R で行の合計が一定のランダム行列を作成する
Jesse John
2023年6月21日
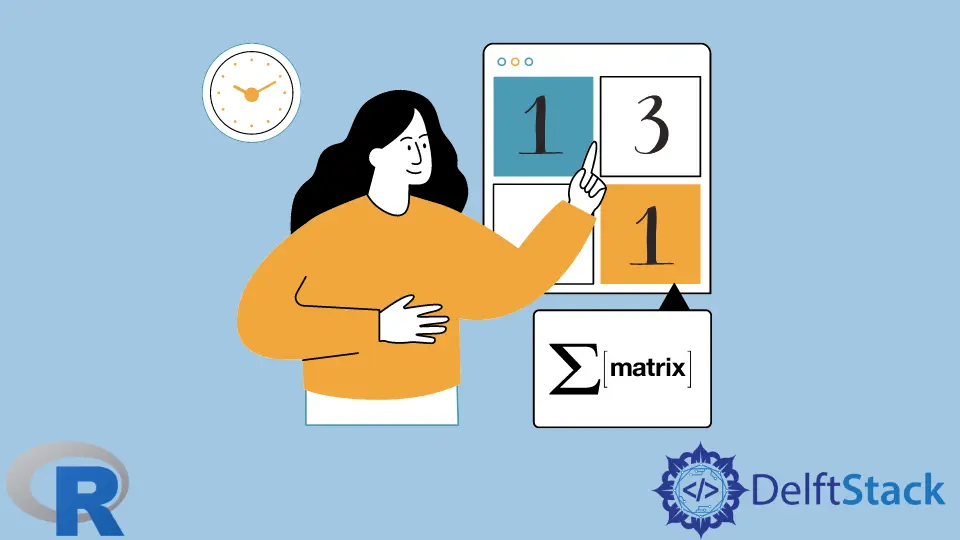
この記事では、合計がすべての行で一定になるように、ランダムに選択された負でない値を各行に含む行列を作成する方法を説明します。
簡単にするために、すべての行の合計が 1 になるケースを取り上げます。行ベクトルを任意の数で乗算して、その数を行の合計として取得できます。
R で行の合計が一定のランダム行列を作成する
各行に 4つの要素が必要だとします。 これは、1 より大きい任意の正の整数にすることができます。
パーセンタイルを表す 0 から 100 の範囲から始めます。 ランダムに選択した 3つのカット ポイントを使用して、この範囲を 4つの部分に分割します。
これを行うために、上記の範囲でランダムに 3つのポイントを選択しました。 簡単にするために、3つの別々の数値を選択します。
選択したカット ポイントを降順に並べ替えます。 これらは、範囲をカットするパーセンタイルです。
カット ポイントを 100 で割ります。これにより、カット ポイントが 0 から 1 の小数として得られます。
次のように、1 の 4つのセクションを取得します。
- 1 から最初の (最大の) カット ポイントを引いて、最初の要素を取得します。
- 最初のカット ポイントから 2 番目のカット ポイントを引いて、2 番目の要素を取得します。
- 2 番目のカット ポイントから 3 番目 (最小) のカット ポイントを引くことによって、3 番目の要素を取得します。
- 3 番目のカット ポイントから 0 を引いて、4 番目の要素を取得します。
これらの数値は 1つの行を構成します。 合計すると 1 になります。このプロセスを必要な行数だけ繰り返す必要があります。
マトリックスのコード
ここで、各行の合計が 1 である 5 行 4 列のランダム行列を取得するコードを見ていきます。
コード例:
# The final matrix.
# It begins as an empty matrix. It has 4 columns in our example.
Mx = matrix(byrow= TRUE, ncol = 4, nrow = 0)
# The number of rows. 5 rows in our example.
# We will repeat the code as many times as the number of rows we need.
for(RowNum in 1:5) {
# Cut points. With 3 cuts, we will get 4 elements.
# Randomly choose 3 different integers from the sequence 0 to 100.
cuts = sample(0:100, 3, replace = FALSE)
# Sort the cut points in descending order.
cuts.sort = sort(cuts, decreasing = TRUE)
# Convert the sorted cut points to decimals from 0 to 1.
cuts.dec = cuts.sort/100
# An empty vector to hold the current row that we will build.
Elements = c()
# The starting point for each cut.
# The first cut starts at 1. Then resets this to its value.
ElementStart = 1
# Create each element.
for(Cut in cuts.dec){
Elements = c(Elements, (ElementStart-Cut))
ElementStart = Cut
}
# Append the last element to get the full row.
# This is from the last (smallest) cut value to 0.
# (This vector can be multiplied by any number to get that number as the row sum.)
Elements = c(Elements, cuts.dec[3])
# Add the row to the matrix.
Mx = rbind(Mx, Elements)
rownames(Mx) = NULL
}
# Print the matrix.
print(Mx)
# Check that all 5 rows sum to 1.
table(apply(Mx, 1, sum))
出力:
> # Print the matrix.
> print(Mx)
[,1] [,2] [,3] [,4]
[1,] 0.38 0.36 0.16 0.10
[2,] 0.41 0.15 0.03 0.41
[3,] 0.08 0.50 0.35 0.07
[4,] 0.17 0.15 0.06 0.62
[5,] 0.07 0.09 0.23 0.61
>
> # Check that all 5 rows sum to 1.
> table(apply(Mx, 1, sum))
1
5
著者: Jesse John