在 C++ 中把浮點數取整到兩位小數
-
在 C++ 中使用
printf
函式格式說明符將浮點數四捨五入為 2 個小數 -
在 C++ 中使用
fprintf
函式格式說明符將浮點數舍入到 2 位小數 -
在 C++ 中使用
std::setprecision
和std::fixed
將浮點數舍入為 2 個小數
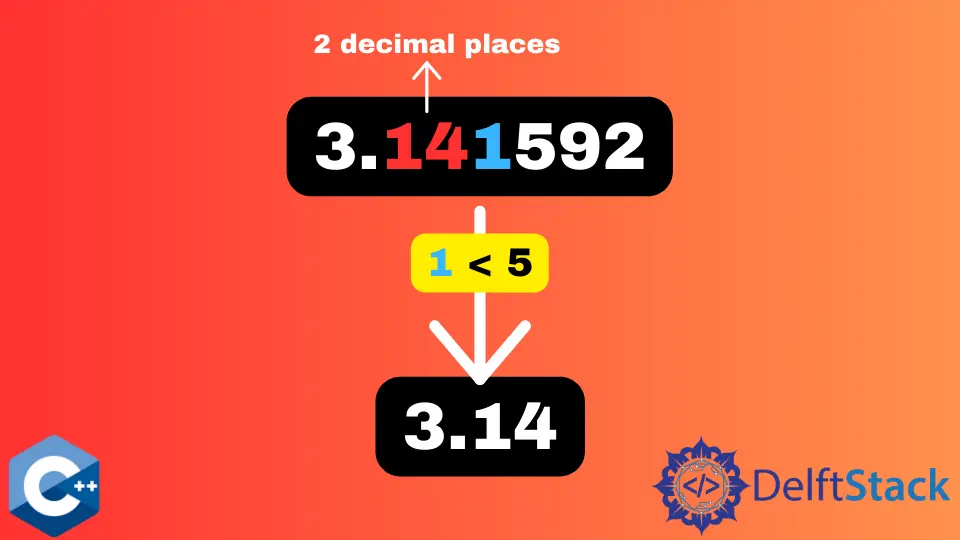
本文將介紹幾種在 C++ 中如何將浮點數四捨五入到兩位小數的方法。
在 C++ 中使用 printf
函式格式說明符將浮點數四捨五入為 2 個小數
浮點數具有特殊的二進位制表示形式,這意味著機器無法精確表示實數。因此,在對浮點數進行運算並對其進行計算時,通常要藉助於舍入函式。但是,在這種情況下,我們只需要輸出數字的小數部分。
第一種解決方案是利用 printf
函式將格式化的文字輸出到 stdout
流。注意,浮點數數字的常規格式說明符-%f
應修改為%.2f
。後一種表示法確保從該數字僅列印 2 個小數,並且同時根據通用數學規則進行舍入。請注意,其他資料型別的格式說明符也可以使用類似的符號。
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<double> floats{-3.512312, -21.1123, -1.99, 0.129, 2.5, 3.111};
for (auto &item : floats) {
printf("%.2f; ", item);
}
return EXIT_SUCCESS;
}
輸出:
-3.51; -21.11; -1.99; 0.13; 2.50; 3.11;
在 C++ 中使用 fprintf
函式格式說明符將浮點數舍入到 2 位小數
fprintf
是另一個可以用來做輸出格式化的函式,就像 printf
呼叫一樣,此外,它可以寫入任何可以作為第一個引數傳遞的 FILE*
流物件。在下面的示例中,我們演示了列印到 stderr
流,這是 stdout
的無緩衝版本,用於錯誤報告和日誌記錄。
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<double> floats{-3.512312, -21.1123, -1.99, 0.129, 2.5, 3.111};
for (auto &item : floats) {
fprintf(stderr, "%.2f; ", item);
}
return EXIT_SUCCESS;
}
輸出:
-3.51; -21.11; -1.99; 0.13; 2.50; 3.11;
在 C++ 中使用 std::setprecision
和 std::fixed
將浮點數舍入為 2 個小數
或者,可以將 I/O 機械手庫中的 std::setprecision
函式與 std::fixed
一起使用。後者用於修改浮點輸入/輸出操作的預設格式。如果我們將其與 std::setprecision
一起使用,則結果是實數的固定精度,並且可以通過傳遞給 std::setprecision
本身的整數引數來指定精度。
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::fixed;
using std::setprecision;
using std::vector;
int main() {
vector<double> floats{-3.512312, -21.1123, -1.99, 0.129, 2.5, 3.111};
for (auto &item : floats) {
cout << setprecision(2) << fixed << item << "; ";
}
return EXIT_SUCCESS;
}
輸出:
-3.51; -21.11; -1.99; 0.13; 2.50; 3.11;
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu