Java에서 HTTP 프록시 서버 만들기
Sheeraz Gul
2023년10월12일
Java
Java HTTPS
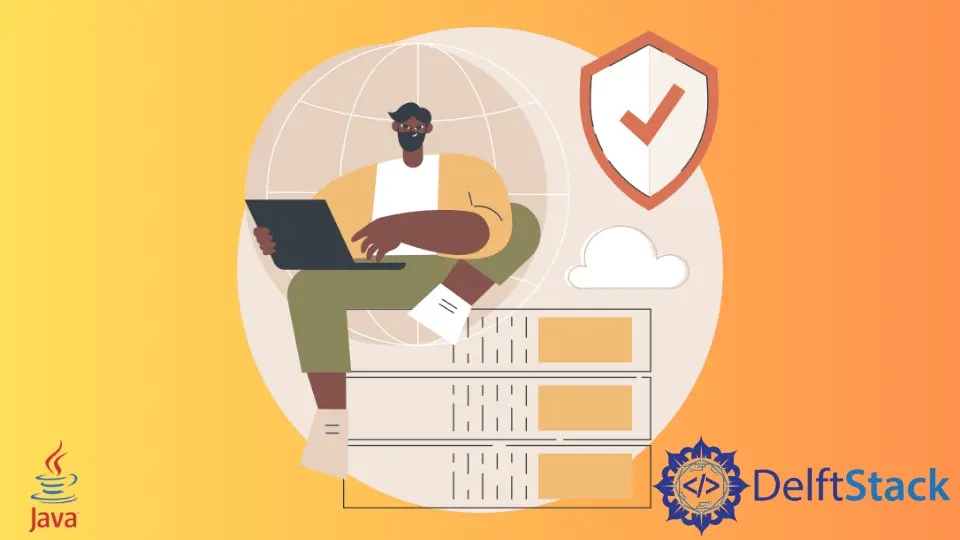
오늘날의 세계에서 프록시는 거의 모든 사람에게 필요합니다. 이 튜토리얼은 Java에서 HTTP 프록시 서버를 생성하는 방법을 보여줍니다.
Java에서 HTTP 프록시 서버 만들기
프록시 서버는 클라이언트와 서버 간의 중간 접근 방식입니다. 이 튜토리얼은 자바에서 HTTP 프록시 서버를 생성하는 방법을 보여줄 것입니다.
프록시 서버에는 다음과 같은 몇 가지 장점이 있습니다.
- Proxy Server를 사용하여 트래픽에 대한 통계를 생성할 수 있습니다.
- 프록시 서버를 사용하여 클라이언트의 콘텐츠를 업데이트할 수 있습니다.
- Proxy Server를 사용하여 문제가 있는 네트워크 시스템 반응을 분석할 수 있습니다.
- 프록시 서버를 사용하여 다운로드 및 업로드 제한을 제어할 수 있습니다.
- 프록시 서버를 사용하여 서버와 클라이언트 간의 트래픽을 캡처할 수 있습니다.
Java는 HTTP, FTP, HTTPS 등과 같은 다양한 프로토콜에 대한 프록시 처리기를 지원합니다. ProxyHost 및 ProxyPort는 Java에서 HTTP 프록시 서버에 사용됩니다.
Java에서 HTTP 프록시 서버를 구현해 보겠습니다.
package delftstack;
import java.io.*;
import java.net.*;
public class Proxy_Server {
public static void main(String[] args) throws IOException {
try {
String Proxy_Host = "Our Proxy Server";
int Remote_Port = 1025;
int Local_Port = 1026;
// Printing the start-up message
System.out.println(
"Starting proxy for " + Proxy_Host + ":" + Remote_Port + " on port " + Local_Port);
// start the server
Run_Server(Proxy_Host, Remote_Port, Local_Port);
} catch (Exception e) {
System.err.println(e);
}
}
public static void Run_Server(String Proxy_Host, int Remote_Port, int Local_Port)
throws IOException {
// Create a ServerSocket to listen connections
ServerSocket Server_Socket = new ServerSocket(Local_Port);
final byte[] Request = new byte[1024];
byte[] Reply = new byte[4096];
while (true) {
Socket Socket_Client = null, Socket_Server = null;
try {
// wait for a connection on the local port
Socket_Client = Server_Socket.accept();
final InputStream InputStreamClient = Socket_Client.getInputStream();
final OutputStream OutputStreamClient = Socket_Client.getOutputStream();
// Create the connection to the real server.
try {
Socket_Server = new Socket(Proxy_Host, Remote_Port);
} catch (IOException e) {
PrintWriter out = new PrintWriter(OutputStreamClient);
out.print("The Proxy Server could not connect to " + Proxy_Host + ":" + Remote_Port
+ ":\n" + e + "\n");
out.flush();
Socket_Client.close();
continue;
}
final InputStream InputStreamServer = Socket_Server.getInputStream();
final OutputStream OutputStreamServer = Socket_Server.getOutputStream();
// The thread to read the client's requests and to pass them
Thread New_Thread = new Thread() {
public void run() {
int Bytes_Read;
try {
while ((Bytes_Read = InputStreamClient.read(Request)) != -1) {
OutputStreamServer.write(Request, 0, Bytes_Read);
OutputStreamServer.flush();
}
} catch (IOException e) {
}
// Close the connections
try {
OutputStreamServer.close();
} catch (IOException e) {
}
}
};
// client-to-server request thread
New_Thread.start();
// Read server's responses and pass them to the client.
int Bytes_Read;
try {
while ((Bytes_Read = InputStreamServer.read(Reply)) != -1) {
OutputStreamClient.write(Reply, 0, Bytes_Read);
OutputStreamClient.flush();
}
} catch (IOException e) {
}
// Close the connection
OutputStreamClient.close();
} catch (IOException e) {
System.err.println(e);
} finally {
try {
if (Socket_Server != null)
Socket_Server.close();
if (Socket_Client != null)
Socket_Client.close();
} catch (IOException e) {
}
}
}
}
}
위의 코드는 Java로 HTTP 프록시 서버를 구현합니다. 성공적인 연결의 출력은 다음과 같습니다.
Starting proxy for Our Proxy Server:1025 on port 1026
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다
작가: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook