R で train() 関数を実装する
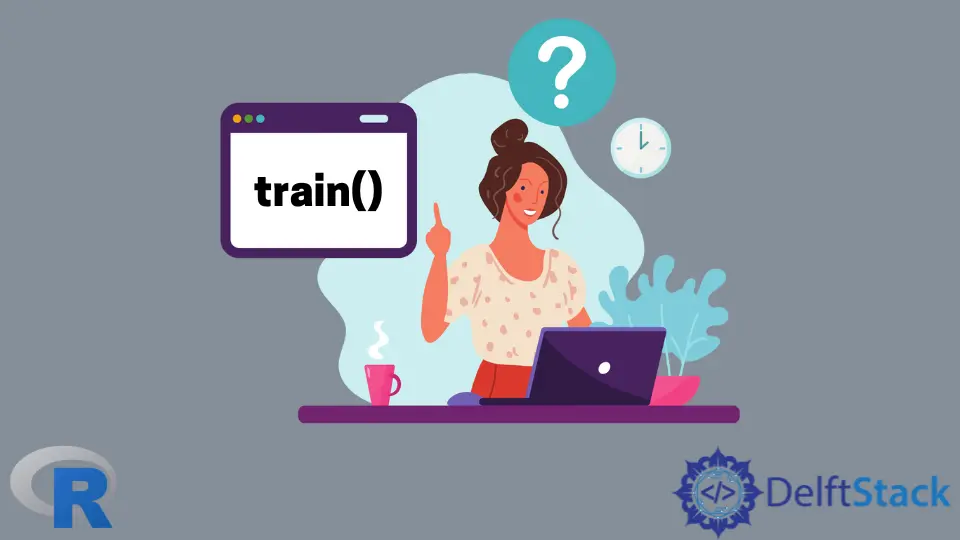
train()
メソッド (キャレット ライブラリから) は、分類と回帰トレーニングに使用されます。 また、複雑さのパラメーターを選択してモデルを調整するためにも使用されます。
このチュートリアルでは、R で caret パッケージの train()
メソッドを使用する方法を示します。
R で train()
関数を実装する
train()
メソッドを使用するには、まずキャレットとその他の必要なパッケージをインストールする必要があります。 この場合、caret および mlbench パッケージを使用します。
install.packages("caret", dependencies = c("Depends", "Suggests"))
install.packages('mlbench')
パッケージがインストールされたら、それらをロードして使用できます。 train()
メソッドに組み込みのデータセット iris
を使用します。
# Load Packages
library(caret)
library(mlbench)
# Load IRIS dataset
data(iris)
# Show the first Six lines of the iris data set
head(iris)
上記のコードは、パッケージとデータ セットを読み込み、アイリス データ セットの最初の 6 行を表示します。
Sepal.Length Sepal.Width Petal.Length Petal.Width Species
1 5.1 3.5 1.4 0.2 setosa
2 4.9 3.0 1.4 0.2 setosa
3 4.7 3.2 1.3 0.2 setosa
4 4.6 3.1 1.5 0.2 setosa
5 5.0 3.6 1.4 0.2 setosa
6 5.4 3.9 1.7 0.4 setosa
次に行うことは、データセットからオブジェクトを作成することです。これは、後で train()
メソッドで使用されます。
# create binary object
iris$binary <- ifelse(iris$Species=="setosa",1,0)
iris$Species <- NULL
その後、trainControl()
メソッドを使用してリサンプリング メソッドを変更します。 このメソッドは複数のパラメーターを取ります。 出力は train()
メソッドで使用されます。
ctrl <- trainControl(method = "repeatedcv",
number = 4,
savePredictions = TRUE,
verboseIter = T,
returnResamp = "all")
最後に、train()
メソッドを使用してデータ モデルを調整できます。 上記で作成したバイナリ オブジェクトを取得します。
パラメータとして、data
、method
、family
、および trControl
があります。
# the train method
iris_train <- train(binary ~.,
data=iris,
method = "glm",
family="binomial",
trControl = ctrl)
出力:
+ Fold1.Rep1: parameter=none
- Fold1.Rep1: parameter=none
+ Fold2.Rep1: parameter=none
- Fold2.Rep1: parameter=none
+ Fold3.Rep1: parameter=none
- Fold3.Rep1: parameter=none
+ Fold4.Rep1: parameter=none
- Fold4.Rep1: parameter=none
Aggregating results
Fitting final model on full training set
モデルは現在トレーニングされています。 フォールド レベルのパフォーマンスを確認できます。
# fold level performance
iris_train$resample
リサンプリング出力:
RMSE Rsquared MAE parameter Resample
1 3.929660e-06 1.0000000 6.420415e-07 none Fold1.Rep1
2 2.735382e-13 1.0000000 6.115382e-14 none Fold2.Rep1
3 8.221919e-12 1.0000000 1.397945e-12 none Fold3.Rep1
4 9.119130e-04 0.9999968 1.479318e-04 none Fold4.Rep1
モデルがトレーニングされると、データセット内の他のオプションを使用して中間モデルを作成できます。
完全なサンプルコード
便宜上、完全なサンプル コードを次に示します。
install.packages("caret", dependencies = c("Depends", "Suggests"))
install.packages('mlbench')
# Load Packages
library(caret)
library(mlbench)
# Load IRIS dataset
data(iris)
# Show the first Six lines of the iris data set
head(iris)
# create binary object
iris$binary <- ifelse(iris$Species=="setosa",1,0)
iris$Species <- NULL
# use trainControl() method to modify the resampling method
ctrl <- trainControl(method = "repeatedcv",
number = 4,
savePredictions = TRUE,
verboseIter = T,
returnResamp = "all")
# the train method
iris_train <- train(binary ~.,
data=iris,
method = "glm",
family="binomial",
trControl = ctrl)
# fold level performance
iris_train$resample
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook