使用 PHP 和 MySQL 設定搜尋系統
Habdul Hazeez
2023年1月30日
PHP
PHP MySQL
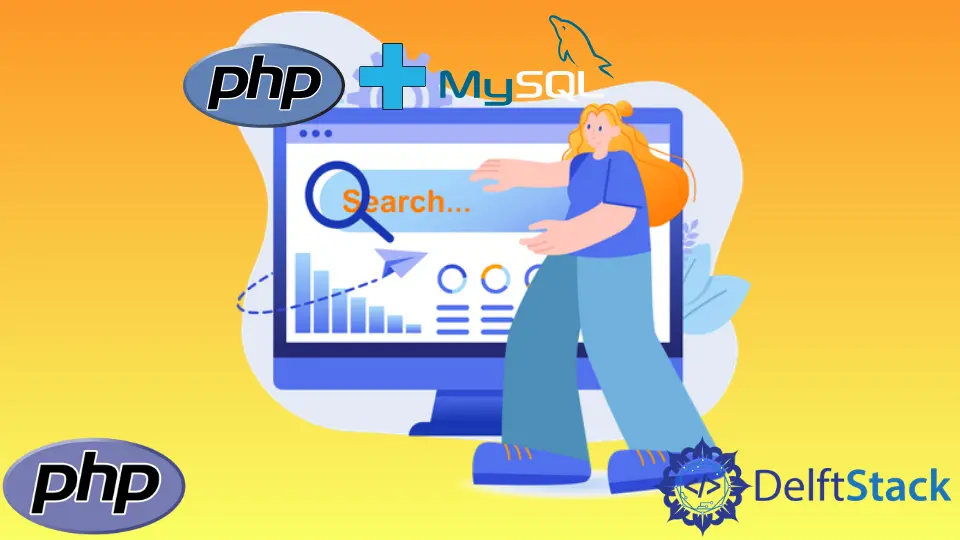
本教程將教你使用 PHP 和 MySQL 建立一個搜尋系統。你將學習如何設定 HTML、MySQL 資料庫和 PHP 後端。在 PHP 程式碼中,你將學習在 SQL 中使用帶有 LIKE 運算子的準備好的語句。
設定資料庫
下載並安裝 XAMPP 伺服器。它與 MySQL 一起提供。在 XAMPP 控制面板中啟動 shell。使用以下命令登入 MySQL shell:
# This login command assumes that the
# password is empty and the user is "root"
mysql -u root -p
使用以下 SQL 查詢建立一個名為 fruit_db
的資料庫。
CREATE database fruit_db;
輸出:
Query OK, 1 row affected (0.001 sec)
你將需要可以使用的示例資料。因此,在 fruit_db
資料庫上執行以下 SQL:
CREATE TABLE fruit
(id INT NOT NULL AUTO_INCREMENT,
name VARCHAR(20) NOT NULL,
color VARCHAR(20) NOT NULL,
PRIMARY KEY (id))
ENGINE = InnoDB;
輸出:
Query OK, 0 rows affected (0.028 sec)
檢查表的結構:
DESC fruit;
輸出:
+-------+-------------+------+-----+---------+----------------+
| Field | Type | Null | Key | Default | Extra |
+-------+-------------+------+-----+---------+----------------+
| id | int(11) | NO | PRI | NULL | auto_increment |
| name | varchar(20) | NO | | NULL | |
| color | varchar(20) | NO | | NULL | |
+-------+-------------+------+-----+---------+----------------+
建立表後,使用以下 SQL 插入示例資料:
INSERT INTO fruit (id, name, color) VALUES (NULL, 'Banana', 'Yellow'), (NULL, 'Pineapple', 'Green')
輸出:
Query OK, 2 rows affected (0.330 sec)
Records: 2 Duplicates: 0 Warnings: 0
使用以下 SQL 確認資料存在:
SELECT * FROM fruit;
輸出:
+----+-----------+--------+
| id | name | color |
+----+-----------+--------+
| 1 | Banana | Yellow |
| 2 | Pineapple | Green |
+----+-----------+--------+
建立 HTML 程式碼
搜尋系統的 HTML 程式碼是一個 HTML 表單。該表單有一個表單輸入和一個 submit
按鈕。表單輸入中的 required
屬性確保使用者在表單中輸入內容。
下一個程式碼塊是搜尋表單的 HTML 程式碼。
<main>
<form action="searchdb.php" method="post">
<input
type="text"
placeholder="Enter your search term"
name="search"
required>
<button type="submit" name="submit">Search</button>
</form>
</main>
下面的 CSS 使表單更美觀。
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
display: grid;
align-items: center;
place-items: center;
height: 100vh;
}
main {
width: 60%;
border: 2px solid #1560bd;
padding: 2em;
display: flex;
justify-content: center;
}
input,
button {
padding: 0.2em;
}
HTML 應該類似於你的網路瀏覽器中的下一個影象。
建立 PHP 程式碼
PHP 程式碼將處理表單提交。以下是程式碼工作原理的摘要:
- 檢查使用者提交的表單。
- 連線資料庫。
- 轉義搜尋字串並去除所有的空白。
- 檢查無效字元,如
<
或-
(不帶引號)。 - 通過準備好的語句執行搜尋。
- 返回結果。
下一個程式碼塊是執行搜尋的完整 PHP 程式碼。將程式碼儲存在名為 searchdb.php
的檔案中。
<?php
if (isset($_POST['submit'])) {
// Connect to the database
$connection_string = new mysqli("localhost", "root", "", "fruit_db");
// Escape the search string and trim
// all whitespace
$searchString = mysqli_real_escape_string($connection_string, trim(htmlentities($_POST['search'])));
// If there is a connection error, notify
// the user, and Kill the script.
if ($connection_string->connect_error) {
echo "Failed to connect to Database";
exit();
}
// Check for empty strings and non-alphanumeric
// characters.
// Also, check if the string length is less than
// three. If any of the checks returns "true",
// return "Invalid search string", and
// kill the script.
if ($searchString === "" || !ctype_alnum($searchString) || $searchString < 3) {
echo "Invalid search string";
exit();
}
// We are using a prepared statement with the
// search functionality to prevent SQL injection.
// So, we need to prepend and append the search
// string with percent signs
$searchString = "%$searchString%";
// The prepared statement
$sql = "SELECT * FROM fruit WHERE name LIKE ?";
// Prepare, bind, and execute the query
$prepared_stmt = $connection_string->prepare($sql);
$prepared_stmt->bind_param('s', $searchString);
$prepared_stmt->execute();
// Fetch the result
$result = $prepared_stmt->get_result();
if ($result->num_rows === 0) {
// No match found
echo "No match found";
// Kill the script
exit();
} else {
// Process the result(s)
while ($row = $result->fetch_assoc()) {
echo "<b>Fruit Name</b>: ". $row['name'] . "<br />";
echo "<b>Fruit Color</b>: ". $row['color'] . "<br />";
} // end of while loop
} // end of if($result->num_rows)
} else { // The user accessed the script directly
// Tell them nicely and kill the script.
echo "That is not allowed!";
exit();
}
?>
下一張圖片包含搜尋字串 pine
的結果。它返回水果 pine
及其顏色。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Habdul Hazeez
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn