Pandas DataFrame DataFrame.to_excel() 函式
Jinku Hu
2023年1月30日
Pandas
Pandas DataFrame
-
pandas.DataFrame.to_excel()
語法 -
示例程式碼:Pandas
DataFrame.to_excel()
-
示例程式碼:Pandas
DataFrame.to_excel()
和ExcelWriter
-
示例程式碼:Pandas
DataFrame.to_excel
來追加到一個現有的 Excel 檔案中 -
示例程式碼:Pandas
DataFrame.to_excel
寫入 Excel 中的多頁 -
示例程式碼: 帶有引數
header
的 PandasDataFrame.to_excel
-
示例程式碼: 當
index=False
時的 PandasDataFrame.to_excel
-
示例程式碼:Pandas
DataFrame.to_excel
引數為index_label
-
示例程式碼:Pandas
DataFrame.to_excel
與float_format
引數 -
示例程式碼:Pandas
DataFrame.to_excel
引數為freeze_panes
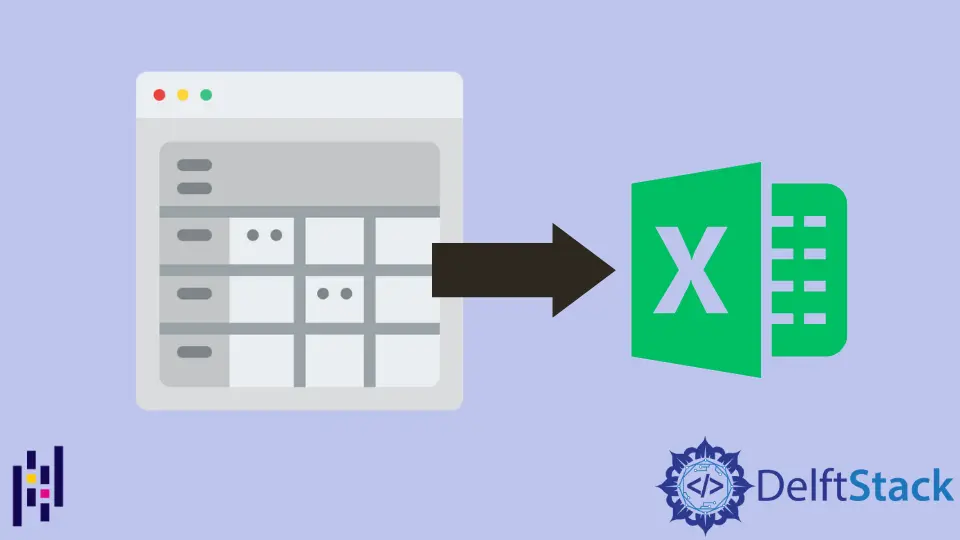
Python Pandas DataFrame.to_excel(values)
函式將 DataFrame 資料轉儲到 Excel 檔案中,單頁或多頁中。
pandas.DataFrame.to_excel()
語法
DataFrame.isin(
excel_writer,
sheet_name="Sheet1",
na_rep="",
float_format=None,
columns=None,
header=True,
index=True,
index_label=None,
startrow=0,
startcol=0,
engine=None,
merge_cells=True,
encoding=None,
inf_rep="inf",
verbose=True,
freeze_panes=None,
)
引數
excel_writer |
Excel 檔案路徑或現有的 pandas.ExcelWriter |
sheet_name |
DataFrame 轉儲到的工作表名稱 |
na_rep |
空值的表示方法 |
float_format |
浮點數的格式 |
header |
指定生成的 Excel 檔案的標題 |
index |
如果為 True ,將 DataFrame index 寫入 Excel |
index_label |
索引列的列標籤 |
startrow |
將資料寫入 Excel 的左上角單元格行。 預設為 0 |
startcol |
將資料寫入 Excel 的左上角單元格。 預設為 0 |
engine |
可選引數,用於指定要使用的引擎。openyxl 或 xlswriter |
merge_cells |
合併 MultiIndex 到合併的單元格中 |
encoding |
輸出 Excel 檔案的編碼。只有當使用 xlwt 寫入器時才需要,其他寫入器原生支援 Unicode |
inf_rep |
無限大的表示方法。預設為 inf |
verbose |
如果為 True ,錯誤日誌包含更多的資訊 |
freeze_panes |
指定凍結窗格的最底部和最右側。它是基於一的,但不是基於零的 |
返回值
None
示例程式碼:Pandas DataFrame.to_excel()
import pandas as pd
dataframe= pd.DataFrame({'Attendance': [60, 100, 80, 78, 95],
'Name': ['Olivia', 'John', 'Laura', 'Ben', 'Kevin'],
'Marks': [90, 75, 82, 64, 45]})
dataframe.to_excel('test.xlsx')
呼叫者 DataFrame
為
Attendance Name Marks
0 60 Olivia 90
1 100 John 75
2 80 Laura 82
3 78 Ben 64
4 95 Kevin 45
test.xlsx
檔案被建立。
示例程式碼:Pandas DataFrame.to_excel()
和 ExcelWriter
上面的例子使用檔案路徑作為 excel_writer
,我們也可以使用 pandas.Excelwriter
來指定 DataFrame 轉儲的 excel 檔案。
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": [60, 100, 80, 78, 95],
"Name": ["Olivia", "John", "Laura", "Ben", "Kevin"],
"Marks": [90, 75, 82, 64, 45],
}
)
with pd.ExcelWriter("test.xlsx") as writer:
dataframe.to_excel(writer)
示例程式碼:Pandas DataFrame.to_excel
來追加到一個現有的 Excel 檔案中
import pandas as pd
import openpyxl
dataframe = pd.DataFrame(
{
"Attendance": [60, 100, 80, 78, 95],
"Name": ["Olivia", "John", "Laura", "Ben", "Kevin"],
"Marks": [90, 75, 82, 64, 45],
}
)
with pd.ExcelWriter("test.xlsx", mode="a", engine="openpyxl") as writer:
dataframe.to_excel(writer, sheet_name="new")
我們應該指定引擎為 openpyxl
,而不是預設的 xlsxwriter
;否則,我們會得到 xlswriter
不支援 append
模式的錯誤資訊。
ValueError: Append mode is not supported with xlsxwriter!
因為 openpyxl
不屬於 pandas 的一部分,所以要安裝和匯入。
pip install openpyxl
示例程式碼:Pandas DataFrame.to_excel
寫入 Excel 中的多頁
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": [60, 100, 80, 78, 95],
"Name": ["Olivia", "John", "Laura", "Ben", "Kevin"],
"Marks": [90, 75, 82, 64, 45],
}
)
with pd.ExcelWriter("test.xlsx") as writer:
dataframe.to_excel(writer, sheet_name="Sheet1")
dataframe.to_excel(writer, sheet_name="Sheet2")
它將 DataFrame 物件轉儲到 Sheet1
和 Sheet2
。
如果你指定了 columns
引數,你也可以將不同的資料寫入多個表。
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": [60, 100, 80, 78, 95],
"Name": ["Olivia", "John", "Laura", "Ben", "Kevin"],
"Marks": [90, 75, 82, 64, 45],
}
)
with pd.ExcelWriter("test.xlsx") as writer:
dataframe.to_excel(writer, columns=["Name", "Attendance"], sheet_name="Sheet1")
dataframe.to_excel(writer, columns=["Name", "Marks"], sheet_name="Sheet2")
示例程式碼: 帶有引數 header
的 Pandas DataFrame.to_excel
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": [60, 100, 80, 78, 95],
"Name": ["Olivia", "John", "Laura", "Ben", "Kevin"],
"Marks": [90, 75, 82, 64, 45],
}
)
with pd.ExcelWriter("test.xlsx") as writer:
dataframe.to_excel(writer, header=["Student", "First Name", "Score"])
在建立的 Excel 檔案中,預設的標題與 DataFrame 的列名相同。header
引數指定新的標題,以取代預設的標題。
示例程式碼: 當 index=False
時的 Pandas DataFrame.to_excel
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": [60, 100, 80, 78, 95],
"Name": ["Olivia", "John", "Laura", "Ben", "Kevin"],
"Marks": [90, 75, 82, 64, 45],
}
)
with pd.ExcelWriter("test.xlsx") as writer:
dataframe.to_excel(writer, index=False)
index = False
指定 DataFrame.to_excel()
生成一個沒有頭行的 Excel 檔案。
示例程式碼:Pandas DataFrame.to_excel
引數為 index_label
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": [60, 100, 80, 78, 95],
"Name": ["Olivia", "John", "Laura", "Ben", "Kevin"],
"Marks": [90, 75, 82, 64, 45],
}
)
with pd.ExcelWriter("test.xlsx") as writer:
dataframe.to_excel(writer, index_label="id")
index_label='id'
設定索引列的列名為 id
。
示例程式碼:Pandas DataFrame.to_excel
與 float_format
引數
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": [60, 100, 80, 78, 95],
"Name": ["Olivia", "John", "Laura", "Ben", "Kevin"],
"Marks": [90, 75, 82, 64, 45],
}
)
with pd.ExcelWriter("test.xlsx") as writer:
dataframe.to_excel(writer, float_format="%.1f")
float_format="%.1f"
指定浮點數小數點後有兩位。
示例程式碼:Pandas DataFrame.to_excel
引數為 freeze_panes
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": [60, 100, 80, 78, 95],
"Name": ["Olivia", "John", "Laura", "Ben", "Kevin"],
"Marks": [90, 75, 82, 64, 45],
}
)
with pd.ExcelWriter("test.xlsx") as writer:
dataframe.to_excel(writer, freeze_panes=(1, 1))
freeze_panes=(1,1)
指定 excel 檔案有凍結的頂行和凍結的第一列。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu