PHP 私有函式
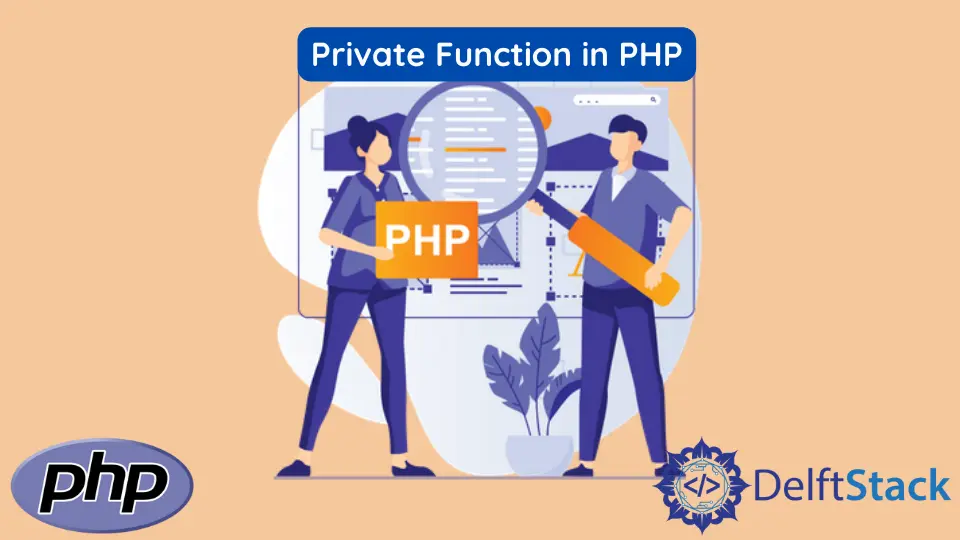
如果某些屬性或方法在 PHP 中被宣告為 private
可見性關鍵字,則只能在其類中訪問它。
Private
函式只能在定義它的類中訪問,不能在類外訪問。
使用 private
函式的一個主要缺點是子類不能繼承這些函式。private
功能應正確使用。
本教程演示了 private
函式的用法。
示範 private
函式的使用
首先,我們將建立一個具有一個 private
和一個預設 public
方法的類。我們將呼叫 private
函式,然後退出類。
班上:
<?php
class test_private
{
// Public constructor
public function __construct() { }
// Declaring a private method
private function TestPrivate() {
echo "Hello! this is private function declared in test_private class";
}
// Default is always public
function test()
{
$this->TestPrivate();
}
}
?>
在類外呼叫函式 TestPrivate()
:
<?php
$testclass = new test_private;
$testclass->TestPrivate(); // This will cause fatal error.
?>
輸出:
PHP Fatal error: Uncaught Error: Call to private method test_private::TestPrivate() from context '' in /home/znhT6B/prog.php:22 Stack trace: #0 {main} thrown in /home/znhT6B/prog.php on line 22
在類外呼叫函式 test()
:
$testclass = new test_private;
$testclass->test(); // this will run the private function because it is called inside the class
輸出:
Hello! this is private function declared in test_private class
正如我們所看到的,當 private
函式僅在其類內部呼叫時才有效,在我們的程式碼中,我們建立了一個 public
函式測試來呼叫 private
函式,以便我們可以在類外部呼叫它。
演示在繼承中使用 private
函式
不可能呼叫在子類中宣告的 private
方法。私有方法只能在同一個類中呼叫。
參見示例:
<?php
class test_private2 extends test_private
{
// This is default public
function test2()
{
$this->TestPrivate();
}
}
$testclass2 = new test_private2;
$testclass2->test2(); // this will generate the same error.
?>
Class test_private2
是第一個示例程式碼中給出的 test_private
類的子類。我們將父
類稱為子類中的 private
函式。
輸出:
Fatal error: Uncaught Error: Call to private method test_private::TestPrivate() from context 'test_private2' in C:\Apache24\htdocs\php private.php:25 Stack trace: #0 C:\Apache24\htdocs\php private.php(30): test_private2->test2() #1 {main} thrown in C:\Apache24\htdocs\php private.php on line 25
如果我們通過在子類中建立一個具有相同名稱的新函式來覆蓋 private
函式會怎樣?答案是一旦宣告瞭 private
函式,就不能在子類中覆蓋它。
<?php
class Cars
{
public function test() {
$this->test_public();
$this->test_private();
}
public function test_public() {
echo "This is public function from Cars class.";
echo "<br>";
}
private function test_private() {
echo "This is private function from Cars class.";
echo "<br>";
}
}
class Bikes extends Cars
{
public function test_public() {
echo "This is public function from Bikes class.";
echo "<br>";
}
private function test_private() {
echo "This is private function from Bikes class.";
echo "<br>";
}
}
$mybike = new Bikes();
$mybike->test();
?>
上面的程式碼試圖覆蓋子類中的 private
和 public
函式,但只有 public
函式將被覆蓋。
輸出:
This is public function from Bikes class.
This is private function from Cars class.
正如我們從輸出中看到的,私有函式沒有被子類覆蓋。
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook