How to Check a Radio Button Using JavaScript or jQuery
- Check Radio Button Using JavaScript
- Check Radio Button Using jQuery
- Check the Radio Button Based on a Button Click
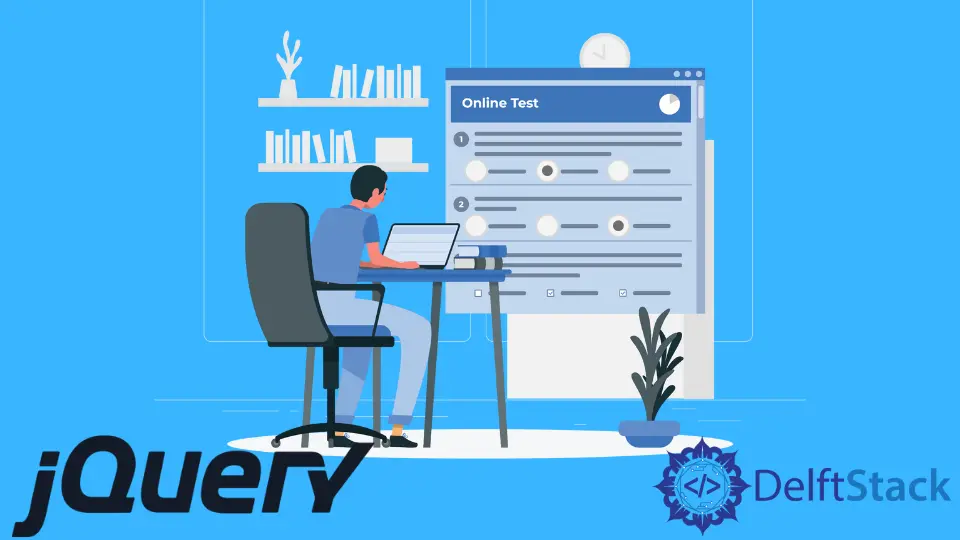
We often use client-side scripting like jQuery or JavaScript, to create interactive and fast performing websites and can complete simple operations like form validations efficiently without any extra trip to the webserver.
Given a scenario where we want a radio button selected by default or on a button click event, we can use JavaScript or jQuery.
Let us begin by defining a form.
<div id='radiobuttonset'>
<input type='radio' id='myradio_1' name='radiobutton' value='1' />1
<input type='radio' id='myradio_2' name='radiobutton' value='2' />2
<input type='radio' id='myradio_3' name='radiobutton' value='3' />3
</div>
Check Radio Button Using JavaScript
Select the Radio Button Using ID
One of the best possible ways to preselect a radio button is to refer to it by its ID. To select the DOM element using ID, we add the prefix #
to the ID and set the checked value as true.
document.querySelector('#myradio_1').checked = true;
Output: Radio button 1 is selected.
Select the Radio Button Using the Value
An alternate option is to use the value of the radio button to preselect the radio button. Selection by value can be useful in scenarios where we don’t know the ID of a Radio Button but know the value.
We can use the ID of the parent div and select the element whose value is 2. Then we can set the checked
attribute to be true
.
document.querySelector('#radiobuttonset > [value="2"]').checked = true;
Output: Radio button 2 is selected.
In some cases, where we know that there are no other elements in the DOM equal to the predefined value, we can select the element by just checking if the value. In this case, we will not need the parent ID.
document.querySelector('[value="3"]').checked = true;
Output: Radio button 3 is selected.
Check Radio Button Using jQuery
Apart from using JavaScript, we can also use the jQuery library to check a radio button based on a predefined value. jQuery is a library, built using JavaScript. It helps to simplify the HTML DOM traversal and manipulation. The syntax for jQuery is also simpler than JavaScript.
To add use jQuery add a reference to the jQuery library either from CDN or from a local file.
Select the Radio Button Using ID
Like JavaScript, to access the DOM element using ID, we use the prefix #
. However, the exact syntax depends on the release of jQuery used.
The current stable jQuery version is 3.4.1.
the Version of jQuery Is Equal or Above (>=) 1.6
If the version of jQuery used is greater than or equal to 1.6, we use prop
to set the checked attribute to be true.
$('#myradio_1').prop('checked', true);
Output: Radio button 1 is selected.
the Version of jQuery Is Versions Before (<) 1.6
If the jQuery version is before 1.6, we could update the attr
as checked.
$('#myradio_2').attr('checked', 'checked');
Output: Radio button 2 is selected
Select the Radio Button by Using the Value
We can also update the selected value for the radio button based on the value of the button.
$('input[name=radiobutton][value=\'1\']').prop('checked', true);
Output: Radio button 2 is selected
Check the Radio Button Based on a Button Click
Once we know how to update the radio button using JavaScript or jQuery, we can update the selection based on some event like a button click or any by some other element selection. To test it, let us update our form to add a button.
<div id='radiobuttonset'>
<input type='radio' id='myradio_1' name='radiobutton' value='1' />1
<input type='radio' id='myradio_2' name='radiobutton' value='2' />2
<input type='radio' id='myradio_3' name='radiobutton' value='3' />3
</div>
<button id="button_1">check 1</button>
We can then add a click
event on the button. On click, it selects the first radio button.
$('#button_1').click(function() {
$('input[name=radiobutton]').val(['1']);
});
Output: Radio button 1 is selected