在 C++ 中使用 goto 语句
Jinku Hu
2023年10月12日
C++
C++ Statement
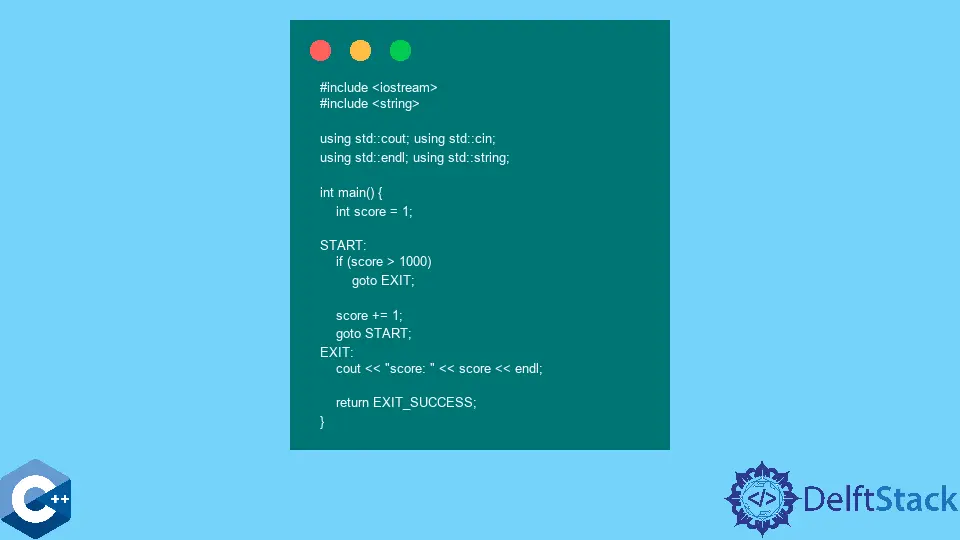
本文将介绍如何在 C++ 中使用 goto
语句。
使用 goto
语句在 C++ 中实现程序控制流跳转
goto
语句强制程序控制流跳转到标签指向的行。通常,C++ 中的任何语句都可以使用特殊符号进行标记,该符号由一个标识符和一个冒号组成。通常,这些标签标识符在函数范围内随处可用。因此,它们可以通过位于标签之前的 goto
语句引用。前面的作用域规则与变量的作用域规则不同——它强制变量在被其他语句使用之前被声明。
但请注意,goto
不能向前跳转到作用域并跳过具有初始化程序、非平凡构造函数/析构函数或非标量类型的变量声明语句。否则,预计会引发编译器错误。在下面的例子中,我们搜索给定字符串在文本中的第一个出现,一旦找到,循环就会用 goto
语句中断,在程序的末尾移动控制。因此,指示失败消息的 cout
语句将被跳过,并且仅在没有字符串与用户传递的字符串值匹配时才执行。
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
string text(
"Lorem ipsum dolor sit amet, consectetur adipiscing elit."
" Nam mollis felis eget diam fermentum, sed consequat justo pulvinar. ");
string search_query;
cout << "Enter a string to search: ";
cin >> search_query;
std::istringstream iss(text);
string word;
while (iss >> word) {
if (word == search_query) {
cout << "'" << search_query << "' - found in text" << endl;
goto END;
}
}
cout << "Could not find such string!" << endl;
END:
return EXIT_SUCCESS;
}
或者,我们可以使用用户输入验证函数来实现前面的示例,该函数在故障安全行为方面为代码增加了更强的鲁棒性。cin
成员函数用于实现输入检查功能并返回对读取数据的引用。
#include <iostream>
#include <limits>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
template <typename T>
T &validateInput(T &val) {
while (true) {
cout << "Enter a string to search: ";
if (cin >> val) {
break;
} else {
if (cin.eof()) exit(EXIT_SUCCESS);
cout << "Enter a string to search:\n";
cin.clear();
cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n');
}
}
return val;
}
int main() {
string text(
"Lorem ipsum dolor sit amet, consectetur adipiscing elit."
" Nam mollis felis eget diam fermentum, sed consequat justo pulvinar. ");
string search_query;
validateInput(search_query);
std::istringstream iss(text);
string word;
while (iss >> word) {
if (word == search_query) {
cout << "'" << search_query << "' - found in text" << endl;
goto END;
}
}
cout << "Could not find such string!" << endl;
END:
return EXIT_SUCCESS;
}
在 C++ 中使用 goto
语句来实现循环式迭代
goto
语句可用于实现类似循环的行为,其中 if
条件评估每次迭代并确定控制是否会退出循环。请注意,我们用名称 START
和 END
标记了循环体。基本上,迭代会增加 score
变量,直到它大于 1000
。因此,当值计算为 1001
时,迭代会中断,此时,程序将移动到调用 cout
语句的行。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
int score = 1;
START:
if (score > 1000) goto EXIT;
score += 1;
goto START;
EXIT:
cout << "score: " << score << endl;
return EXIT_SUCCESS;
}
输出:
score: 1001
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu