如何在 Go 中查找对象的类型
Suraj Joshi
2023年12月11日
Go
Go Data Type
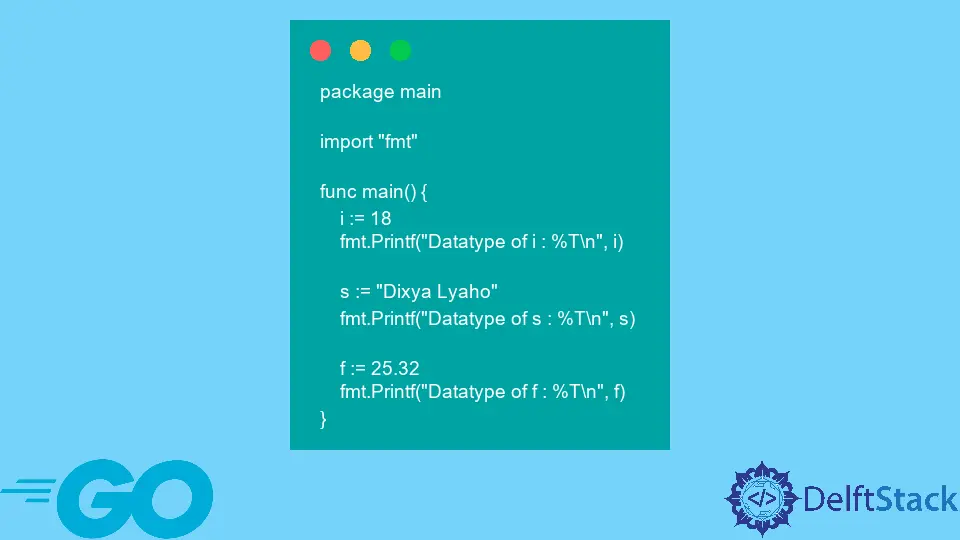
数据类型指定与有效 Go 变量关联的类型。Go 中有四类数据类型,如下所示:
- 基本类型:数字,字符串和布尔值
-
集合类型:数组和结构
-
参考类型:指针,切片,Map,函数和通道
-
接口类型
我们可以使用字符串格式,反射包和类型断言在 Go 中找到对象的数据类型。
在 Go 中字符串格式以查找数据类型
我们可以将软件包 fmt
的 Printf
功能与特殊格式设置一起使用。使用 fmt
显示变量的可用格式选项是:
格式 | 描述 |
---|---|
%v |
以默认格式打印变量值 |
%+v |
用值添加字段名称 |
%#v |
该值的 Go 语法表示形式 |
%T |
值类型的 Go 语法表示形式 |
%% |
文字百分号;不消耗任何价值 |
我们在 fmt
包中使用%T
标志来在 Go 语言中找到对象的类型。
package main
import "fmt"
func main() {
i := 18
fmt.Printf("Datatype of i : %T\n", i)
s := "Dixya Lyaho"
fmt.Printf("Datatype of s : %T\n", s)
f := 25.32
fmt.Printf("Datatype of f : %T\n", f)
}
输出:
Datatype of i : int
Datatype of s : string
Datatype of f : float64
Go reflect
包
我们也可以使用 reflect
包来查找对象的数据类型。reflect
包的 Typeof
函数返回值可以通过 .String()
转换为字符串数据类型。
package main
import (
"fmt"
"reflect"
)
func main() {
o1 := "string"
o2 := 10
o3 := 1.2
o4 := true
o5 := []string{"foo", "bar", "baz"}
o6 := map[string]int{"apple": 23, "tomato": 13}
fmt.Println(reflect.TypeOf(o1).String())
fmt.Println(reflect.TypeOf(o2).String())
fmt.Println(reflect.TypeOf(o3).String())
fmt.Println(reflect.TypeOf(o4).String())
fmt.Println(reflect.TypeOf(o5).String())
fmt.Println(reflect.TypeOf(o6).String())
}
输出:
string
int
float64
bool
[]string
map[string]int
类型断言方法
类型断言方法返回一个布尔变量,以判断断言操作是否成功。我们使用 switch
方法来串联执行几个类型断言,以找到对象的数据类型。
package main
import (
"fmt"
)
func main() {
var x interface{} = 3.85
switch x.(type) {
case int:
fmt.Println("x is of type int")
case float64:
fmt.Println("x is of type float64")
default:
fmt.Println("x is niether int nor float")
}
}
输出:
x is of type float64
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn