C++ Switch 語句中的中斷
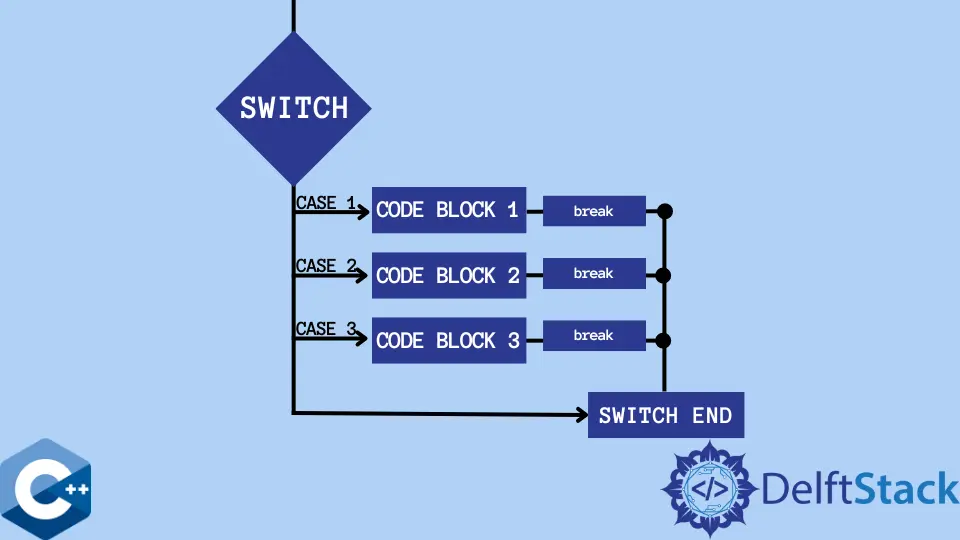
C 和 C++ 中的 break
語句用於在 switch
語句的程式碼塊中滿足所需條件時停止迴圈迭代。
如果沒有使用 break
語句,程式將繼續執行,直到到達 switch
語句的末尾。
首先,我們必須瞭解 switch
語句的工作原理。
在 switch
語句的開頭,我們必須提供一個表示式,然後程式將遍歷 switch
語句中的每個 case
,如果找到與我們提供的表示式匹配的 case,它將執行那個 case
。
如果上述過程不能匹配表示式,程式會跳出 switch
語句並執行 default
語句。
C++ 中帶有 break
的 switch
語句
#include <iostream>
using namespace std;
int main() {
int rating = 2;
switch (rating) {
case 1:
cout << "Rated as 1. ";
break;
case 2:
cout << "Rated as 2. ";
break;
case 3:
cout << "Rated as 3. ";
break;
case 4:
cout << "Rated as 4. ";
break;
case 5:
cout << "Rated as 5. ";
break;
}
return 0;
}
這裡的表示式是 rating = 2
。程式在這裡所做的是它一個接一個地遍歷每個 case
並檢查提供的表示式的潛在匹配以停止進一步執行迴圈並終止它,給我們下面的輸出。
Rated as 2.
C++ 中沒有 break
的 switch
語句
讓我們再次執行相同的程式碼,但這次是在每個 case 之後刪除 break
語句。
#include <iostream>
using namespace std;
int main() {
int rating = 2;
switch (rating) {
case 1:
cout << "Rated as 1. ";
case 2:
cout << "Rated as 2. ";
case 3:
cout << "Rated as 3. ";
case 4:
cout << "Rated as 4. ";
case 5:
cout << "Rated as 5. ";
}
return 0;
}
輸出:
Rated as 2. Rated as 3. Rated as 4. Rated as 5.
你可以看到,如果沒有 break
語句,即使在滿足要求後,程式也會列印每個 case
的值。
break
語句用於迴圈應該迭代的次數未知或迴圈滿足某個預定義條件的情況。
當語句體中沒有 default
語句且沒有 case
匹配時,不會執行 switch
語句體。switch
語句主體內的任何位置都只能有一個 default
語句。
最後,break
語句也用於 do、for 和 while 迴圈。在這些情況下,break
語句會在滿足特定條件時強制程式退出迴圈。
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.