在 PHP 中執行多個 MySQL 查詢
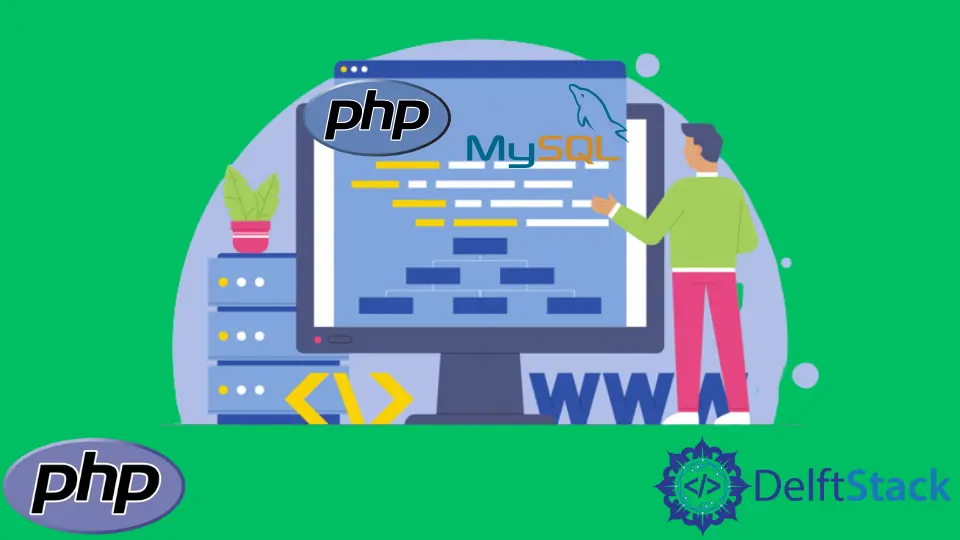
本教程將教你在 PHP 中執行多個 SQL 查詢。我們將討論兩種方法來展示如何做到這一點。
第一種方法使用 PHP prepared statements,而第二種方法將使用 PHP multi_query
函式。
在 PHP 中使用準備好的語句執行多個 SQL 查詢
為了演示如何使用準備好的語句執行多個 SQL 查詢,我們需要三樣東西:HTML 表單、MySQL 資料庫和執行查詢的 PHP 程式碼。讓我們從資料庫開始。
使用 MySQL 建立資料庫和資料庫表
要建立資料庫,我們將使用帶有 XAMPP 的本地 MySQL 資料庫。從他們的官方網站下載並安裝 XAMPP。
下載完成後,啟動 XAMPP 控制面板。然後使用以下命令啟動 MySQL shell。
# This login command assumes that the
# password is empty, and the user is "root"
mysql -u root -p
建立一個名為 fruit_database
的資料庫並使用它。
CREATE database fruit_database;
USE fruit_database;
輸出:
Query OK, 1 row affected (0.002 sec)
Database changed
在資料庫中建立一個名為 fruits
的表。
CREATE TABLE fruits
(id INT NOT NULL AUTO_INCREMENT,
name VARCHAR(20) NOT NULL,
PRIMARY KEY (id))
ENGINE = InnoDB;
輸出:
Query OK, 0 rows affected (0.028 sec)
建立 HTML 表單
我們將在 HTML 表單中使用兩個表單輸入和一個提交按鈕。表單輸入將採用我們將插入資料庫的兩個字串。
這是表單的 HTML。
<main>
<form action="multi-sql.php" method="post">
<div class="form-row">
<label
for="first-color">
First Color
</label>
<input
type="text"
placeholder="Enter the first color"
name="first-color"
id="first-color"
required
>
</div>
<div class="form-row">
<label
for="second-color">
Second Color
</label>
<input
type="text"
placeholder="Enter the second color"
name="second-color"
id="second-color"
required
>
</div>
<div class="form-row flex-center">
<button
type="submit"
name="submit"
>
Submit Colors
</button>
</div>
</form>
</main>
建立 PHP 程式碼
PHP 程式碼將處理表單輸入,然後將其值提交到資料庫。
我們清理使用者提交的字串。然後我們將這些字串用作 SQL 查詢的一部分。
之後,我們將查詢儲存在一個陣列中。為了提交它們,我們使用準備好的語句。
將以下 PHP 程式碼儲存為 multi-sql.php
。
<?php
if (isset($_POST['submit'])) {
// Connect to the database
$connection_string = new mysqli("localhost", "root", "", "fruit_database");
// Sanitise the input strings
$firstColor = mysqli_real_escape_string($connection_string, trim(htmlentities($_POST['first-color'])));
$secondColor = mysqli_real_escape_string($connection_string, trim(htmlentities($_POST['second-color'])));
// If there is a connection error, notify
// the user, and Kill the script.
if ($connection_string->connect_error) {
echo "Failed to connect to Database";
exit();
}
// Check string length, empty strings and
// non-alphanumeric characters.
if ( $firstColor === "" || !ctype_alnum($firstColor) ||
strlen($firstColor) <= 3
) {
echo "The first color value is invalid.";
exit();
}
if ( $secondColor === "" || !ctype_alnum($secondColor) ||
strlen($secondColor) <= 3
) {
echo "The second color value is invalid.";
exit();
}
$queries = [
"INSERT into fruits (name) VALUES ('$firstColor')",
"INSERT into fruits (name) VALUES ('$secondColor')"
];
// Execute the multiple SQL queries
foreach ($queries as $query) {
$stmt = $connection_string->prepare($query);
$stmt->execute();
}
if ($stmt->affected_rows === 1) {
echo "Data inserted successfully";
}
} else { // The user accessed the script directly
// Kill the script.
echo "That is not allowed!";
exit();
}
?>
輸出(如果成功):
Data inserted successfully
輸出(如果輸入有錯誤):
The first color value is invalid.
在 PHP 中使用 Multi_query
執行多個 SQL 查詢
你可以使用 PHP 中的內建函式 multi_query
執行多個 SQL 查詢。SQL 查詢應該在一個帶引號的字串中,以便使用 multi_query
進行多個查詢,但每個 SQL 應該用分號分隔。
對於 HTML 和 CSS,你可以使用與上一節相同的 HTML 和 CSS 程式碼。
下一個 PHP 程式碼將使用 multi_query
將資料插入資料庫。將其儲存為 multi-sql-v2.php
。
相應地更新你的 HTML。
<?php
if (isset($_POST['submit'])) {
// Connect to the database
$connection_string = new mysqli("localhost", "root", "", "fruit_database");
// Sanitise the input strings
$firstColor = mysqli_real_escape_string($connection_string, trim(htmlentities($_POST['first-color'])));
$secondColor = mysqli_real_escape_string($connection_string, trim(htmlentities($_POST['second-color'])));
// If there is a connection error, notify
// the user, and Kill the script.
if ($connection_string->connect_error) {
echo "Failed to connect to Database";
exit();
}
// Check string length, empty strings
// and non-alphanumeric characters.
if ($firstColor === "" || !ctype_alnum($firstColor) ||
strlen($firstColor) <= 3
) {
echo "The first color value is invalid.";
exit();
}
if ( $secondColor === "" || !ctype_alnum($secondColor) ||
strlen($secondColor) <= 3
) {
echo "The second color value is invalid.";
exit();
}
// Prepare the SQL queries for MySQL
// multi queries
$sql = "INSERT into fruits (name) VALUES ('$firstColor');
INSERT into fruits (name) VALUES ('$secondColor')";
// Execute the queries with multi_query
if ($connection_string->multi_query($sql) === TRUE) {
echo "Data inserted successfully";
}
} else { // The user accessed the script directly
// Kill the script.
echo "That is not allowed!";
exit();
}
?>
輸出(如果成功):
Data inserted successfully
輸出(如果輸入有錯誤):
The first color value is invalid.
建立 CSS 程式碼來設定 HTML 表單的樣式
下面的 CSS 程式碼將為本文中建立的 HTML 表單設定樣式。
* {
box-sizing: border-box;
padding: 0;
margin: 0;
}
body {
display: grid;
align-items: center;
place-items: center;
height: 100vh;
}
main {
display: flex;
justify-content: center;
width: 60%;
border: 5px solid #1a1a1a;
padding: 1em;
}
form {
font-size: 1.2em;
width: 100%;
}
input,
label {
width: 50%;
font-size: 1em;
padding: 0.2em;
}
button {
padding: 0.2em;
font-size: 1em;
}
.form-row {
display: flex;
justify-content: space-between;
margin-bottom: 0.5em;
padding: 0.2em;
}
.flex-center {
display: flex;
justify-content: center;
}
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn