Crear un servidor proxy HTTP en Java
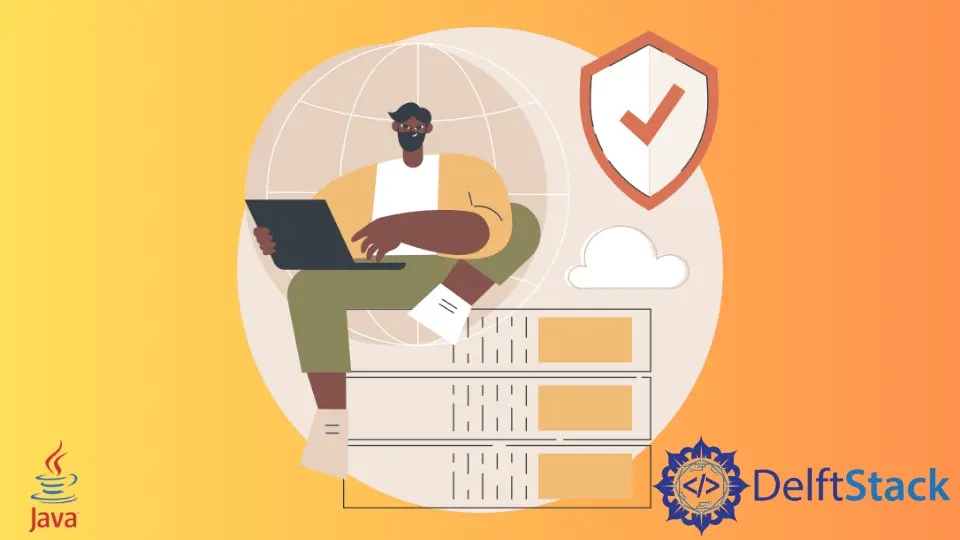
En el mundo de hoy, el proxy es la necesidad de casi todo el mundo. Este tutorial demuestra cómo crear un servidor proxy HTTP en Java.
Crear un servidor proxy HTTP en Java
Un servidor proxy es un enfoque intermedio entre el cliente y el servidor. Este tutorial demostrará un enfoque para crear un servidor proxy HTTP en Java.
Un servidor proxy tiene algunas ventajas, que incluyen:
- Con los Servidores Proxy, podemos crear estadísticas sobre el tráfico.
- Con Servidores Proxy, podemos actualizar el contenido del cliente.
- Podemos analizar la reacción del sistema de red de problemas con servidores proxy.
- Podemos usar servidores Proxy para controlar el límite de carga y descarga.
- Podemos usar servidores Proxy para capturar el tráfico entre el servidor y el cliente.
Java admite controladores de proxy para diferentes protocolos, por ejemplo, HTTP, FTP, HTTPS, etc. ProxyHost y ProxyPort se utilizan para un servidor proxy HTTP en Java.
Intentemos implementar un servidor proxy HTTP en Java:
package delftstack;
import java.io.*;
import java.net.*;
public class Proxy_Server {
public static void main(String[] args) throws IOException {
try {
String Proxy_Host = "Our Proxy Server";
int Remote_Port = 1025;
int Local_Port = 1026;
// Printing the start-up message
System.out.println(
"Starting proxy for " + Proxy_Host + ":" + Remote_Port + " on port " + Local_Port);
// start the server
Run_Server(Proxy_Host, Remote_Port, Local_Port);
} catch (Exception e) {
System.err.println(e);
}
}
public static void Run_Server(String Proxy_Host, int Remote_Port, int Local_Port)
throws IOException {
// Create a ServerSocket to listen connections
ServerSocket Server_Socket = new ServerSocket(Local_Port);
final byte[] Request = new byte[1024];
byte[] Reply = new byte[4096];
while (true) {
Socket Socket_Client = null, Socket_Server = null;
try {
// wait for a connection on the local port
Socket_Client = Server_Socket.accept();
final InputStream InputStreamClient = Socket_Client.getInputStream();
final OutputStream OutputStreamClient = Socket_Client.getOutputStream();
// Create the connection to the real server.
try {
Socket_Server = new Socket(Proxy_Host, Remote_Port);
} catch (IOException e) {
PrintWriter out = new PrintWriter(OutputStreamClient);
out.print("The Proxy Server could not connect to " + Proxy_Host + ":" + Remote_Port
+ ":\n" + e + "\n");
out.flush();
Socket_Client.close();
continue;
}
final InputStream InputStreamServer = Socket_Server.getInputStream();
final OutputStream OutputStreamServer = Socket_Server.getOutputStream();
// The thread to read the client's requests and to pass them
Thread New_Thread = new Thread() {
public void run() {
int Bytes_Read;
try {
while ((Bytes_Read = InputStreamClient.read(Request)) != -1) {
OutputStreamServer.write(Request, 0, Bytes_Read);
OutputStreamServer.flush();
}
} catch (IOException e) {
}
// Close the connections
try {
OutputStreamServer.close();
} catch (IOException e) {
}
}
};
// client-to-server request thread
New_Thread.start();
// Read server's responses and pass them to the client.
int Bytes_Read;
try {
while ((Bytes_Read = InputStreamServer.read(Reply)) != -1) {
OutputStreamClient.write(Reply, 0, Bytes_Read);
OutputStreamClient.flush();
}
} catch (IOException e) {
}
// Close the connection
OutputStreamClient.close();
} catch (IOException e) {
System.err.println(e);
} finally {
try {
if (Socket_Server != null)
Socket_Server.close();
if (Socket_Client != null)
Socket_Client.close();
} catch (IOException e) {
}
}
}
}
}
El código anterior implementa un servidor proxy HTTP en Java. La salida para una conexión exitosa es:
Starting proxy for Our Proxy Server:1025 on port 1026
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook