C#에서 문자열을 유형으로 변환
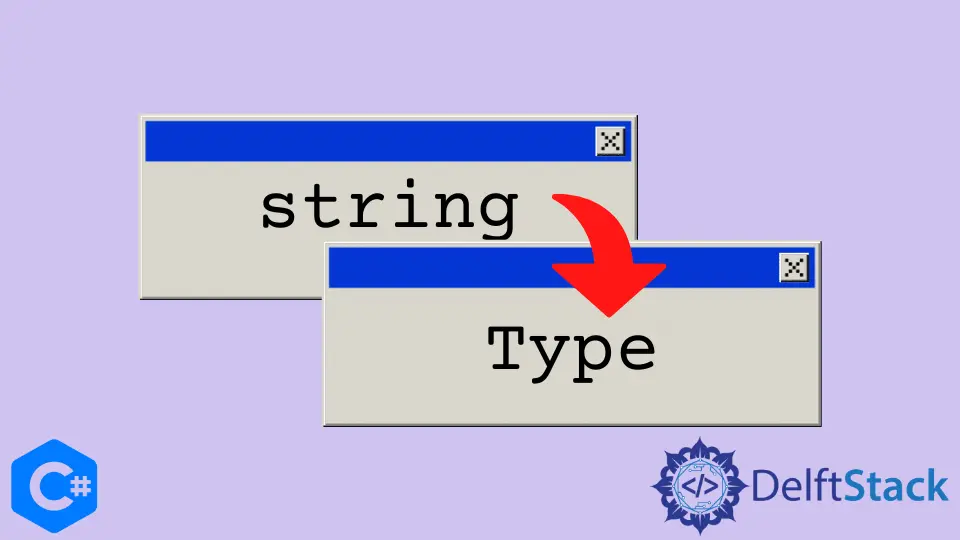
이 게시물에서는 문자열을 type
으로 변환하거나 C#에서 데이터 유형을 가져오는 방법을 설명합니다. 값 유형을 결정하기 위해 .GetType
함수를 사용합니다.
C#
에서 String
값의 type
가져오기
아래 예는 문자열의 runtime
유형 및 기타 값을 가져오고 각 값의 유형을 가져옵니다.
StringtoType
이라는 클래스와 Main()
메소드를 작성하십시오.
class StringtoType {
public static void Main() {}
}
다음으로 allvalues
라는 Object[]
유형 변수를 만들고 "Abc"
(문자열) 및 89
(바이트)와 같은 값을 지정합니다.
object[] allvalues = { "Abc",
(long)193527,
"Happy Programming",
(byte)89,
'Z',
(sbyte)-11,
"Zeelandia is 8th continent",
27.9,
"I am a string line",
(int)20,
'7' };
그런 다음 foreach
루프를 사용하여 배열의 각 항목을 확인하여 해당 유형을 확인합니다.
foreach (var data in allvalues) {
}
foreach
루프 내에서 유형의 Type
의 t
라는 변수를 초기화합니다. 변수 t
는 data.GetType()
메소드의 도움으로 allvalues
배열에 있는 모든 값의 데이터 유형을 보유합니다.
Type t = data.GetType();
그런 다음 if
조건을 적용하여 모든 값이 문자열인지 확인합니다.
if (t.Equals(typeof(string)))
값이 문자열인 경우 아래와 같은 메시지가 표시됩니다.
'Happy Programming' is a String
문자열을 Type
으로 변환하고 else if
검사를 통해 byte
, sbyte
, int
및 double
등과 같은 다른 유형의 데이터:
else if (t.Equals(typeof(sbyte))) Console.WriteLine(" '{0}' is a Signed Byte", data);
else if (t.Equals(typeof(byte))) Console.WriteLine(" '{0}' is a Byte", data);
else if (t.Equals(typeof(int))) Console.WriteLine(" '{0}' is an Integer of 32-bit", data);
else if (t.Equals(typeof(long))) Console.WriteLine(" '{0}' is an Integer of 64-bit", data);
else if (t.Equals(typeof(double))) Console.WriteLine("'{0}' is a double ", data);
마지막으로 allvalues
배열에 byte
, sbyte
, int
, double
및 long
유형의 데이터가 아닌 다른 유형이 있는 경우 다음과 같은 메시지가 표시됩니다.
'Z' is another type of data
전체 코드 예:
using System;
using System.Diagnostics;
class StringtoType {
public static void Main() {
object[] allvalues = { "Abc",
(long)193527,
"Happy Programming",
(byte)89,
'Z',
(sbyte)-11,
"Zeelandia is 8th continent",
27.9,
"I am a string line",
(int)20,
'7' };
foreach (var data in allvalues) {
Type t = data.GetType();
if (t.Equals(typeof(string)))
Console.WriteLine(" '{0}' is a String", data);
else if (t.Equals(typeof(sbyte)))
Console.WriteLine(" '{0}' is a Signed Byte", data);
else if (t.Equals(typeof(byte)))
Console.WriteLine(" '{0}' is a Byte", data);
else if (t.Equals(typeof(int)))
Console.WriteLine(" '{0}' is an Integer of 32-bit", data);
else if (t.Equals(typeof(long)))
Console.WriteLine(" '{0}' is an Integer of 64-bit", data);
else if (t.Equals(typeof(double)))
Console.WriteLine("'{0}' is a double", data);
else
Console.WriteLine("'{0}' is another type of data", data);
}
}
}
출력:
'Abc' is a String
'193527' is an Integer of 64-bit
'Happy Programming' is a String
'89' is a Byte
'Z' is another type of data
'-11' is a Signed Byte
'Zeelandia is 8th continent' is a String
'27.9' is a double
'I am a string line' is a String
'20' is an Integer of 32-bit
'7' is another type of data
C#
의 type
개체 비교
유형이 있는 Type
개체는 고유합니다. 두 개의 Type
개체 식별자는 동일한 유형을 나타내는 경우 동일한 개체에 해당합니다.
참조 동등성을 사용하여 Type
개체를 비교할 수 있습니다. 아래 예는 여러 정수 값을 포함하는 Type
개체를 비교하여 동일한 유형인지 확인합니다.
먼저 Main()
메소드 내에서 int
, double
, long
유형의 변수를 초기화하고 일부 값을 할당합니다.
int Value1 = 2723;
double Value2 = 123.56;
int Value3 = 1747;
long Value4 = 123456789;
.GetType
메소드의 도움으로 Value1
이라는 값 1의 데이터 유형을 저장합니다.
Type t = Value1.GetType();
이제 Object.ReferenceEquals(t, Value2.GetType())
함수의 도움으로 Value1
을 사용하여 모든 개체의 유형을 비교할 것입니다.
Console.WriteLine("Data type of Value1 and Value2 are equal: {0}",
Object.ReferenceEquals(t, Value2.GetType()));
Console.WriteLine("Data type of Value1 and Value3 are equal: {0}",
Object.ReferenceEquals(t, Value3.GetType()));
Console.WriteLine("Data type of Value1 and Value4 are equal: {0}",
Object.ReferenceEquals(t, Value4.GetType()));
전체 코드 예:
using System;
using System.Diagnostics;
class CompareTypeObjects {
public static void Main() {
int Value1 = 2723;
double Value2 = 123.56;
int Value3 = 1747;
long Value4 = 123456789;
Type t = Value1.GetType();
Console.WriteLine("The data type of Value1 and Value2 are equal: {0}",
Object.ReferenceEquals(t, Value2.GetType()));
Console.WriteLine("The data type of Value1 and Value3 are equal: {0}",
Object.ReferenceEquals(t, Value3.GetType()));
Console.WriteLine("The data type of Value1 and Value4 are equal: {0}",
Object.ReferenceEquals(t, Value4.GetType()));
}
}
출력:
The data type of Value1 and Value2 are equal: False
The data type of Value1 and Value3 are equal: True
The data type of Value1 and Value4 are equal: False
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn