Pandas DataFrame DataFrame.drop_duplicates() 函式
Suraj Joshi
2023年1月30日
Pandas
Pandas DataFrame
-
pandas.DataFrame.drop_duplicates()
的語法 -
示例程式碼:使用 Pandas
DataFrame.set_index()
方法刪除重複的行 -
示例程式碼設定
subset
引數的 PandasDataFrame.set_index()
方法 -
示例程式碼:設定
keep
引數 PandasDataFrame.set_index()
方法 -
示例程式碼:設定
ignore_index
引數的 PandasDataFrame.set_index()
方法
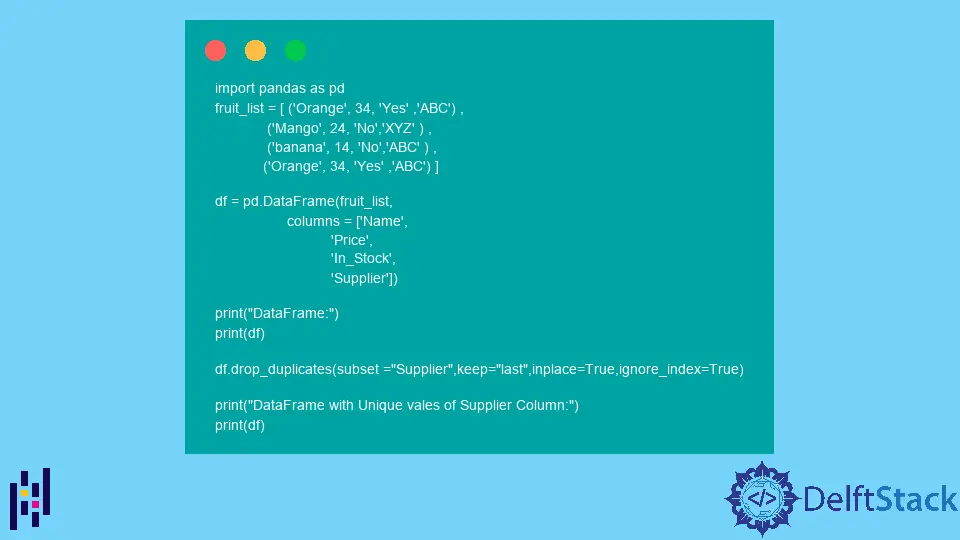
Python Pandas DataFrame.drop_duplicates()
函式從 DataFrame
中刪除所有重複的行。
pandas.DataFrame.drop_duplicates()
的語法
DataFrame.drop_duplicates(subset: Union[Hashable, Sequence[Hashable], NoneType]=None,
keep: Union[str, bool]='first',
inplace: bool=False,
ignore_index: bool=False)
引數
subset |
列標籤或標籤序列。識別重複時需要考慮的列 |
keep |
first 、last 或 False 。刪除除第一個以外的所有重複資料(keep=first ),刪除除最後一個以外的所有重複資料(keep=last )或刪除所有重複資料(keep=False ) |
inplace |
Boolean.如果為 True ,修改呼叫者的 DataFrame 。 |
ignore_index |
布林型。如果是 True ,則忽略原始 DataFrame 中的索引,預設值是 False ,即使用索引。預設值是 False ,表示使用索引。 |
返回值
如果 inplace
為 True
,則從 DataFrame
中刪除所有重複的行,否則為 None
。
示例程式碼:使用 Pandas DataFrame.set_index()
方法刪除重複的行
import pandas as pd
fruit_list = [ ('Orange', 34, 'Yes' ,'ABC') ,
('Mango', 24, 'No','XYZ' ) ,
('banana', 14, 'No','BCD' ) ,
('Orange', 34, 'Yes' ,'ABC') ]
df = pd.DataFrame(fruit_list,
columns = ['Name',
'Price',
'In_Stock',
'Supplier'])
print("DataFrame:")
print(df)
df_unique=df.drop_duplicates()
print("DataFrame with Unique Rows:")
print(df_unique)
輸出:
DataFrame:
Name Price In_Stock Supplier
0 Orange 34 Yes ABC
1 Mango 24 No XYZ
2 banana 14 No BCD
3 Orange 34 Yes ABC
DataFrame with Unique Rows:
Name Price In_Stock Supplier
0 Orange 34 Yes ABC
1 Mango 24 No XYZ
2 banana 14 No BCD
原始的 DataFrame
的第 1 行和第 4 行是相同的。
你可以通過使用 drop_duplicates()
方法從 DataFrame 中刪除所有重複的行。
示例程式碼設定 subset
引數的 Pandas DataFrame.set_index()
方法
import pandas as pd
fruit_list = [ ('Orange', 34, 'Yes' ,'ABC') ,
('Mango', 24, 'No','XYZ' ) ,
('banana', 14, 'No','ABC' ) ,
('Orange', 34, 'Yes' ,'ABC') ]
df = pd.DataFrame(fruit_list,
columns = ['Name',
'Price',
'In_Stock',
'Supplier'])
print("DataFrame:")
print(df)
df_unique=df.drop_duplicates(subset ="Supplier")
print("DataFrame with Unique vales of Supplier Column:")
print(df_unique)
輸出:
DataFrame:
Name Price In_Stock Supplier
0 Orange 34 Yes ABC
1 Mango 24 No XYZ
2 banana 14 No ABC
3 Orange 34 Yes ABC
DataFrame with Unique vales of Supplier Column:
Name Price In_Stock Supplier
0 Orange 34 Yes ABC
1 Mango 24 No XYZ
該方法刪除 DataFrame 中所有不具有 Supplier
列唯一值的行。
在這裡,第 1、3 和 4 行的 Supplier
列有一個共同的值。因此,第 3 和第 4 行將被從 DataFrame
中刪除;預設情況下,第一條重複的行不會被刪除。
示例程式碼:設定 keep
引數 Pandas DataFrame.set_index()
方法
import pandas as pd
fruit_list = [ ('Orange', 34, 'Yes' ,'ABC') ,
('Mango', 24, 'No','XYZ' ) ,
('banana', 14, 'No','ABC' ) ,
('Orange', 34, 'Yes' ,'ABC') ]
df = pd.DataFrame(fruit_list,
columns = ['Name',
'Price',
'In_Stock',
'Supplier'])
print("DataFrame:")
print(df)
df_unique=df.drop_duplicates(subset ="Supplier",keep="last")
print("DataFrame with Unique vales of Supplier Column:")
print(df_unique)
輸出:
DataFrame:
Name Price In_Stock Supplier
0 Orange 34 Yes ABC
1 Mango 24 No XYZ
2 banana 14 No ABC
3 Orange 34 Yes ABC
DataFrame with Unique vales of Supplier Column:
Name Price In_Stock Supplier
1 Mango 24 No XYZ
3 Orange 34 Yes ABC
該方法刪除 DataFrame
中所有在 Supplier
列中沒有唯一值的行,只保留最後一條重複的行。
在這裡,第 1,3,4 行的 Supplier
列有一個共同的值。所以第 1 和第 3 行將從 DataFrame
中刪除。
示例程式碼:設定 ignore_index
引數的 Pandas DataFrame.set_index()
方法
import pandas as pd
fruit_list = [ ('Orange', 34, 'Yes' ,'ABC') ,
('Mango', 24, 'No','XYZ' ) ,
('banana', 14, 'No','ABC' ) ,
('Orange', 34, 'Yes' ,'ABC') ]
df = pd.DataFrame(fruit_list,
columns = ['Name',
'Price',
'In_Stock',
'Supplier'])
print("DataFrame:")
print(df)
df.drop_duplicates(subset ="Supplier",keep="last",inplace=True,ignore_index=True)
print("DataFrame with Unique vales of Supplier Column:")
print(df)
輸出:
DataFrame:
Name Price In_Stock Supplier
0 Orange 34 Yes ABC
1 Mango 24 No XYZ
2 banana 14 No ABC
3 Orange 34 Yes ABC
DataFrame with Unique vales of Supplier Column:
Name Price In_Stock Supplier
0 Mango 24 No XYZ
1 Orange 34 Yes ABC
這裡,由於 ignore_index
被設定為 True
,原 DataFrame
中的索引被忽略,併為該行設定新的索引。
由於 inplace=True
函式的作用,在呼叫 ignore_index()
函式後,原 DataFrame
被修改。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn