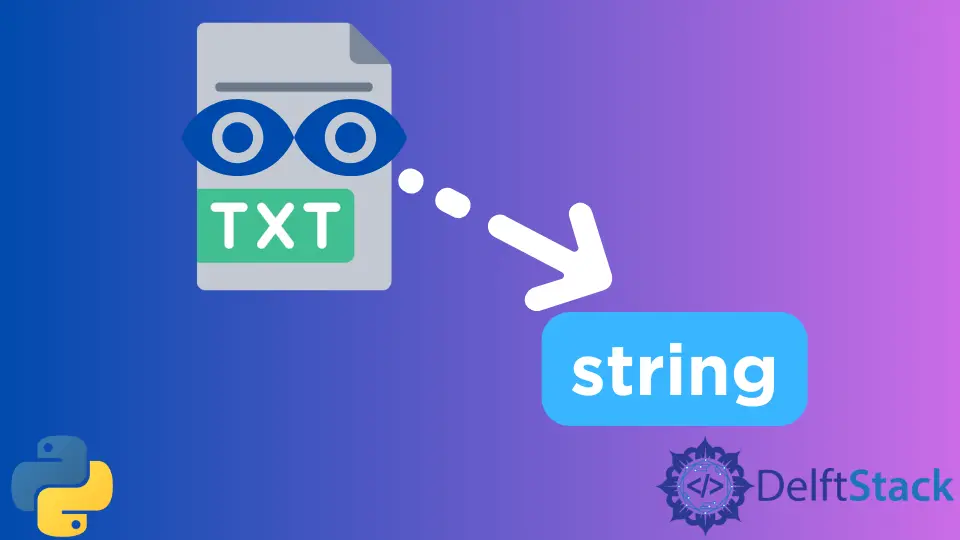
在 Python 中,我們有內建函式可以處理不同檔案型別的檔案操作。文字檔案包含一系列字串,其中每行以換行符\n
終止。
在本教程中,我們將學習如何在 Python 中將文字檔案讀取為字串。
在 Python 中使用 read()
方法將文字檔案讀取為字串
檔案物件的 read()
方法使我們可以一次從一個文字檔案中讀取所有內容。首先,我們將建立一個檔案物件,並使用 open()
函式以閱讀模式開啟所需的文字檔案。然後,我們將 read()
函式與此檔案物件一起使用,以將所有文字讀入字串並按如下所示進行列印。
with open("sample.txt") as f:
content = f.read()
print(content)
輸出:
sample line 1\n sample line 2\n sample line 3\n
當我們讀取檔案時,它也會讀取換行符\n
。我們可以使用 replace()
函式來去掉該字元。此函式將用該函式中的指定字元替換字串中的所有換行符。
例如,
with open("sample.txt") as f:
content = f.read().replace("\n", " ")
print(content)
輸出:
sample line 1 sample line 2 sample line 3
在 Python 中使用 pathlib.read_text()
函式將文字檔案讀取為字串
pathlib
模組已新增到 Python 3.4 中,並具有可用於檔案處理和系統路徑的更有效方法。該模組中的 read_text()
函式可以讀取文字檔案並在同一行中將其關閉。以下程式碼顯示了這一點。
from pathlib import Path
content = Path("sample.txt").read_text().replace("\n", " ")
print(content)
輸出:
sample line 1 sample line 2 sample line 3
在 Python 中使用 join()
函式將文字檔案讀取為字串
join()
方法允許我們在 Python 中連線不同的可迭代物件。我們也可以使用此方法將文字檔案讀取為字串。為此,我們將使用檔案物件讀取所有內容,然後使用列表推導方法,並使用 join()
函式將它們組合在一起。下面的程式碼實現了這一點。
with open("sample.txt") as f:
content = " ".join([l.rstrip() for l in f])
print(content)
輸出:
sample line 1 sample line 2 sample line 3
這裡的 rstrip()
函式從行中刪除所有尾隨字元。
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn相關文章 - Python File
- Python 如何得到資料夾下的所有檔案
- Python 如何查詢具有特定副檔名的檔案
- 如何在 Python 中從檔案讀取特定行
- 在 Python 中讀取文字檔案並列印其內容
- 在 Python 中逐行讀取 CSV
- 在 Python 中實現 touch 檔案