Python Tutorial - Namescape and Scope
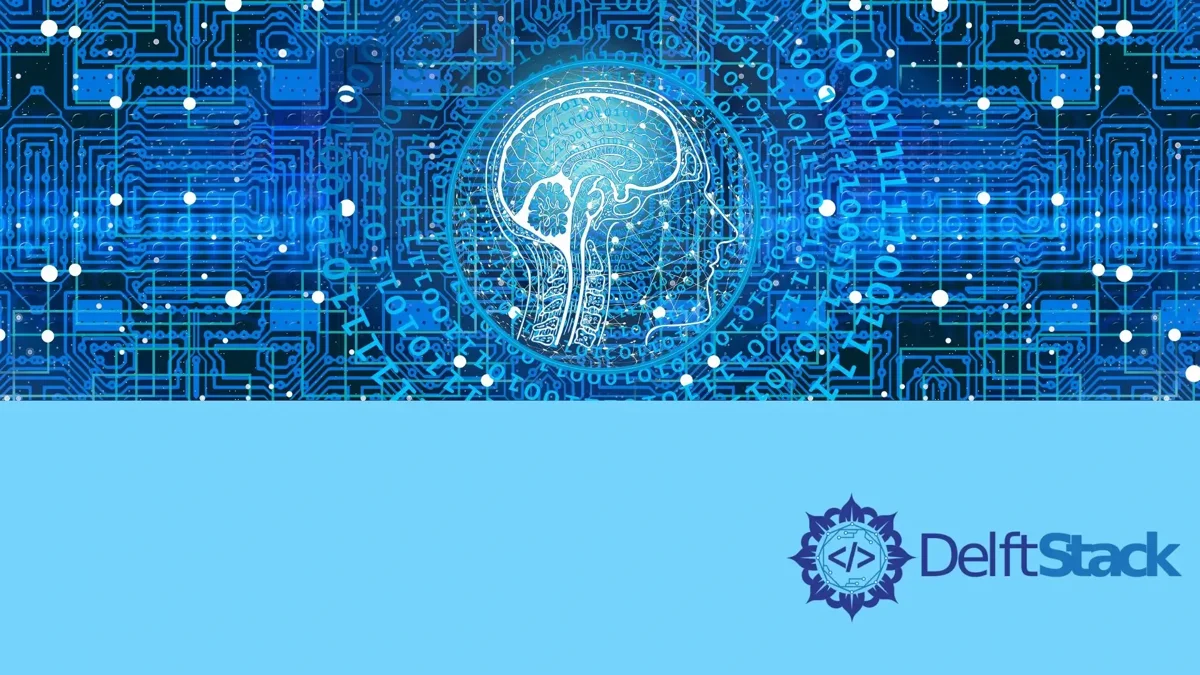
In this section, you will learn namespace and scope of a variable in Python programming.
Name in Python
A name in Python is an identifier given to the object. Python is an object-oriented programming language which means everything is an object in Python and names are used to access objects.
For instance, when you assign a value to a variable x = 3
. Here 3
is an object and is stored at a memory location (RAM). This memory location is a named memory location that could be accessed by the name x
. The address of this object (3) can be obtained by using the built-in function id()
.
x = 3
print("id(3) =", id(3))
print("id(x) =", id(x))
id(3) = 1864160336
id(x) = 1864160336
Now here object 3
is stored in a location whose name is x
and hence the address of both of them is the same.
When you assign the value of x
to some other variable let’s say y
and change value of x
, then x
will have a new location and y
will have the location of previous x
. This is demonstrated below:
x = 3
print("id(x) =", id(x))
x = 4
print("id(4) =", id(4))
print("id(x) =", id(x))
y = 3
print("id(y) =", id(y))
id(x) = 1864160336
id(4) = 1864160352
id(x) = 1864160352
id(y) = 1864160336
You can see here the address of y
equals that of old x
.
Namespace in Python
A namespace is a collection of names. A namespace is a mapping of name to an object and is implemented as a dictionary in Python. Namespaces ensure that every name used in a program is unique.
Namespaces are created when the interpreter is started, deleted when program execution is ended. This namespace contains all the built-in names, so whenever you need a built-in function for example id()
you can use it directly in your program.
When you call a function, a local namespace that contains all the defined names is created.
Variable Scope in Python
When you create a variable in your program, you may not have access to that variable from every part of the program. This is because of the scope of variables. You try to access variables from the namespace where they are not defined.
A scope can be defined as a place from where you can access your namespace without any prefix.
The Scope can be categorized as:
- Scope of a function where you have local names.
- Scope of a module where you have global variables.
- Outermost scope where you have built-in names.
When you create a reference inside a function, it is searched in the local namespace first and then it is searched in global namespace; if it is not found anywhere it will be searched in the built-in namespace.
When creating a nested function, you will have a new scope inside of the local scope.
Use Scope and Namespace in Python
def outer():
x = 12
def inner():
y = 13
z = 14
Here, a nested function is defined (function inside another function). Variable y
is in the nested local namespace of the inner function, variable x
is in the local namespace of the outer function and variable z
is in the global namespace.
When it is inside the inner function, variable y
is a local variable, x
is non-local and z
is global. The inner function has no access to non-local x
and global z
variables.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook