Go에서 객체 유형을 찾는 방법
Suraj Joshi
2024년2월16일
Go
Go Data Type
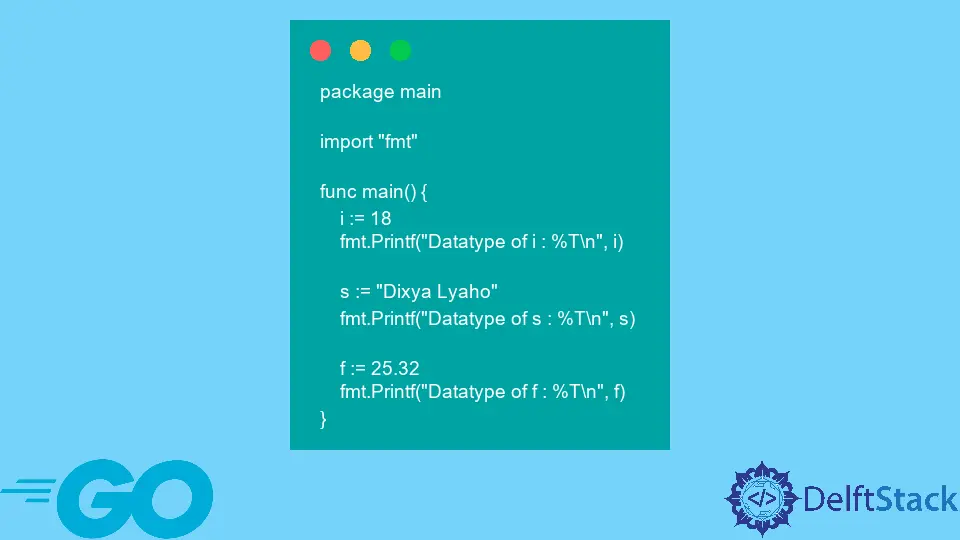
데이터 유형은 유효한 Go 변수와 연관된 유형을 지정합니다. Go에는 다음과 같은 네 가지 범주의 데이터 유형이 있습니다.
- 기본 유형 : 숫자, 문자열 및 부울
-
집계 유형 : 배열 및 구조체
-
참조 유형 : 포인터, 슬라이스, 맵, 기능 및 채널
-
인터페이스 유형
string 서식
,reflect
패키지 및type assertions
를 사용하여 Go에서 객체의 데이터 유형을 찾을 수 있습니다.
Go에서 데이터 유형을 찾기위한 문자열 형식화
특별한 포맷팅 옵션과 함께 패키지 fmt
의Printf
기능을 사용할 수 있습니다. fmt
를 사용하여 변수를 표시하는 데 사용할 수있는 형식화 옵션은 다음과 같습니다.
체재 | 기술 |
---|---|
%v |
변수 값을 기본 형식으로 인쇄 |
%+v |
값으로 필드 이름 추가 |
%#v |
값의 Go 구문 표현 |
%T |
값의 유형에 대한 Go 구문 표현 |
%% |
문자 퍼센트 부호; 가치가 없다 |
fmt
패키지의%T
플래그를 사용하여 Go에서 객체 유형을 찾습니다.
package main
import "fmt"
func main() {
i := 18
fmt.Printf("Datatype of i : %T\n", i)
s := "Dixya Lyaho"
fmt.Printf("Datatype of s : %T\n", s)
f := 25.32
fmt.Printf("Datatype of f : %T\n", f)
}
출력:
Datatype of i : int
Datatype of s : string
Datatype of f : float64
reflect
패키지
또한 reflect
패키지를 사용하여 객체의 데이터 유형을 찾을 수 있습니다. reflect
패키지의 Typeof
함수 는.String()
을 사용하여string
으로 변환 될 수있는 데이터 유형을 반환합니다.
package main
import (
"fmt"
"reflect"
)
func main() {
o1 := "string"
o2 := 10
o3 := 1.2
o4 := true
o5 := []string{"foo", "bar", "baz"}
o6 := map[string]int{"apple": 23, "tomato": 13}
fmt.Println(reflect.TypeOf(o1).String())
fmt.Println(reflect.TypeOf(o2).String())
fmt.Println(reflect.TypeOf(o3).String())
fmt.Println(reflect.TypeOf(o4).String())
fmt.Println(reflect.TypeOf(o5).String())
fmt.Println(reflect.TypeOf(o6).String())
}
출력:
string
int
float64
bool
[]string
map[string]int
type assertions
방법
형식 어설 션 메서드는 어설 션 작업의 성공 여부를 알려주는 부울 변수를 반환합니다. 우리는 switch
유형을 사용하여 객체의 데이터 유형을 찾기 위해 여러 유형의 assertion을 직렬로 수행합니다.
package main
import (
"fmt"
)
func main() {
var x interface{} = 3.85
switch x.(type) {
case int:
fmt.Println("x is of type int")
case float64:
fmt.Println("x is of type float64")
default:
fmt.Println("x is niether int nor float")
}
}
출력:
x is of type float64
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다
작가: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn