C++에서 goto 문 활용
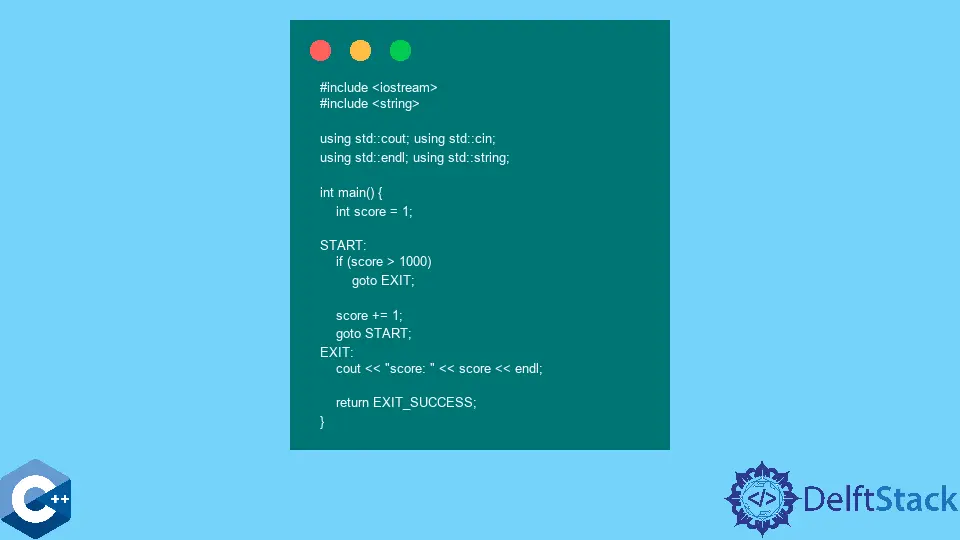
이 기사에서는 C++에서goto
문을 활용하는 방법을 소개합니다.
goto
문 사용 C++에서 프로그램 제어 흐름 점프 구현
goto
문은 프로그램 제어 흐름이 레이블이 가리키는 행으로 이동하도록합니다. 일반적으로 C++의 모든 명령문은 식별자 뒤에 콜론이 오는 특수 표기법을 사용하여 레이블을 지정할 수 있습니다. 일반적으로 이러한 레이블 식별자는 함수 범위의 모든 곳에서 사용할 수 있습니다. 따라서 레이블 앞에있는goto
문으로 참조 할 수 있습니다. 이전 범위 규칙은 변수에 대한 규칙과 다릅니다. 즉, 다른 문에서 사용하기 전에 변수를 선언해야합니다.
그러나goto
는 범위로 앞으로 이동할 수없고 이니셜 라이저, 사소하지 않은 생성자 / 소멸자가 있거나 스칼라 유형이 아닌 변수 선언문을 건너 뛸 수 없습니다. 그렇지 않으면 컴파일러 오류가 발생할 것으로 예상됩니다. 다음 예에서는 텍스트에서 주어진 문자열의 첫 번째 모양을 검색하고, 발견되면goto
문으로 루프를 끊고 프로그램 끝에서 제어를 이동합니다. 결과적으로 실패 메시지를 나타내는cout
문은 건너 뛰고 사용자가 전달한 문자열 값과 일치하는 문자열이 없을 때만 실행됩니다.
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
string text(
"Lorem ipsum dolor sit amet, consectetur adipiscing elit."
" Nam mollis felis eget diam fermentum, sed consequat justo pulvinar. ");
string search_query;
cout << "Enter a string to search: ";
cin >> search_query;
std::istringstream iss(text);
string word;
while (iss >> word) {
if (word == search_query) {
cout << "'" << search_query << "' - found in text" << endl;
goto END;
}
}
cout << "Could not find such string!" << endl;
END:
return EXIT_SUCCESS;
}
또는 오류 방지 동작 측면에서 코드에 더 견고성을 추가하는 사용자 입력 유효성 검사 기능을 사용하여 이전 예제를 수행 할 수 있습니다. cin
멤버 함수는 입력 검사 기능을 구현하고 읽은 데이터에 대한 참조를 반환하는 데 사용됩니다.
#include <iostream>
#include <limits>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
template <typename T>
T &validateInput(T &val) {
while (true) {
cout << "Enter a string to search: ";
if (cin >> val) {
break;
} else {
if (cin.eof()) exit(EXIT_SUCCESS);
cout << "Enter a string to search:\n";
cin.clear();
cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n');
}
}
return val;
}
int main() {
string text(
"Lorem ipsum dolor sit amet, consectetur adipiscing elit."
" Nam mollis felis eget diam fermentum, sed consequat justo pulvinar. ");
string search_query;
validateInput(search_query);
std::istringstream iss(text);
string word;
while (iss >> word) {
if (word == search_query) {
cout << "'" << search_query << "' - found in text" << endl;
goto END;
}
}
cout << "Could not find such string!" << endl;
END:
return EXIT_SUCCESS;
}
goto
문을 사용하여 C++에서 루프 스타일 반복 구현
goto
문을 사용하여 루프와 유사한 동작을 구현할 수 있습니다. 여기서if
조건은 각 반복을 평가하고 제어가 루프에서 종료되는지 여부를 결정합니다. START
및END
라는 이름으로 루프 본문에 레이블을 지정했습니다. 기본적으로 반복은1000
보다 클 때까지score
변수를 증가시킵니다. 따라서 값이1001
을 평가할 때 반복이 중단되고 그 지점에서 프로그램은cout
문이 호출되는 행으로 이동합니다.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
int score = 1;
START:
if (score > 1000) goto EXIT;
score += 1;
goto START;
EXIT:
cout << "score: " << score << endl;
return EXIT_SUCCESS;
}
출력:
score: 1001
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook