C#에서 알파벳 순서로 목록 정렬
Haider Ali
2023년10월12일
Csharp
Csharp List
Csharp Sort
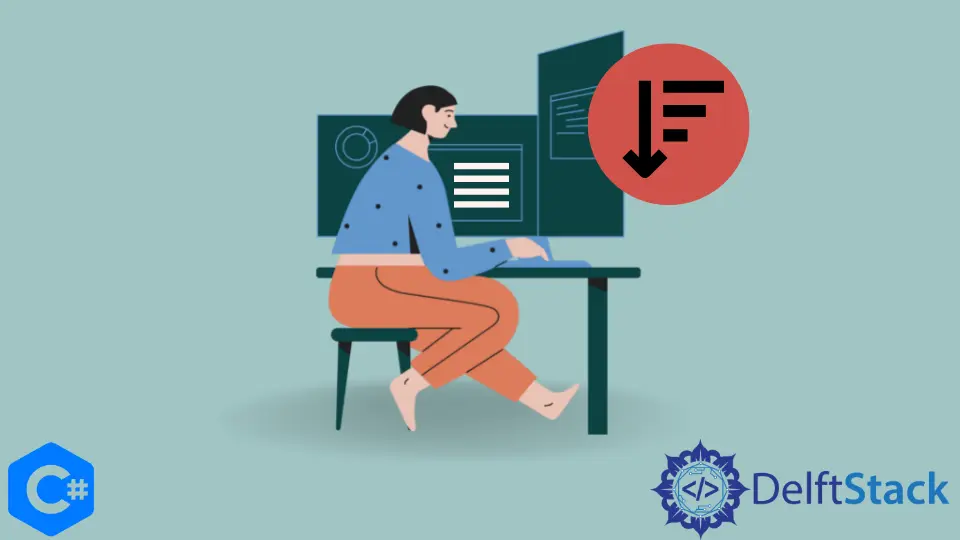
이 가이드는 C#에서 알파벳 순서로 단어를 정렬하는 방법을 보여줍니다. C#에는 목록을 정렬하는 데 사용할 수 있는 내장 함수가 있습니다.
Sort()
메서드를 사용하여 C#
에서 알파벳 순서로 목록 정렬
먼저 using System.Collections.Generic;
, 이것은 C#에서 목록을 사용하기 위해 가져와야 하는 라이브러리입니다. 목록을 정렬하려면 Sort()
를 사용해야 합니다.
그런 다음 비교기를 사용하여 두 문자열을 비교해야 합니다. 예를 들어 다음 코드를 살펴보십시오.
citizens.Sort((x, y) => string.Compare(x.Name, y.Name));
위의 코드 줄에서 citizens
는 목록이고 우리는 시민 목록을 알파벳 순서로 정렬하기 위해 시민의 이름을 비교합니다.
foreach
루프를 사용하여 C#
에서 알파벳순으로 목록을 인쇄합니다
using System;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Collections.Generic; // library import to use list;
namespace list_sort {
class Program {
static void Main(string[] args) {
List<Person> citizens = new List<Person>(5); // Creating List Of Person having size 5;
// Adding Persons in List
citizens.Add(new Person("Mark", "Zuker", "Silicon Valley United States", 50));
citizens.Add(new Person("Bill ", "Gates", "Silicon Valley United States", 70));
citizens.Add(new Person("Jeff", "Bezoz", "Silicon Valley United States", 40));
citizens.Add(new Person("Elon", "Musk", "Silicon Valley United States", 20));
citizens.Add(new Person("Antony", "Gates", "Silicon Valley United States", 30));
Console.WriteLine(":::::::::::::::::::::::::::::::Before Sorting ::::::::::::::::::::::::");
// Prinring The List Names...
foreach (Person p in citizens) { // Loop through List with foreach
Console.WriteLine(p.Name);
}
Console.WriteLine(":::::::::::::::::::::::::::::::After Sorting :::::::::::::::::");
// Problem # Sort List of Citizens According to Citizen Names orderby-alphabetical-order
citizens.Sort((x, y) => string.Compare(x.Name, y.Name));
foreach (Person p in citizens) // Prniting After alphabetical Sort.
{ // Loop through List with foreach
Console.WriteLine(p.Name);
}
Console.ReadKey(); // to Stay On Screen.
}
}
class Person {
public String Name;
public String LastName;
public String Address;
public int age;
public Person(String Name, String LastName, String Address, int Age) {
this.Name = Name;
this.LastName = LastName;
this.Address = Address;
this.age = Age;
}
}
}
먼저 citizens
목록을 만든 다음 이름, 주소 및 나이를 추가했습니다. 목록을 정렬하기 전에 인쇄한 다음 목록을 정렬한 후에 인쇄했습니다.
출력:
:::::::::::::::::::::::::::::::Before Sorting ::::::::::::::::::::::::
Mark
Bill
Jeff
Elon
Antony
:::::::::::::::::::::::::::::::After Sorting :::::::::::::::::
Antony
Bill
Elon
Jeff
Mark
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다
작가: Haider Ali
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn관련 문장 - Csharp List
- IEnumerable을 C#의 목록으로 변환하는 방법
- C# 목록에서 항목 제거
- C# 두 목록을 함께 결합
- C#에 나열할 고유 항목
- C#에서 값을 사용하여 목록 초기화
- C#에서 내림차순으로 목록 정렬