TypeScript で配列を初期化する
Shuvayan Ghosh Dastidar
2023年6月21日
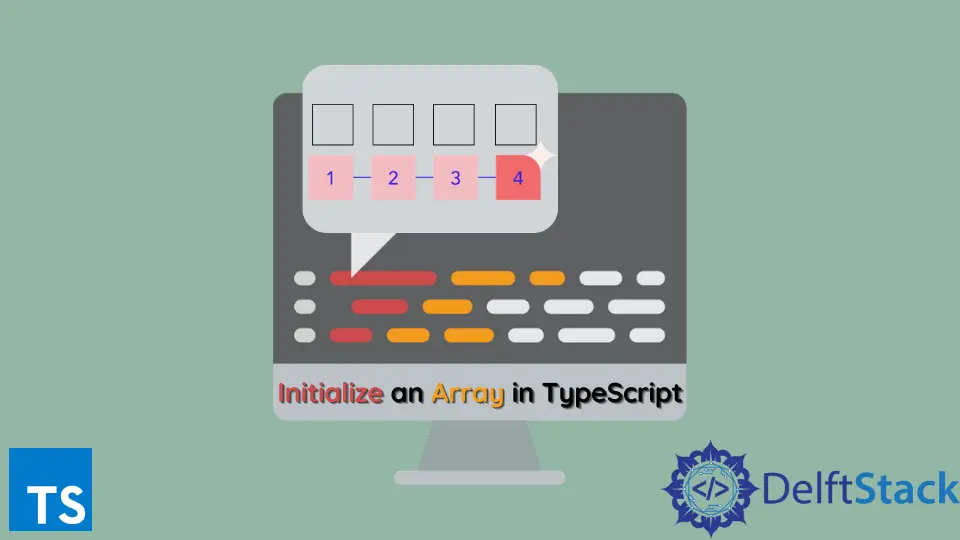
TypeScript または JavaScript の配列は、連続したメモリ セグメントを格納するためのデータ構造です。 配列は非常に便利で、ほぼすべてのアプリケーションでよく使用されます。
このチュートリアルでは、TypeScript で配列を初期化する方法を示します。
TypeScript で特定の型の配列を初期化する
TypeScript の配列は、カスタム型に割り当てられた空の配列値と Array
型を使用して初期化できます。
class Product {
name : string;
quantity : number;
constructor(opt : { name? : string, quantity? : number}){
this.name = opt.name ?? 'default product';
this.quantity = opt.quantity ?? 0;
}
}
var products : Array<Product> = [];
products.push( new Product({name : 'pencil', quantity : 10}) )
products.push( new Product({name : 'pen', quantity : 4}) )
console.log(products);
出力:
[Product: {
"name": "pencil",
"quantity": 10
}, Product: {
"name": "pen",
"quantity": 4
}]
var products : Array<Product> = [];
の代わりに、var products : Product[] = [];
を使用して、同じ機能と TypeScript による型指定サポートを取得することもできます。
配列は interface
型にすることもできます。
interface Product {
name: string;
quantity : number;
}
var products : Array<Product> = [];
var prod : Product[] = []
products.push({name : 'pencil', quantity : 10});
products.push({name : 'pen', quantity : 4});
console.log(products);
出力:
[{
"name": "pencil",
"quantity": 10
}, {
"name": "pen",
"quantity": 4
}]
TypeScript で名前付きパラメーターを使用して配列を初期化する
Array()
クラスを使用して、クラス内で配列を初期化することもできます。 次に、クラス変数を直接使用して、配列内の要素を設定したり、要素をプッシュしたりできます。
class Car {
model : string;
buildYear : number;
constructor( opt : { model? : string, buildYear? : number }){
this.model = opt.model ?? 'default';
this.buildYear = opt.buildYear ?? 0;
}
}
class Factory {
cars : Car[] = new Array();
}
var factory = new Factory();
factory.cars = [ new Car({model : 'mercedes', buildYear : 2004}), new Car( { model : 'BMW', buildYear : 2006}) ];
console.log(factory.cars);
出力:
[Car: {
"model": "mercedes",
"buildYear": 2004
}, Car: {
"model": "BMW",
"buildYear": 2006
}]