Configurar un sistema de búsqueda con PHP y MySQL
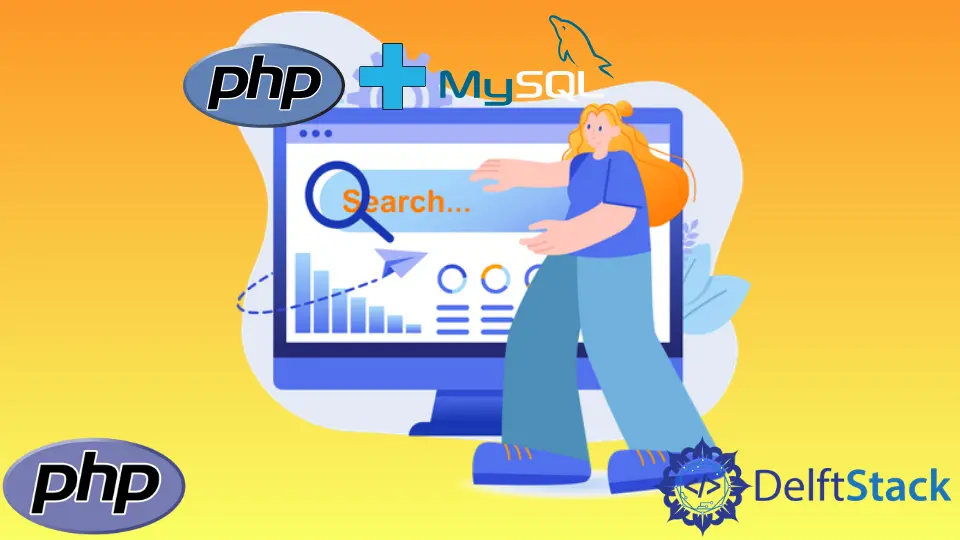
Este tutorial le enseñará a crear un sistema de búsqueda con PHP y MySQL. Aprenderá a configurar HTML, la base de datos MySQL y el backend de PHP. En el código PHP, aprenderá a usar una declaración preparada con el operador LIKE en SQL.
Configurar una base de datos
Descargar e instalar el servidor XAMPP. Viene con MySQL. Inicie el shell en el panel de control de XAMPP. Inicie sesión en el shell de MySQL con el siguiente comando:
# This login command assumes that the
# password is empty and the user is "root"
mysql -u root -p
Use la siguiente consulta SQL para crear una base de datos llamada fruit_db
.
CREATE database fruit_db;
Producción :
Query OK, 1 row affected (0.001 sec)
Necesitará datos de muestra que pueda usar. Entonces, ejecute el siguiente SQL en la base de datos fruit_db
:
CREATE TABLE fruit
(id INT NOT NULL AUTO_INCREMENT,
name VARCHAR(20) NOT NULL,
color VARCHAR(20) NOT NULL,
PRIMARY KEY (id))
ENGINE = InnoDB;
Producción :
Query OK, 0 rows affected (0.028 sec)
Compruebe la estructura de la tabla:
DESC fruit;
Producción :
+-------+-------------+------+-----+---------+----------------+
| Field | Type | Null | Key | Default | Extra |
+-------+-------------+------+-----+---------+----------------+
| id | int(11) | NO | PRI | NULL | auto_increment |
| name | varchar(20) | NO | | NULL | |
| color | varchar(20) | NO | | NULL | |
+-------+-------------+------+-----+---------+----------------+
Una vez que la tabla esté configurada, use el siguiente SQL para insertar datos de muestra:
INSERT INTO fruit (id, name, color) VALUES (NULL, 'Banana', 'Yellow'), (NULL, 'Pineapple', 'Green')
Producción :
Query OK, 2 rows affected (0.330 sec)
Records: 2 Duplicates: 0 Warnings: 0
Confirme la existencia de datos con el siguiente SQL:
SELECT * FROM fruit;
Producción :
+----+-----------+--------+
| id | name | color |
+----+-----------+--------+
| 1 | Banana | Yellow |
| 2 | Pineapple | Green |
+----+-----------+--------+
Crear el código HTML
El código HTML para el sistema de búsqueda es un formulario HTML. El formulario tiene una sola entrada y un botón submit
. Un atributo required
en la entrada del formulario asegura que los usuarios introduzcan algo en el formulario.
El siguiente bloque de código es el código HTML para el formulario de búsqueda.
<main>
<form action="searchdb.php" method="post">
<input
type="text"
placeholder="Enter your search term"
name="search"
required>
<button type="submit" name="submit">Search</button>
</form>
</main>
El siguiente CSS hace que el formulario sea más presentable.
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
display: grid;
align-items: center;
place-items: center;
height: 100vh;
}
main {
width: 60%;
border: 2px solid #1560bd;
padding: 2em;
display: flex;
justify-content: center;
}
input,
button {
padding: 0.2em;
}
El HTML debe ser como la siguiente imagen en su navegador web.
Crear el código PHP
El código PHP procesará el envío del formulario. Aquí hay un resumen de cómo funciona el código:
- Verifique el formulario enviado por el usuario.
- Conéctese a la base de datos.
- Escapa de la cadena de búsqueda y recorta los espacios en blanco.
- Compruebe si hay caracteres no válidos como
<
o-
(sin comillas). - Realice la búsqueda a través de una declaración preparada.
- Devuelva los resultados.
El siguiente bloque de código es el código PHP completo para realizar la búsqueda. Guarda el código en un archivo llamado searchdb.php
.
<?php
if (isset($_POST['submit'])) {
// Connect to the database
$connection_string = new mysqli("localhost", "root", "", "fruit_db");
// Escape the search string and trim
// all whitespace
$searchString = mysqli_real_escape_string($connection_string, trim(htmlentities($_POST['search'])));
// If there is a connection error, notify
// the user, and Kill the script.
if ($connection_string->connect_error) {
echo "Failed to connect to Database";
exit();
}
// Check for empty strings and non-alphanumeric
// characters.
// Also, check if the string length is less than
// three. If any of the checks returns "true",
// return "Invalid search string", and
// kill the script.
if ($searchString === "" || !ctype_alnum($searchString) || $searchString < 3) {
echo "Invalid search string";
exit();
}
// We are using a prepared statement with the
// search functionality to prevent SQL injection.
// So, we need to prepend and append the search
// string with percent signs
$searchString = "%$searchString%";
// The prepared statement
$sql = "SELECT * FROM fruit WHERE name LIKE ?";
// Prepare, bind, and execute the query
$prepared_stmt = $connection_string->prepare($sql);
$prepared_stmt->bind_param('s', $searchString);
$prepared_stmt->execute();
// Fetch the result
$result = $prepared_stmt->get_result();
if ($result->num_rows === 0) {
// No match found
echo "No match found";
// Kill the script
exit();
} else {
// Process the result(s)
while ($row = $result->fetch_assoc()) {
echo "<b>Fruit Name</b>: ". $row['name'] . "<br />";
echo "<b>Fruit Color</b>: ". $row['color'] . "<br />";
} // end of while loop
} // end of if($result->num_rows)
} else { // The user accessed the script directly
// Tell them nicely and kill the script.
echo "That is not allowed!";
exit();
}
?>
La siguiente imagen contiene el resultado de la cadena de búsqueda pine
. Devuelve la fruta pineapple
y su color.
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn