使用 jQuery 在懸停時顯示工具提示訊息
- jQuery-UI 工具提示
-
使用帶有
options
引數的tooltip()
方法使用 jQuery 在懸停時顯示工具提示訊息 -
使用帶有
actions
引數的tooltip()
方法使用 jQuery 在懸停時顯示工具提示訊息
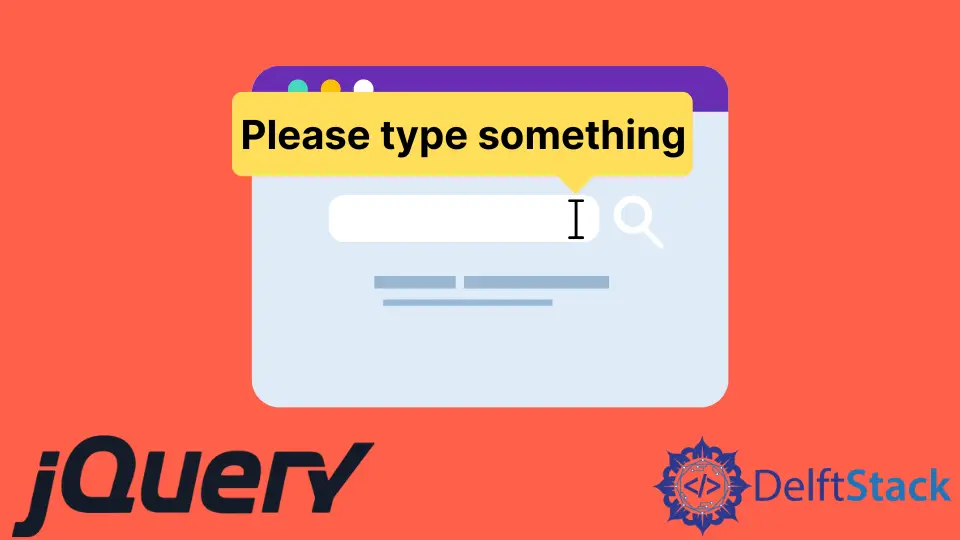
Tooltip 是一個來自 jQuery-UI 的小部件,用於新增新主題並允許自定義。本教程演示瞭如何使用 jQuery-UI 工具提示。
jQuery-UI 工具提示
來自 jQuery 的 Tooltip 用於允許自定義和建立新主題。工具提示附加到任何元素;為了顯示它們,我們為它們新增了一個標題屬性,它將用作工具提示。
當我們將滑鼠懸停在該元素上時,帶有 title
屬性的工具提示將可見。jQuery-UI 提供了一個方法 tooltip()
,它可以為任何元素新增工具提示。
tooltip()
方法在顯示和隱藏工具提示時預設提供淡入淡出動畫。
此方法的語法是:
Method 1:
$(selector, context).tooltip (options)
Method 2:
$(selector, context).tooltip ("action", [params])
有兩種方法可以使用 tooltip()
方法。
使用帶有 options
引數的 tooltip()
方法使用 jQuery 在懸停時顯示工具提示訊息
第一個帶有 options
引數的方法用於指定工具提示的行為和外觀。引數 options
可以多次插入並且有多種型別。
下表顯示了 options
的型別:
選項 | 描述 |
---|---|
content |
此選項使用函式的預設值表示工具提示的內容,該值返回 title 屬性。 |
disabled |
此選項用於禁用具有 true 或 false 值的工具提示。 |
hide |
此選項表示隱藏工具提示時的動畫。值為真或假。 |
show |
此選項表示工具提示在顯示時的動畫。值為真或假。 |
track |
此選項在使用工具提示時跟蹤滑鼠。預設值為假。 |
items |
此選項用於選擇哪個專案將顯示工具提示。預設值為標題 |
position |
此選項用於決定工具提示的位置。預設值是返回標題屬性的函式。 |
tooltip class |
此選項表示可以新增到工具提示的類。它可以表示警告或錯誤;此選項的預設值為 null。 |
首先,讓我們嘗試一個沒有引數的簡單 tooltip()
示例。確保匯入 jQuery-UI,以便 tooltip()
方法可以工作。
參見示例:
<!doctype html>
<html lang = "en">
<head>
<meta charset = "utf-8">
<title>jQuery Tooltip</title>
<script src = "https://code.jquery.com/jquery-1.10.2.js"></script>
<script src = "https://code.jquery.com/ui/1.10.4/jquery-ui.js"></script>
<link href = "https://code.jquery.com/ui/1.10.4/themes/ui-lightness/jquery-ui.css" rel = "stylesheet">
<script>
$(function() {
$("#Demotooltip1").tooltip();
$("#Demotooltip2").tooltip();
});
</script>
</head>
<body>
Type Something: <input id = "Demotooltip1" title = "Please Type Something">
<p><label id = "Demotooltip2" title = "This is tooltip Demo"> Hover Here to See Tooltip </label></p>
</body>
</html>
上面的程式碼將在文字輸入和標籤上顯示工具提示。見輸出:
現在,讓我們嘗試一個帶有 content
、disabled
和 track
選項的示例:
<!doctype html>
<html lang = "en">
<head>
<meta charset = "utf-8">
<title>jQuery Tooltip </title>
<link href = "https://code.jquery.com/ui/1.10.4/themes/ui-lightness/jquery-ui.css" rel = "stylesheet">
<script src = "https://code.jquery.com/jquery-1.10.2.js"></script>
<script src = "https://code.jquery.com/ui/1.10.4/jquery-ui.js"></script>
<script>
$(function() {
$( "#Demotooltip1" ).tooltip({
content: "Tooltip from Content",
track:true
}),
$( "#Demotooltip2" ).tooltip({
disabled: true
});
});
</script>
</head>
<body>
Type Something: <input id = "Demotooltip1" title = "Original">
<br><br>
Disabled Tooltip: <input id = "Demotooltip2" title = "Demotooltip">
</body>
</html>
上面的程式碼演示瞭如何使用 content
、track
和 disabled
選項。見輸出:
讓我們再嘗試一個帶有選項 position
的示例。參見示例:
<!doctype html>
<html lang = "en">
<head>
<meta charset = "utf-8">
<title>jQuery Tooltip</title>
<link href = "https://code.jquery.com/ui/1.10.4/themes/ui-lightness/jquery-ui.css" rel = "stylesheet">
<script src = "https://code.jquery.com/jquery-1.10.2.js"></script>
<script src = "https://code.jquery.com/ui/1.10.4/jquery-ui.js"></script>
<style>
body {
margin-top: 50px;
}
.ui-tooltip-content::after, .ui-tooltip-content::before {
position: absolute;
content: "";
display: block;
left: 100px;
border-style: solid;
}
.ui-tooltip-content::before {
bottom: -5px;
border-width: 5px 5px 0;
border-color: #AAA transparent;
}
.ui-tooltip-content::after {
bottom: -7px;
border-width: 10px 10px 0;
border-color: white transparent;
}
</style>
<script>
$(function() {
$( "#Demotooltip" ).tooltip({
position: {
my: "center bottom",
at: "center top-10",
collision: "none"
}
});
});
</script>
</head>
<body>
Type Something: <input id = "Demotooltip" title = "Please Type Something.">
</body>
</html>
上面的程式碼顯示了 position
選項的演示,它為工具提示新增了一個提示。見輸出:
類似地,其他選項可用於上表中描述的目的。現在,讓我們看看方法 2。
使用帶有 actions
引數的 tooltip()
方法使用 jQuery 在懸停時顯示工具提示訊息
$ (selector, context).tooltip ("action", [params])
方法將對元素執行操作,例如禁用工具提示。action
表示第一個引數中的字串,params
將根據給定的操作提供。
action
有多種型別,如下表所示:
行動 | 描述 |
---|---|
close |
此操作用於關閉工具提示。它沒有論據。 |
destroy |
此操作將完全刪除工具提示。它沒有論據。 |
disable |
此操作將停用工具提示。它沒有論據。 |
enable |
此操作將啟用工具提示。它沒有論據。 |
open |
此操作將以程式設計方式開啟工具提示。沒有爭論。 |
option() |
此操作將獲取表示當前工具提示選項雜湊的鍵值對。 |
option(Options) |
此操作用於設定工具提示的選項。 |
option(OptionName) |
這個動作是為了獲取 OptionName 的值。沒有其他論據。 |
option(OptionName, value) |
此操作將根據給定值設定 OptionName 的值。 |
widget |
此操作將返回一個包含原始元素的 jQuery 物件。沒有引數。 |
讓我們為 open
和 close
操作嘗試一個簡單的示例,這將通過按下按鈕保持工具提示開啟並在按下另一個按鈕時將其關閉。參見示例:
<!doctype html>
<html lang = "en">
<head>
<meta charset = "utf-8">
<title>jQuery Tooltip</title>
<link href = "https://code.jquery.com/ui/1.10.4/themes/ui-lightness/jquery-ui.css"
rel = "stylesheet">
<script src = "https://code.jquery.com/jquery-1.10.2.js"></script>
<script src = "https://code.jquery.com/ui/1.10.4/jquery-ui.js"></script>
<script>
$(function() {
$("#Demotooltip").tooltip({
//the 'of' keyword will be used to link the tooltip to the specified input
position: { of: '#DemoInput', my: 'left center', at: 'left center' },
}),
$('#DemoButton1').click(function () {
$('#Demotooltip').tooltip("open");
});
$('#DemoButton2').click(function () {
$('#Demotooltip').tooltip("close");
});
});
</script>
</head>
<body >
<label id = "Demotooltip" title = "Open and Close"></label>
<input id = "DemoInput" type = "text" size = "20" />
<input id = "DemoButton1" type = "submit" value = "Click to Show" />
<input id = "DemoButton2" type = "submit" value = "Click to Hide" />
</body>
</html>
上面的程式碼將在按鈕單擊時開啟和關閉工具提示。見輸出:
現在,讓我們嘗試一個 widget
動作的示例。widget
方法用於獲取 jQuery-UI 的例項。
參見示例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<link href= "https://code.jquery.com/ui/1.10.4/themes/ui-lightness/jquery-ui.css" rel="stylesheet"/>
<script src= "https://code.jquery.com/jquery-1.10.2.js"> </script>
<script src= "https://code.jquery.com/ui/1.10.4/jquery-ui.js"> </script>
<script>
$(function () {
$("#Demo2").tooltip({
track: true,
});
$("#Demo1").click(function () {
var a = $("#Demo2").tooltip("widget");
console.log(a);
});
});
</script>
</head>
<body>
<h1 style="color: lightblue">DELFTSTACK</h1>
<input id="Demo1" type="submit" name="delftstack" value="click" />
<span id="Demo2" title="delftstack"> Click Me</span>
</body>
</html>
上面的程式碼將使用 widget
方法來獲取 jQuery-UI 的例項。見輸出:
類似地,其他動作也可以用於表中描述的目的。
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook