How to Set Window Icon in Tkinter
-
Method 1: Using the
iconbitmap
Method -
Method 2: Using the
photo
Method with PNG or GIF - Method 3: Setting the Icon Dynamically
- Conclusion
- FAQ
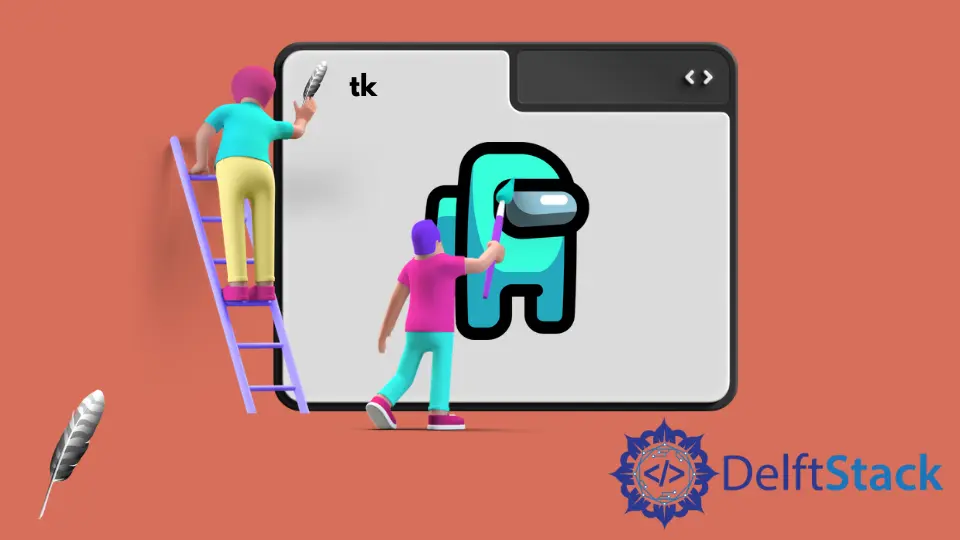
Setting a window icon in Tkinter is an essential aspect of creating a visually appealing and user-friendly application. The window icon not only enhances the aesthetics of your application but also helps users identify your program quickly among multiple open windows.
In this tutorial, we will explore the various methods to set a custom icon for your Tkinter window. Whether you are a beginner or an experienced developer, this guide will provide you with clear, step-by-step instructions to achieve your goal. By the end of this article, you will have the knowledge and skills to personalize your Tkinter applications effectively.
Method 1: Using the iconbitmap
Method
The iconbitmap
method is one of the simplest ways to set a window icon in Tkinter. This method allows you to specify an icon file in the .ico format. The file can be an absolute path or a relative path from your script’s location. Here’s how you can do it:
import tkinter as tk
root = tk.Tk()
root.title("My Application")
root.iconbitmap('path/to/icon.ico')
root.geometry("400x300")
label = tk.Label(root, text="Hello, Tkinter!")
label.pack(pady=20)
root.mainloop()
In this example, we start by importing the Tkinter library and creating a root window. We set the title of the window and then use the iconbitmap
method to specify the path to our icon file. The geometry method is used to define the size of the window. Finally, we create a label to display some text and start the Tkinter main loop.
Output:
The iconbitmap
method is straightforward but requires the icon to be in .ico format. If you have an image in another format, you will need to convert it to .ico before using it. This method is perfect for those who want a quick and effective way to set an icon without getting into complex configurations.
Method 2: Using the photo
Method with PNG or GIF
If you prefer to use a PNG or GIF file instead of an ICO file, you can utilize the PhotoImage
class in Tkinter. This method allows for more flexibility in icon design and file types. Here’s how to do it:
import tkinter as tk
root = tk.Tk()
root.title("My Application")
icon_image = tk.PhotoImage(file='path/to/icon.png')
root.iconphoto(False, icon_image)
root.geometry("400x300")
label = tk.Label(root, text="Hello, Tkinter!")
label.pack(pady=20)
root.mainloop()
In this code snippet, we create a PhotoImage
object by loading a PNG file. We then use the iconphoto
method to set this image as the window icon. The first parameter is set to False
, which indicates that we are not replacing the default icon. The rest of the code remains the same as in the previous example.
Output:
A window will appear with the specified PNG icon.
Using the PhotoImage
method is beneficial when you want to use more modern image formats like PNG or GIF. This approach can also allow for more intricate designs and higher-quality icons, making your application visually appealing. However, keep in mind that PhotoImage
does not support all image formats, so ensure your image is in a compatible format.
We need to set the image as tk.PhotoImage
but not the image itself, otherwise we will have a _tkinter.TclError
error.
Method 3: Setting the Icon Dynamically
Sometimes, you may want to set the window icon dynamically based on user actions or other events. You can achieve this by updating the icon during runtime. Here’s a simple example:
import tkinter as tk
def change_icon():
new_icon = tk.PhotoImage(file='path/to/new_icon.png')
root.iconphoto(False, new_icon)
root = tk.Tk()
root.title("My Application")
default_icon = tk.PhotoImage(file='path/to/default_icon.png')
root.iconphoto(False, default_icon)
root.geometry("400x300")
change_button = tk.Button(root, text="Change Icon", command=change_icon)
change_button.pack(pady=20)
label = tk.Label(root, text="Hello, Tkinter!")
label.pack(pady=20)
root.mainloop()
In this example, we define a function called change_icon
, which loads a new icon and sets it as the window’s icon when called. The main window initially uses a default icon. A button labeled “Change Icon” allows users to trigger the icon change. When the button is clicked, the window’s icon updates to the new image.
This dynamic approach is particularly useful for applications that require user interaction or changing themes. It enhances user experience by providing visual feedback based on actions. Remember that the new icon must be in a compatible format, such as PNG or GIF, when using the PhotoImage
class.
Conclusion
Setting a window icon in Tkinter is a relatively simple yet impactful way to enhance your application’s user interface. Whether you choose to use the iconbitmap
method for ICO files or the PhotoImage
class for PNG and GIF files, these techniques will help you create a more personalized and recognizable application. Additionally, the ability to change icons dynamically opens up new possibilities for user interaction and customization. With the knowledge gained from this tutorial, you are well-equipped to make your Tkinter applications stand out.
FAQ
-
How do I convert an image to .ico format?
You can use online converters or image editing software like GIMP or Photoshop to convert images to .ico format. -
Can I use SVG files as window icons in Tkinter?
No, Tkinter does not support SVG files directly. You will need to convert them to a supported format like PNG or ICO. -
What is the maximum size for window icons in Tkinter?
The recommended size for window icons is 16x16 pixels, but larger sizes can be used depending on the application. -
Can I set different icons for different operating systems?
Yes, you can check the operating system using Python’splatform
module and set icons accordingly. -
Is it necessary to use an icon for my Tkinter application?
While not mandatory, using an icon improves the user experience and helps in branding your application.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook