Inicializar una matriz en TypeScript
- Inicializar una matriz de ciertos tipos en TypeScript
- Usar parámetros con nombre para inicializar una matriz en TypeScript
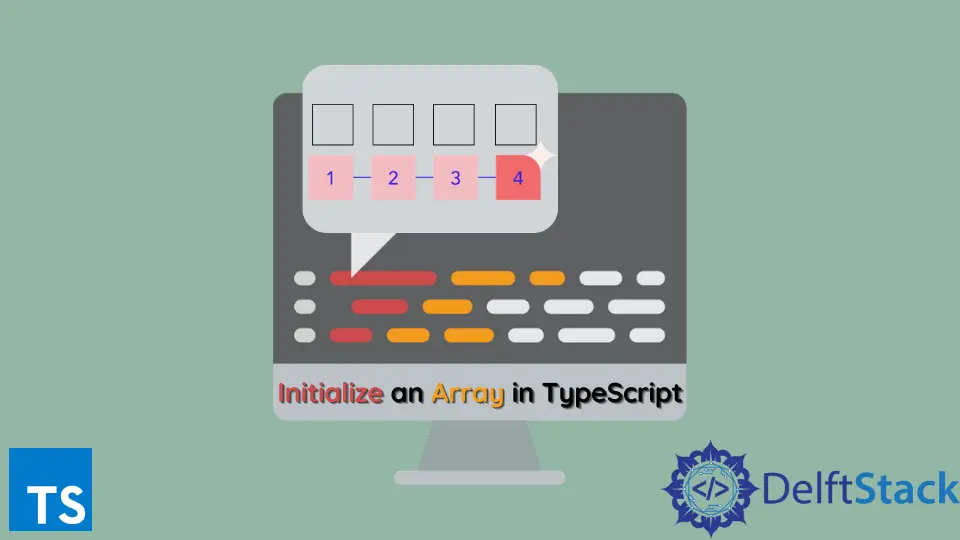
Las matrices en TypeScript o JavaScript son estructuras de datos para almacenar segmentos de memoria continuos. Los arreglos son extremadamente útiles y se usan a menudo en casi todas las aplicaciones.
Este tutorial demostrará cómo se puede inicializar una matriz en TypeScript.
Inicializar una matriz de ciertos tipos en TypeScript
Una matriz en TypeScript se puede inicializar utilizando el valor de matriz vacío y el tipo Array
, asignado a un tipo personalizado.
class Product {
name : string;
quantity : number;
constructor(opt : { name? : string, quantity? : number}){
this.name = opt.name ?? 'default product';
this.quantity = opt.quantity ?? 0;
}
}
var products : Array<Product> = [];
products.push( new Product({name : 'pencil', quantity : 10}) )
products.push( new Product({name : 'pen', quantity : 4}) )
console.log(products);
Producción :
[Product: {
"name": "pencil",
"quantity": 10
}, Product: {
"name": "pen",
"quantity": 4
}]
En lugar de var productos: Array<Producto> = [];
, también se puede usar var productos: Producto[] = [];
, y obtener la misma funcionalidad y el soporte de tipeo de TypeScript.
Las matrices también pueden ser del tipo interfaz
.
interface Product {
name: string;
quantity : number;
}
var products : Array<Product> = [];
var prod : Product[] = []
products.push({name : 'pencil', quantity : 10});
products.push({name : 'pen', quantity : 4});
console.log(products);
Producción :
[{
"name": "pencil",
"quantity": 10
}, {
"name": "pen",
"quantity": 4
}]
Usar parámetros con nombre para inicializar una matriz en TypeScript
Una matriz también se puede inicializar dentro de una clase utilizando la clase Array()
. Luego, la variable de clase se puede usar directamente para establecer elementos en la matriz o empujar elementos.
class Car {
model : string;
buildYear : number;
constructor( opt : { model? : string, buildYear? : number }){
this.model = opt.model ?? 'default';
this.buildYear = opt.buildYear ?? 0;
}
}
class Factory {
cars : Car[] = new Array();
}
var factory = new Factory();
factory.cars = [ new Car({model : 'mercedes', buildYear : 2004}), new Car( { model : 'BMW', buildYear : 2006}) ];
console.log(factory.cars);
Producción :
[Car: {
"model": "mercedes",
"buildYear": 2004
}, Car: {
"model": "BMW",
"buildYear": 2006
}]