Convertir cadena a tipo en C#
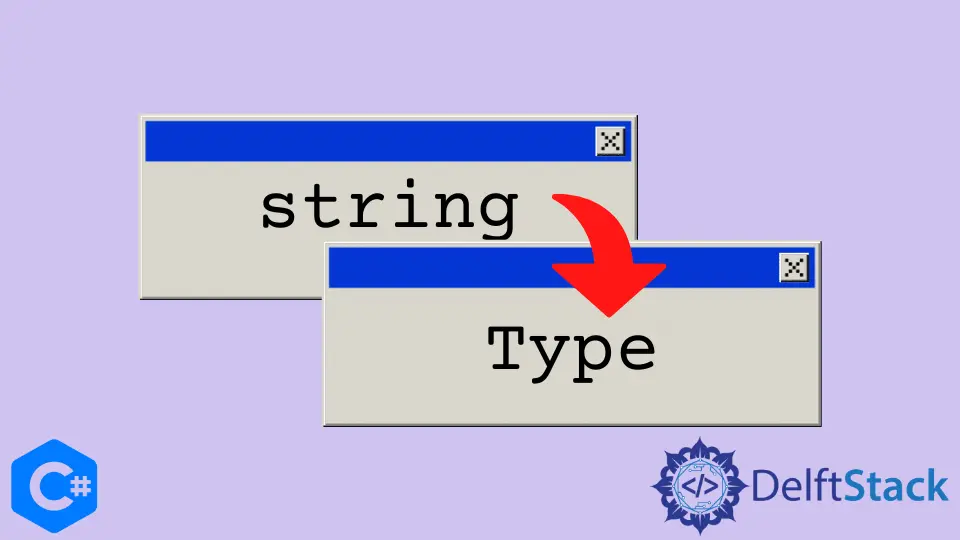
En esta publicación, explicaré cómo convertir una cadena a tipo
u obtener el tipo de datos en C#. Para determinar el tipo de valor, utilizaremos la función .GetType
.
Obtenga el tipo
del valor String
en C#
El siguiente ejemplo obtiene el tipo runtime
de una cadena y otros valores y procede a obtener el tipo de cada valor.
Cree una clase llamada StringtoType
y un método Main()
.
class StringtoType {
public static void Main() {}
}
A continuación, crea una variable de tipo Object[]
llamada allvalues
y dale algunos valores como "Abc"
(como una cadena) y 89
(como un byte).
object[] allvalues = { "Abc",
(long)193527,
"Happy Programming",
(byte)89,
'Z',
(sbyte)-11,
"Zeelandia is 8th continent",
27.9,
"I am a string line",
(int)20,
'7' };
Luego, utilizando un bucle foreach
, verifique cada entrada en el array para determinar su tipo.
foreach (var data in allvalues) {
}
Inicialice una variable llamada t
de Type
de tipo dentro del bucle foreach
. La variable t
contendrá el tipo de datos de cada valor presente en el array allvalues
con la ayuda del método data.GetType()
.
Type t = data.GetType();
Después de eso, aplicaremos la condición if
para verificar si cada valor será una cadena.
if (t.Equals(typeof(string)))
Si se encuentra que un valor es una cadena, se mostrará un mensaje como el siguiente.
'Happy Programming' is a String
Después de convertir una cadena a Type
, y otros tipos de datos como byte
, sbyte
, int
y double
, etc. con la ayuda de las siguientes comprobaciones else if
:
else if (t.Equals(typeof(sbyte))) Console.WriteLine(" '{0}' is a Signed Byte", data);
else if (t.Equals(typeof(byte))) Console.WriteLine(" '{0}' is a Byte", data);
else if (t.Equals(typeof(int))) Console.WriteLine(" '{0}' is an Integer of 32-bit", data);
else if (t.Equals(typeof(long))) Console.WriteLine(" '{0}' is an Integer of 64-bit", data);
else if (t.Equals(typeof(double))) Console.WriteLine("'{0}' is a double ", data);
Finalmente, si se encuentran otros tipos de datos que no sean byte
, sbyte
, int
, double
y long
en el array allvalues
, simplemente mostrará un mensaje como este:
'Z' is another type of data
Ejemplo de código completo:
using System;
using System.Diagnostics;
class StringtoType {
public static void Main() {
object[] allvalues = { "Abc",
(long)193527,
"Happy Programming",
(byte)89,
'Z',
(sbyte)-11,
"Zeelandia is 8th continent",
27.9,
"I am a string line",
(int)20,
'7' };
foreach (var data in allvalues) {
Type t = data.GetType();
if (t.Equals(typeof(string)))
Console.WriteLine(" '{0}' is a String", data);
else if (t.Equals(typeof(sbyte)))
Console.WriteLine(" '{0}' is a Signed Byte", data);
else if (t.Equals(typeof(byte)))
Console.WriteLine(" '{0}' is a Byte", data);
else if (t.Equals(typeof(int)))
Console.WriteLine(" '{0}' is an Integer of 32-bit", data);
else if (t.Equals(typeof(long)))
Console.WriteLine(" '{0}' is an Integer of 64-bit", data);
else if (t.Equals(typeof(double)))
Console.WriteLine("'{0}' is a double", data);
else
Console.WriteLine("'{0}' is another type of data", data);
}
}
}
Producción :
'Abc' is a String
'193527' is an Integer of 64-bit
'Happy Programming' is a String
'89' is a Byte
'Z' is another type of data
'-11' is a Signed Byte
'Zeelandia is 8th continent' is a String
'27.9' is a double
'I am a string line' is a String
'20' is an Integer of 32-bit
'7' is another type of data
Comparar objetos type
en C#
Un objeto Type
con un tipo es distinto. Dos identificadores de objeto Type
corresponden al mismo objeto si representan el mismo tipo.
Permite comparar objetos Type
utilizando la igualdad de referencia. El siguiente ejemplo compara objetos Type
que contienen varios valores enteros para ver si son del mismo tipo.
Al principio, inicialice los tipos de variables int
, double
y long
dentro del método Main()
, y asígneles algunos valores.
int Value1 = 2723;
double Value2 = 123.56;
int Value3 = 1747;
long Value4 = 123456789;
Inicialice una variable llamada t
de tipo Type
en la variable t
almacenaremos el tipo de datos de valor 1 llamado Value1
con la ayuda del método .GetType
.
Type t = Value1.GetType();
Ahora, con la ayuda de la función Object.ReferenceEquals(t, Value2.GetType())
, compararemos los tipos de todos los objetos con Value1
.
Console.WriteLine("Data type of Value1 and Value2 are equal: {0}",
Object.ReferenceEquals(t, Value2.GetType()));
Console.WriteLine("Data type of Value1 and Value3 are equal: {0}",
Object.ReferenceEquals(t, Value3.GetType()));
Console.WriteLine("Data type of Value1 and Value4 are equal: {0}",
Object.ReferenceEquals(t, Value4.GetType()));
Ejemplo de código completo:
using System;
using System.Diagnostics;
class CompareTypeObjects {
public static void Main() {
int Value1 = 2723;
double Value2 = 123.56;
int Value3 = 1747;
long Value4 = 123456789;
Type t = Value1.GetType();
Console.WriteLine("The data type of Value1 and Value2 are equal: {0}",
Object.ReferenceEquals(t, Value2.GetType()));
Console.WriteLine("The data type of Value1 and Value3 are equal: {0}",
Object.ReferenceEquals(t, Value3.GetType()));
Console.WriteLine("The data type of Value1 and Value4 are equal: {0}",
Object.ReferenceEquals(t, Value4.GetType()));
}
}
Producción :
The data type of Value1 and Value2 are equal: False
The data type of Value1 and Value3 are equal: True
The data type of Value1 and Value4 are equal: False
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn