PHP 私有函数
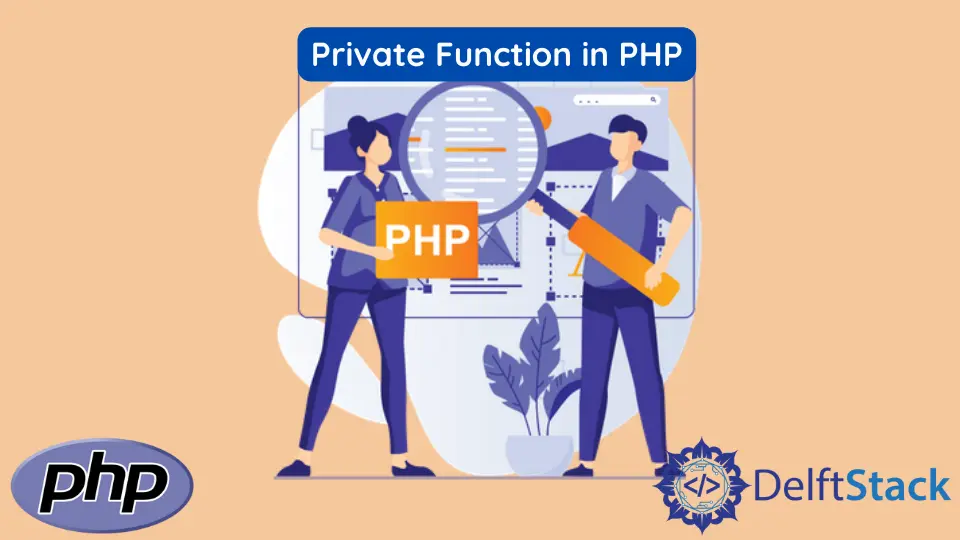
如果某些属性或方法在 PHP 中被声明为 private
可见性关键字,则只能在其类中访问它。
Private
函数只能在定义它的类中访问,不能在类外访问。
使用 private
函数的一个主要缺点是子类不能继承这些函数。private
功能应正确使用。
本教程演示了 private
函数的用法。
示范 private
函数的使用
首先,我们将创建一个具有一个 private
和一个默认 public
方法的类。我们将调用 private
函数,然后退出类。
班上:
<?php
class test_private
{
// Public constructor
public function __construct() { }
// Declaring a private method
private function TestPrivate() {
echo "Hello! this is private function declared in test_private class";
}
// Default is always public
function test()
{
$this->TestPrivate();
}
}
?>
在类外调用函数 TestPrivate()
:
<?php
$testclass = new test_private;
$testclass->TestPrivate(); // This will cause fatal error.
?>
输出:
PHP Fatal error: Uncaught Error: Call to private method test_private::TestPrivate() from context '' in /home/znhT6B/prog.php:22 Stack trace: #0 {main} thrown in /home/znhT6B/prog.php on line 22
在类外调用函数 test()
:
$testclass = new test_private;
$testclass->test(); // this will run the private function because it is called inside the class
输出:
Hello! this is private function declared in test_private class
正如我们所看到的,当 private
函数仅在其类内部调用时才有效,在我们的代码中,我们创建了一个 public
函数测试来调用 private
函数,以便我们可以在类外部调用它。
演示在继承中使用 private
函数
不可能调用在子类中声明的 private
方法。私有方法只能在同一个类中调用。
参见示例:
<?php
class test_private2 extends test_private
{
// This is default public
function test2()
{
$this->TestPrivate();
}
}
$testclass2 = new test_private2;
$testclass2->test2(); // this will generate the same error.
?>
Class test_private2
是第一个示例代码中给出的 test_private
类的子类。我们将父
类称为子类中的 private
函数。
输出:
Fatal error: Uncaught Error: Call to private method test_private::TestPrivate() from context 'test_private2' in C:\Apache24\htdocs\php private.php:25 Stack trace: #0 C:\Apache24\htdocs\php private.php(30): test_private2->test2() #1 {main} thrown in C:\Apache24\htdocs\php private.php on line 25
如果我们通过在子类中创建一个具有相同名称的新函数来覆盖 private
函数会怎样?答案是一旦声明了 private
函数,就不能在子类中覆盖它。
<?php
class Cars
{
public function test() {
$this->test_public();
$this->test_private();
}
public function test_public() {
echo "This is public function from Cars class.";
echo "<br>";
}
private function test_private() {
echo "This is private function from Cars class.";
echo "<br>";
}
}
class Bikes extends Cars
{
public function test_public() {
echo "This is public function from Bikes class.";
echo "<br>";
}
private function test_private() {
echo "This is private function from Bikes class.";
echo "<br>";
}
}
$mybike = new Bikes();
$mybike->test();
?>
上面的代码试图覆盖子类中的 private
和 public
函数,但只有 public
函数将被覆盖。
输出:
This is public function from Bikes class.
This is private function from Cars class.
正如我们从输出中看到的,私有函数没有被子类覆盖。
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook