C++에서 문자열 정렬
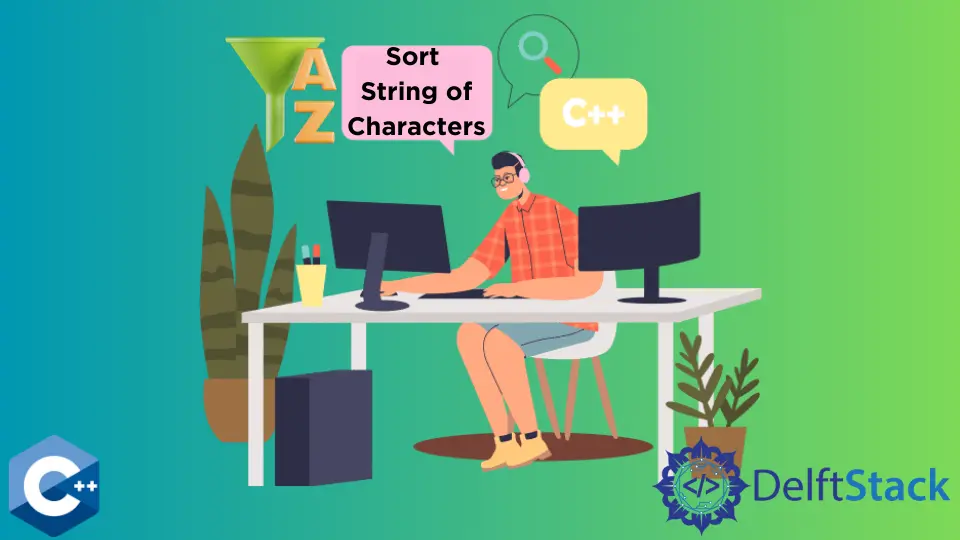
이 가이드에서는 C++에서 문자열을 정렬하는 방법에 대한 몇 가지 방법을 설명합니다.
std::sort
알고리즘을 사용하여 C++에서 문자열 정렬
이 기사에서는 문자 시퀀스가std::string
객체에 저장되어 있다고 가정합니다. std::string
클래스 객체는 반복 가능하므로 범위 기반 STL 함수를 호출 할 수 있습니다. 이 경우 각 문자열에서 STL 알고리즘의std::sort
함수를 사용합니다. 여기서는std::sort
함수의 가장 간단한 오버로드를 사용합니다.이 함수는 두 개의 반복기 인수를 사용하여 범위를 탐색하고 기본적으로 비 내림차순으로 요소를 정렬합니다.
#include <algorithm>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::sort;
using std::string;
using std::vector;
template <typename T>
void printVector(vector<T> &vec) {
for (const auto &item : vec) {
cout << item << "; " << endl;
}
cout << endl;
}
int main() {
vector<string> vec1 = {"algorithms library", "occurrences",
"implementation-specific", "contribute",
"specialization"};
for (auto &item : vec1) {
sort(item.begin(), item.end());
}
printVector(vec1);
return EXIT_SUCCESS;
}
출력:
aabghiillmorrrsty;
ccceenorrsu;
-acceeefiiiilmmnnoppstt;
bceinorttu;
aaceiiilnopstz;
또는 사용자 정의 비교기 함수를std::sort
알고리즘에 전달하여 그에 따라 요소를 정렬 할 수 있습니다. 함수 프로토 타입은bool cmp(const Type1 &a, const Type2 &b);
형식이어야합니다. 다음 예제 코드에서는 람다 식을 사용하여 정렬 순서를 내림차순으로 반전합니다.
#include <algorithm>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::sort;
using std::string;
using std::vector;
template <typename T>
void printVector(vector<T> &vec) {
for (const auto &item : vec) {
cout << item << "; " << endl;
}
cout << endl;
}
int main() {
vector<string> vec1 = {"algorithms library", "occurrences",
"implementation-specific", "contribute",
"specialization"};
for (auto &item : vec1) {
sort(item.begin(), item.end(), [](auto &c1, auto &c2) { return c1 > c2; });
}
printVector(vec1);
return EXIT_SUCCESS;
}
출력:
ytsrrromlliihgbaa ;
usrroneeccc;
ttspponnmmliiiifeeecca-;
uttroniecb;
ztsponliiiecaa;
사용자 지정 함수 래퍼를 사용하여 C++에서 문자열 정렬
이전 솔루션의 눈에 띄는 결함 중 하나는 문자의 구두점과 간격을 유효한 영숫자 문자와 구별 할 수 없다는 것입니다. 따라서 주어진string
객체에서 모든 구두점 및 공백 문자를 삭제 한 다음std::sort
알고리즘을 호출하여 정렬 작업을 수행하는 별도의 함수를 구현할 수 있습니다. 제거 작업은erase-remove_if
관용구를 사용하여 수행됩니다.이 관용구는 람다 식을 사용하여 각 문자의 유형을 확인합니다. isspace
및ispunct
기능은<locale>
헤더 파일에 포함되어 사용됩니다.
#include <algorithm>
#include <iostream>
#include <locale>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::sort;
using std::string;
using std::vector;
template <typename T>
void printVector(vector<T> &vec) {
for (const auto &item : vec) {
cout << item << "; " << endl;
}
cout << endl;
}
void sortStringChars(string &s) {
s.erase(std::remove_if(
s.begin(), s.end(),
[](auto &c) { return std::isspace(c) || std::ispunct(c); }),
s.end());
sort(s.begin(), s.end());
}
int main() {
vector<string> vec1 = {"algorithms library", "occurrences",
"implementation-specific", "contribute",
"specialization"};
for (auto &item : vec1) {
sortStringChars(item);
}
printVector(vec1);
return EXIT_SUCCESS;
}
출력:
aabghiillmorrrsty;
ccceenorrsu;
acceeefiiiilmmnnoppstt;
bceinorttu;
aaceiiilnopstz;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook