How to Convert Array to Object in JavaScript
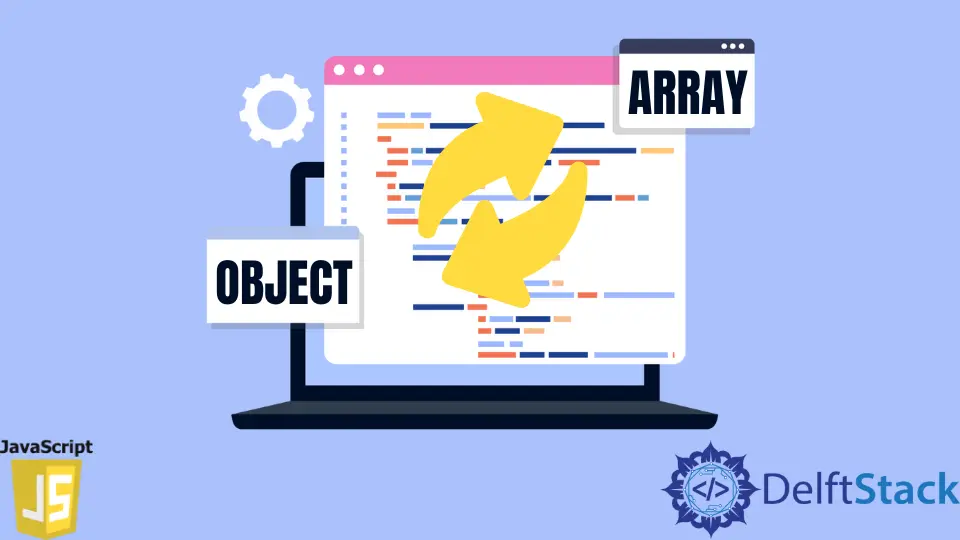
Arrays efficiently store multiple values, offering versatility for ordered collections. In contrast, Objects excel in structuring data by associating variables with values, making them ideal for representing entities with diverse attributes.
We use arrays when we store multiple values in a single variable, while an object can hold multiple variables with their values. Generally, employing objects tends to be more efficient when dealing with sizable datasets.
Converting an array of objects into a single object is a common requirement in JavaScript, especially when dealing with structured data.
Methods to Convert an Array to an Object in JavaScript
We’ll explore various methods to convert array of objects to object JavaScript. By examining techniques such as Object.assign()
, array.reduce()
, and the spread operator (...
), along with loop-based strategies like for...in
, developers can gain insights into diverse approaches for achieving this common task.
Let’s discuss the methods in the following section.
Using the Object.assign()
Method
Using the Object.assign()
method in JavaScript involves merging the properties of one or more source objects into a target object. This method is commonly employed to convert an array of objects into a single object.
Basic Syntax:
const target = Object.assign(target, source1, source2, ...);
In the syntax, the target
is the object to which the properties of the source objects will be copied. The source1, source2, ...
is the one or more source objects whose properties will be copied to the target object.
Let’s have an example.
Example:
const array = ['foo', 'boo', 'zoo'];
const obj = Object.assign({}, array);
console.log(obj)
In the above code, we initialize an array with values 'foo', 'boo', and 'zoo'
. Using the Object.assign()
method, we create a new object obj
by merging the array elements into an empty object {}
.
The Object.assign()
method iteratively copies the properties of the source object (in this case, the array
) into the target object (an initially empty object). Finally, we log the resulting object obj
to the console.
Output:
The output line demonstrates the conversion of the array into an object, with numeric indices as keys. The output string above will look like { '0': 'foo', '1': 'boo', '2': 'zoo' }
.
Using the array.reduce()
Method
Using the reduce()
method method in JavaScript involves applying a provided function to each element in an array, accumulating the results into a single value. This method is often used to convert an array of objects into a single object.
We can use it to make the necessary conversion.
Basic Syntax:
const result = array.reduce(function(accumulator, currentValue, currentIndex, array) {
// Your logic here
return updatedAccumulator;
}, initialValue);
In the syntax of the reduce()
method, the array
parameter denotes the array undergoing reduction, and accumulator
is the variable accumulating results. The currentValue
represents the current array element.
Optionally, currentIndex
is the index of the current element, and array
designates the value of the array on which reduce()
function is called. Lastly, initialValue
is an optional initial value for the accumulator
.
Let’s have an example.
Example:
const array = ['foo', 'boo', 'zoo'];
const resultObject = array.reduce(function(target, key, index) {
target[index] = key;
return target;
}, {});
console.log(resultObject);
In the above code, we initialize an array with values 'foo', 'boo', and 'zoo'
. Using the array.reduce()
method, we transform this array into a single object named resultObject
.
The method iterates through each element, assigning them to keys with their numeric indices in the target object.
Output:
The output demonstrates the successful conversion of the array into an object, with keys corresponding to the numeric indices and values being mapped to the original array elements, resulting in { '0': 'foo', '1': 'boo', '2': 'zoo' }
. The reduce()
method proves versatile for more complex transformations of array elements into objects.
Using the Spread Operator (...
)
Using the operator (...
) in JavaScript involves unpacking the elements of an array or the properties of an object. When it comes to converting an array of objects into a single object, the spread operator can be utilized for a concise and modern approach.
Let’s have an example.
Example:
const arr = ['foo', 'boo', 'zoo'];
const obj = {...arr};
console.log(obj);
In the above code, we create an array arr
and assign values 'foo', 'boo', and 'zoo'
. Using the operator (...
), we effortlessly convert this array into a new object obj
.
Output:
The resulting object has keys representing numeric indices and values as the original array elements. The output, displayed as { '0': 'foo', '1': 'boo', '2': 'zoo' }
.
Use for...in
Loop to Convert an Array to an Object
Using a loop to convert an array of objects into one object in JavaScript involves iterating through the array and dynamically creating key-value pairs in a new object. This process is typically done with a for...in
loop.
Basic Syntax:
for (variable in object) {
// code block to be executed for each property
}
In the syntax, the variable
represents the property name for each iteration. The object
is the enumerable properties the loop is iterating.
Let’s have an example.
Example:
const array = ['foo', 'boo', 'zoo'];
let mergedObject = {};
for (const index in array) {
mergedObject[`key${parseInt(index) + 1}`] = array[index];
}
console.log(mergedObject);
In this code, we convert the array ['foo', 'boo', 'zoo']
into an object named mergedObject
using a for...in
loop. Dynamically generating keys like 'key1'
, 'key2'
, etc., we assign them to the object with corresponding values from the array.
The resulting object is then logged to the console.
Output:
The output showcase the successful conversion with keys as 'key1', 'key2', 'key3'
, and values as 'foo', 'boo', 'zoo'
, respectively. This method, while concise, provides control over the key generation process.
Faq
Q1: Why Would I Need to Convert an Array of Objects Into a Single Object in JavaScript?
A1: Converting an array of objects into a single object is often necessary when dealing with structured data or when you want to aggregate information from multiple sources. This transformation facilitates easier data manipulation, access, and retrieval of combine objects, especially when working with APIs, databases, or scenarios where a flattened representation of the data is more convenient.
Q2: What Is the Difference Between Using Object.assign()
, array.reduce()
, and the Spread Operator (...
) to Convert an Array of Objects Into a Single Object?
A2: The three methods—Object.assign()
, array.reduce()
, and the spread operator (...
)—all achieve the goal of converting an array of objects into one object, but they differ in syntax and usage. Object.assign()
and the spread operator (...
) provide concise and modern solutions.
However, it’s important to note that while array.reduce()
may not be the best answer to the solution, it allows for more customized transformations.
Conclusion
In summary, this article delves into various approaches for converting arrays of objects to a single object in JavaScript. It covers methods like Object.assign()
, array.reduce()
, and the spread operator (...
), along with a thorough examination of loop-based strategies like for...in
.
Each method provides distinct advantages, allowing developers to choose based on their preferences and project requirements. This knowledge empowers developers to efficiently handle array-to-object conversions and enhances their overall proficiency in data manipulation and programming versatility.