JavaScript GUID
-
Use the
math.random()
to Create aguid
in JavaScript -
Use the Regular Expressions to Create a
guid
in JavaScript
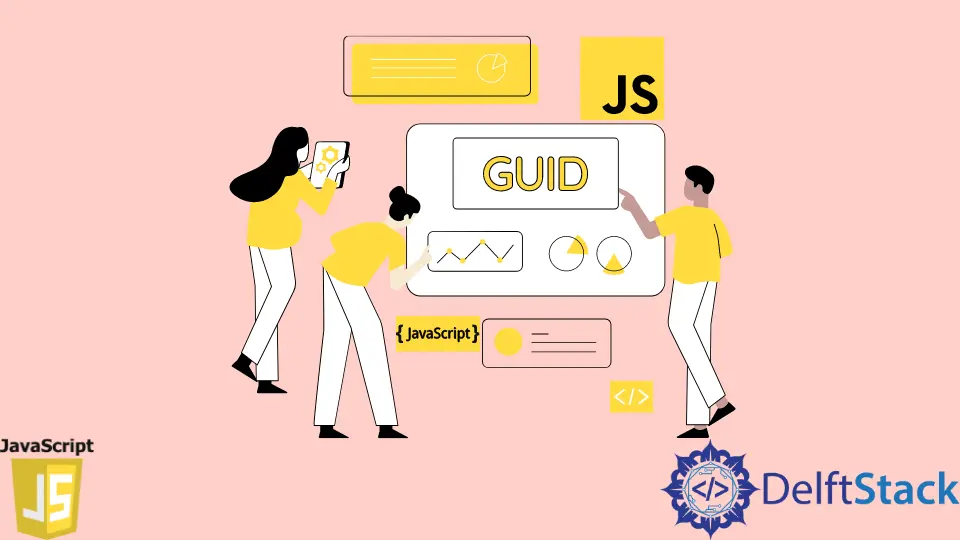
Globally Unique Identifiers or guid
is used by software programs to uniquely identify a data object’s location. Some examples of data that include guid
are streaming media files, Windows registry entries, database keys, and various other file types.
In this tutorial, we will create a guid
in JavaScript.
Use the math.random()
to Create a guid
in JavaScript
The math.random()
function returns a decimal value between 0 and 1 with 16 digits after the decimal fraction point (for example, 0.2451923368509859). We can then scale this random value according to the desired range.
The following example shows its implementation in creating a guid
in JavaScript.
var ID = function() {
return '_' + Math.random().toString(36).substr(2, 9);
};
Math.random().toString(36).slice(2);
function uuidv4() {
return 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function(c) {
var r = Math.random() * 16 | 0, v = c == 'x' ? r : (r & 0x3 | 0x8);
return v.toString(16);
});
}
console.log(uuidv4());
function uuid() {
return 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function(c) {
var r = Math.random() * 16 | 0, v = c == 'x' ? r : (r & 0x3 | 0x8);
return v.toString(16);
});
}
var userID = uuid();
Output:
315a0369-05c9-4165-8049-60e2489ea8e5
We take a string and randomly replace characters of that string to generate a guid
using this method.
Use the Regular Expressions to Create a guid
in JavaScript
Regular expressions are the patterns that are used to match character combinations in a string. They are objects in JavaScript. We can use such patterns with different functions to perform various operations with strings.
We can use such patterns in creating a guid
in JavaScript.
See the code below.
function create_UUID() {
var dt = new Date().getTime();
var uuid =
'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function(c) {
var r = (dt + Math.random() * 16) % 16 | 0;
dt = Math.floor(dt / 16);
return (c == 'x' ? r : (r & 0x3 | 0x8)).toString(16);
});
return uuid;
}
console.log(create_UUID());
Output:
4191eebf-8a5b-4136-bfa0-6a594f4f0a03
Note that in this method, we also need to use the Math.random()
function because it ensures that every output will return with a unique ID.