如何在 Pandas DataFrame 中新增一行
Asad Riaz
2023年1月30日
Pandas
Pandas DataFrame
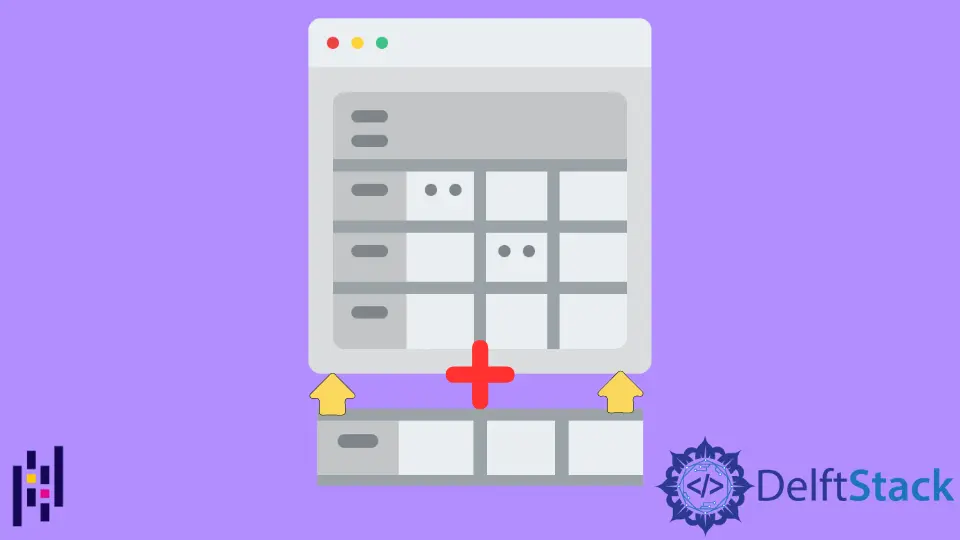
Pandas 旨在載入一個完全填充的 DataFrame
。我們可以在 pandas.DataFrame
中一一新增。這可以通過使用各種方法來完成,例如 .loc
,字典,pandas.concat()
或 DataFrame.append()
。
使用 .loc [index]
方法將行新增到帶有列表的 Pandas DataFrame 中
loc[index]
會將新列表作為新行,並將其新增到 pandas.dataframe
的索引 index
中。
考慮以下程式碼:
# python 3.x
import pandas as pd
# List of Tuples
fruit_list = [("Orange", 34, "Yes")]
# Create a DataFrame object
df = pd.DataFrame(fruit_list, columns=["Name", "Price", "Stock"])
# Add new ROW
df.loc[1] = ["Mango", 4, "No"]
df.loc[2] = ["Apple", 14, "Yes"]
print(df)
結果:
Name Price Stock
0 Orange 34 Yes
1 Mango 4 No
2 Apple 14 Yes
將字典作為行新增到 Pandas DataFrame
append()
可以直接將字典中的鍵值作為一行,將其新增到 pandas dataframe 中。
考慮以下程式碼:
# python 3.x
import pandas as pd
# List of Tuples
fruit_list = [("Orange", 34, "Yes")]
# Create a DataFrame object
df = pd.DataFrame(fruit_list, columns=["Name", "Price", "Stock"])
# Add new ROW
df = df.append({"Name": "Apple", "Price": 23, "Stock": "No"}, ignore_index=True)
df = df.append({"Name": "Mango", "Price": 13, "Stock": "Yes"}, ignore_index=True)
print(df)
結果:
Name Price Stock
0 Orange 34 Yes
1 Apple 23 No
2 Mango 13 Yes
Dataframe .append
方法新增一行
.append
可用於將其他 DataFrame
的行追加到原始 DataFrame
的末尾,並返回一個新的 DataFrame
。來自新 DataFrame
的列(不在原始 datafarme
中)也新增到現有的 DataFrame
中,新的單元格值填充為 NaN
。
考慮以下程式碼:
# python 3.x
import pandas as pd
# List of Tuples
fruit_list = [("Orange", 34, "Yes")]
# Create a DataFrame object
df = pd.DataFrame(fruit_list, columns=["Name", "Price", "Stock"])
print("Original DataFrame:")
print(df)
print(".............................")
print(".............................")
new_fruit_list = [("Apple", 34, "Yes", "small")]
dfNew = pd.DataFrame(new_fruit_list, columns=["Name", "Price", "Stock", "Type"])
print("Newly Created DataFrame:")
print(dfNew)
print(".............................")
print(".............................")
# append one dataframe to othher
df = df.append(dfNew, ignore_index=True)
print("Copying DataFrame to orignal...")
print(df)
ignore_index = True
將忽略新 DataFrame
的 index
並從原始 DataFrame
為其分配新索引。
輸出:
Original DataFrame:
Name Price Stock
0 Orange 34 Yes
.............................
.............................
Newly Created DataFrame:
Name Price Stock Type
0 Apple 34 Yes small
.............................
.............................
Copying DataFrame to original..:
Name Price Stock Type
0 Orange 34 Yes NaN
1 Apple 34 Yes small
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe