C++ Switch 문의 중단
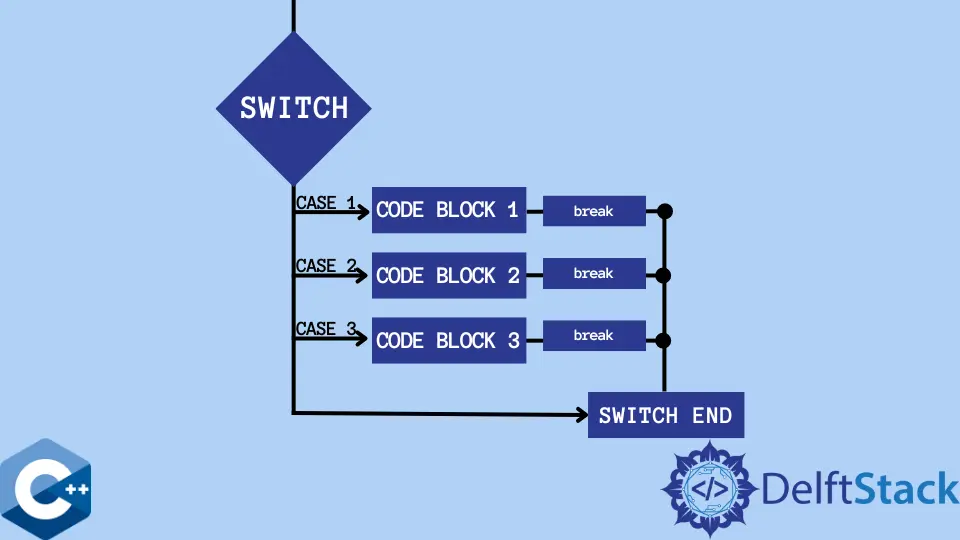
C 및 C++의 break
문은 switch
문의 코드 블록 내에서 필요한 조건이 충족되는 경우 루프가 반복되는 것을 중지하는 데 사용됩니다.
break
문을 사용하지 않으면 switch
문의 끝에 도달할 때까지 프로그램이 계속 실행됩니다.
먼저 switch
문이 어떻게 작동하는지 이해해야 합니다.
switch
문의 시작 부분에 표현식을 제공해야 합니다. 그러면 프로그램은 switch
문의 각 case
를 살펴보고 우리가 제공한 표현식과 일치하는 경우를 찾으면 다음을 수행합니다. 그 케이스
를 실행하십시오.
위의 프로세스가 표현식과 일치하지 않으면 프로그램은 switch
문에서 벗어나 default
문을 실행합니다.
C++에서 break
가 있는 switch
문
#include <iostream>
using namespace std;
int main() {
int rating = 2;
switch (rating) {
case 1:
cout << "Rated as 1. ";
break;
case 2:
cout << "Rated as 2. ";
break;
case 3:
cout << "Rated as 3. ";
break;
case 4:
cout << "Rated as 4. ";
break;
case 5:
cout << "Rated as 5. ";
break;
}
return 0;
}
여기 표현은 rating = 2
입니다. 여기서 프로그램이 하는 일은 각 case
를 하나씩 살펴보고 루프 실행을 더 이상 중지하고 종료하기 위해 제공된 표현식에 대한 잠재적 일치를 확인하여 아래 출력을 제공하는 것입니다.
Rated as 2.
C++에서 break
가 없는 switch
문
동일한 코드를 다시 실행해 보겠습니다. 하지만 이번에는 각 사례 다음에 break
문을 제거하여 실행합니다.
#include <iostream>
using namespace std;
int main() {
int rating = 2;
switch (rating) {
case 1:
cout << "Rated as 1. ";
case 2:
cout << "Rated as 2. ";
case 3:
cout << "Rated as 3. ";
case 4:
cout << "Rated as 4. ";
case 5:
cout << "Rated as 5. ";
}
return 0;
}
출력:
Rated as 2. Rated as 3. Rated as 4. Rated as 5.
break
문이 없으면 요구 사항이 충족된 후에도 프로그램이 각 case
값을 인쇄하는 것을 볼 수 있습니다.
break
문은 루프가 반복되어야 하는 횟수를 알 수 없거나 루프가 미리 정의된 특정 조건을 충족하는 상황에 사용됩니다.
switch
명령문 본문은 default
명령문이 없고 case
일치 항목이 명령문 본문에 없는 경우 실행되지 않습니다. switch
문 본문 내부에는 하나의 default
문만 있을 수 있습니다.
마지막으로 break
문은 doing, for 및 while 루프에도 사용됩니다. 이러한 상황에서 break
문은 특정 기준이 충족될 때 프로그램이 루프를 종료하도록 합니다.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.