C++에서 문자열을 반전하는 방법
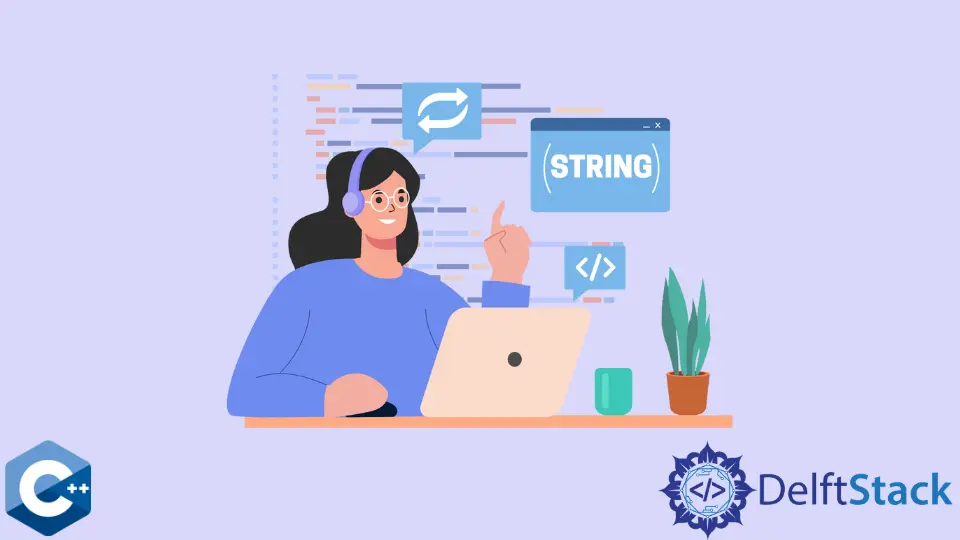
이 기사에서는 C++에서 문자열을 뒤집는 방법을 설명합니다.
문자열 생성자를 사용하여 문자열 반전
std::basic_string
에는 범위의 내용으로 문자열을 만들 수있는 생성자가 있습니다. 그런 다음 새 문자열 변수를 선언하고 생성자에게 원래 문자열 변수tmp_s
의 역 반복자를 제공 할 수 있습니다. 다음 예제는이 방법을 보여주고 확인을 위해 두 문자열을 모두 출력합니다.
#include <algorithm>
#include <iostream>
#include <string>
using std::cout;
using std::endl;
using std::reverse;
using std::string;
int main() {
string tmp_s = "This string will be reversed";
cout << tmp_s << endl;
string tmp_s_reversed(tmp_s.rbegin(), tmp_s.rend());
cout << tmp_s_reversed << endl;
return EXIT_SUCCESS;
}
출력:
This string will be reversed
desrever eb lliw gnirts sihT
std::reverse()
알고리즘을 사용하여 문자열 반전
std::reverse
메소드는<algorithm>
STL 라이브러리에서 가져 오며 범위에있는 요소의 순서를 반대로합니다. 이 메서드는 인수로 전달 된 객체에서 작동하며 데이터의 새 복사본을 반환하지 않으므로 원래 문자열을 유지하려면 다른 변수를 선언해야합니다.
알고리즘이 메모리 할당에 실패하면reverse
함수는std::bad_alloc
예외를 발생시킵니다.
#include <algorithm>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::reverse;
using std::string;
int main() {
string tmp_s = "This string will be reversed";
cout << tmp_s << endl;
string tmp_s_reversed(tmp_s);
reverse(tmp_s_reversed.begin(), tmp_s_reversed.end());
cout << tmp_s_reversed << endl;
return EXIT_SUCCESS;
}
std::copy()
알고리즘을 사용하여 문자열 반전
std::copy
는 여러 시나리오에 사용할 수있는 또 다른 강력한 알고리즘입니다. 이 솔루션은 새 문자열 변수를 초기화하고 내장resize
메소드를 사용하여 크기를 수정합니다. 다음으로copy
메소드를 호출하여 선언 된 문자열을 원래 문자열의 데이터로 채 웁니다. 하지만 처음 두 매개 변수는 소스 범위의 역방향 반복자 여야합니다.
#include <algorithm>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::reverse;
using std::string;
int main() {
string tmp_s = "This string will be reversed";
cout << tmp_s << endl;
string tmp_s_reversed;
tmp_s_reversed.resize(tmp_s.size());
copy(tmp_s.rbegin(), tmp_s.rend(), tmp_s_reversed.begin());
cout << tmp_s_reversed << endl;
return EXIT_SUCCESS;
}
반전 된 문자열 데이터를 저장할 필요가없는 경우copy()
알고리즘을 사용하여 다음 코드 샘플과 같이 문자열 데이터를 역순으로 콘솔에 직접 출력 할 수 있습니다.
#include <algorithm>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::reverse;
using std::string;
int main() {
string tmp_s = "This string will be reversed";
cout << tmp_s << endl;
copy(tmp_s.rbegin(), tmp_s.rend(), std::ostream_iterator<char>(cout, ""));
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook